Python function attribute decorator
Python function attribute decorator
The joys of Python programming!
Python function attributes are a fascinating topic that allows us to enrich our functions with additional metadata. This can be incredibly useful when writing robust and maintainable code.
In Python, we can use the @
symbol followed by the name of a function or class to decorate it. Decorators are essentially higher-order functions that take another function as an argument. They allow us to execute arbitrary code before, after, or around the execution of our original function.
When it comes to Python function attributes, decorators play a crucial role in assigning and accessing these attributes.
Here's a simple example:
def add_logging(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.name} with args: {args}, kwargs: {kwargs}")
return func(*args, **kwargs)
return wrapper
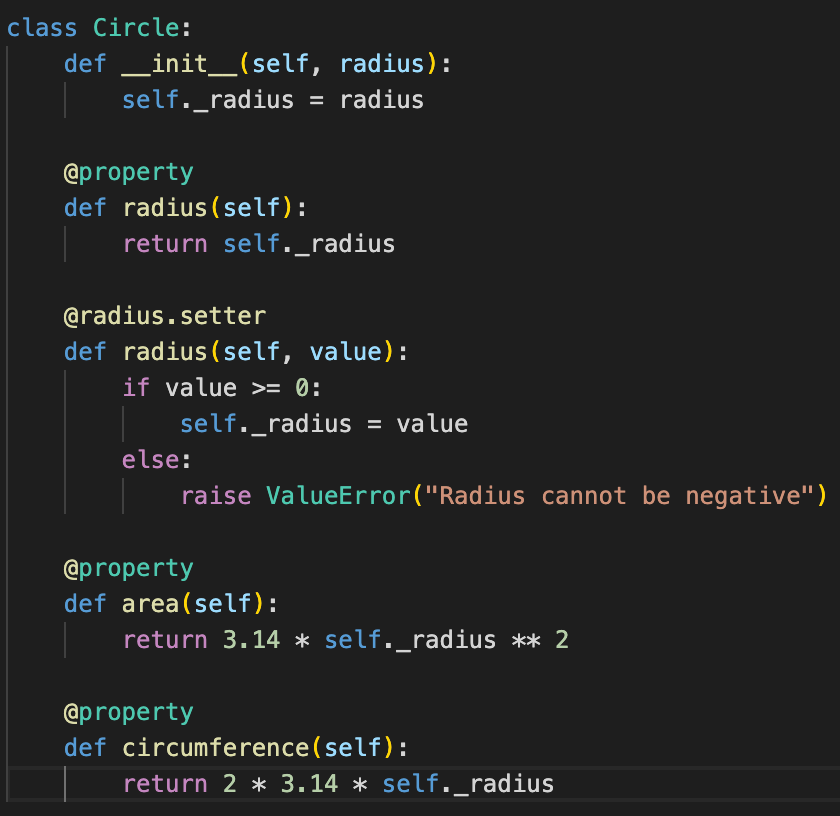
@add_logging
def my_function(x, y):
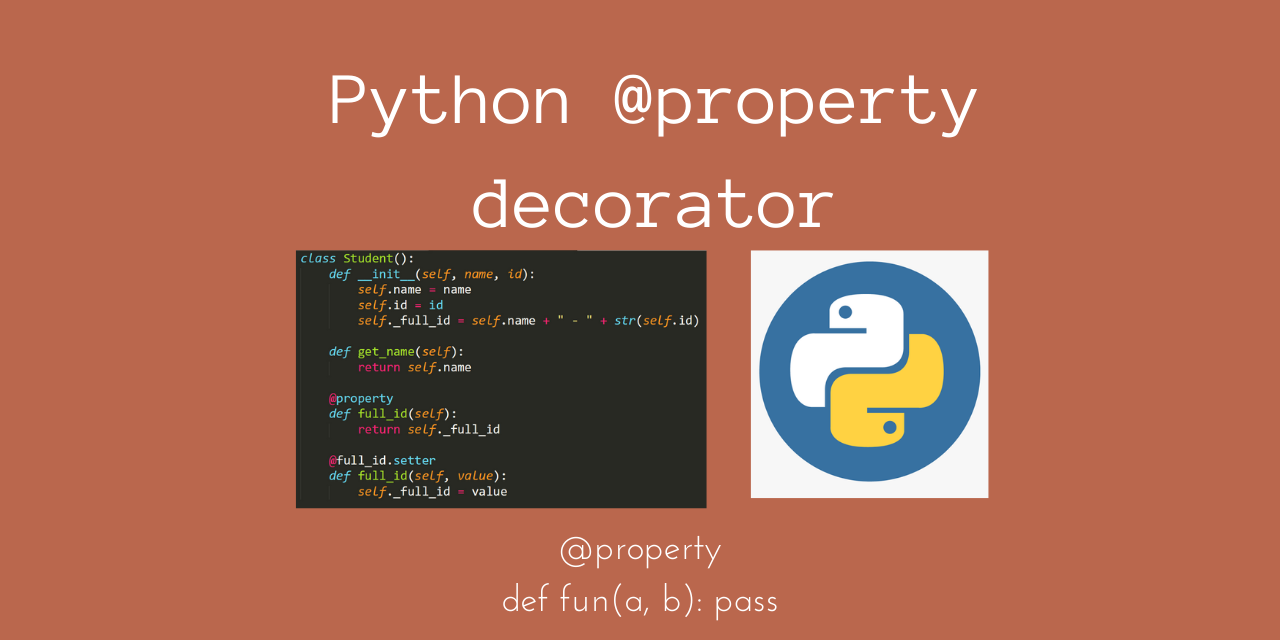
return x + y
result = my_function(2, 3)
print(result) # Output: 5
In this example, the add_logging
decorator is applied to our my_function
. When we call my_function
, the decorator's wrapped function (wrapper
) gets executed first. It logs a message indicating that the original function is being called with some arguments and keyword arguments. Then it calls the original function (my_function
) with the same arguments, effectively wrapping the original function.
Function attributes are essentially arbitrary key-value pairs stored alongside our functions. We can use these to attach additional metadata or behavior to our functions. Decorators provide a way to modify or inspect these attributes programmatically.
Here's an example:
def has_cache(func):
func.cache = {}
return func
@has_cache
def cached_function(x, y):
if (x, y) in cached_function.cache:
return cached_function.cache[(x, y)]
result = x + y # compute the result
cached_function.cache[(x, y)] = result
return result
result1 = cached_function(2, 3)
print(result1) # Output: 5 (cached)
result2 = cached_function(4, 5)
print(result2) # Output: 9 (computed and cached)
In this example, we define a has_cache
decorator that initializes a cache
attribute on our decorated function. When we apply the decorator to our cached_function
, it gets this cache attribute. The function uses this cache to store previous results and avoid redundant computations.
Function attributes can be accessed using the .func_name
or .func
syntax:
def my_decorator(func):
func.my_attribute = "Hello, World!"
return func
@my_decorator
def my_function(x):
pass
print(my_function.name) # Output: my_function
print(my_function.my_attribute) # Output: Hello, World!
In this example, we define a my_decorator
that assigns a my_attribute
to our decorated function. When we access the attribute using .func_name
, it returns the original function name; however, when we access the attribute using .func
, it returns the modified attribute value.
In conclusion, Python function attributes are a powerful feature that allows us to associate additional metadata or behavior with our functions. Decorators provide a way to modify or inspect these attributes programmatically, making our code more robust and maintainable.
So, the next time you're writing Python code, don't forget to leverage these awesomesauce features!
Do Python variables have attributes?
I'd be happy to help you with that!
In Python, variables do not have attributes in the classical sense like they do in other programming languages like Java or C++. Variables are simply names given to a value in memory. When you assign a value to a variable, you're essentially creating a name for that value.
However, if you're thinking of something similar to attributes, Python has its own way of handling it, which is through the __dict__
attribute.
In Python, every object (including functions and classes) has a built-in dictionary called __dict__
that stores all its attributes. You can think of this as a dictionary where the keys are the names of the attributes and the values are the actual values of those attributes.
For example:
class Person:
def init(self, name):
self.name = name
person = Person("John")
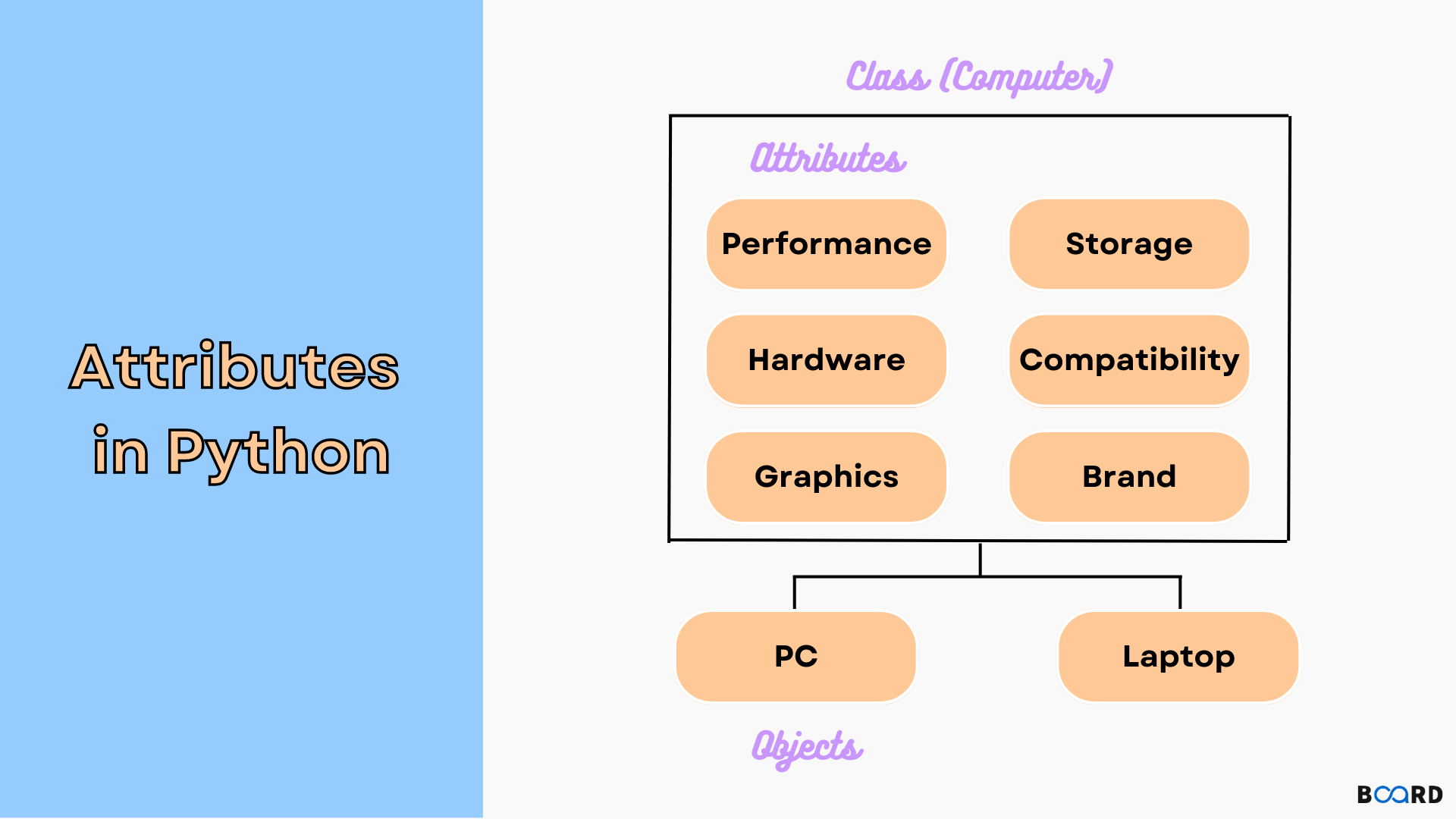
print(person.dict) # {'name': 'John'}
In this case, person
has an attribute called name
, which is stored in its __dict__
. You can also add more attributes to the object using dot notation:
class Person:
def init(self, name):
self.name = name
person = Person("John")
person.age = 30 # adds a new attribute
print(person.dict) # {'name': 'John', 'age': 30}
Now, person
has two attributes: name
and age
. This is similar to having attributes in other languages.
It's worth noting that this __dict__
attribute is not specific to variables; every Python object has one. So if you're working with a class or function, you can access its attributes using the __dict__
attribute.
So, while it might seem like Python variables don't have attributes at first glance, they actually do in a sense! It's all about understanding how Python objects and dictionaries work together.