Python projects with source code for beginners
Python projects with source code for beginners
I'm happy to help you with that!
As a beginner, it's essential to start with simple and practical projects that can help you build a strong foundation in programming. Here are some fantastic Python projects with source code that are perfect for beginners:
To-Do List App: Create a simple to-do list app that allows users to add, remove, and edit tasks. This project is an excellent way to learn about user input, lists, and conditional statements.Source Code:
# todo_list.py
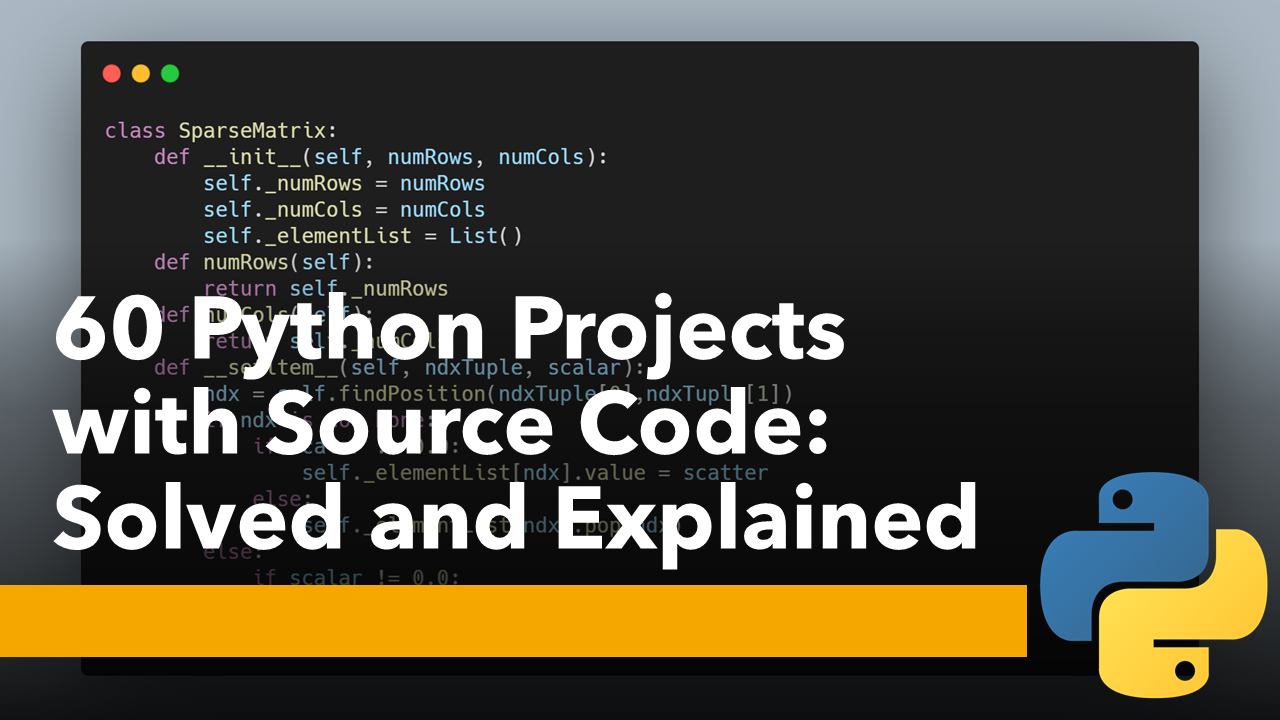
def get_user_input():
task = input("Enter a new task: ")
return task
def display_tasks(tasks):
print("Current Tasks:")
for i, task in enumerate(tasks, start=1):
print(f"{i}. {task}")
def main():
tasks = []
while True:
user_choice = input("What would you like to do? (A)dd, (R)emove, or (E)dit? ")
if user_choice.upper() == "A":
task = get_user_input()
tasks.append(task)
elif user_choice.upper() == "R":
display_tasks(tasks)
remove_task_index = int(input("Enter the task number to remove: ")) - 1
try:
del tasks[remove_task_index]
except IndexError:
print("Task not found!")
elif user_choice.upper() == "E":
display_tasks(tasks)
edit_task_index = int(input("Enter the task number to edit: ")) - 1
try:
new_task = input("Enter the new task: ")
tasks[edit_task_index] = new_task
except IndexError:
print("Task not found!")
else:
print("Invalid choice. Please try again.")
if name == "main":
main()
Guessing Game: Create a guessing game where the user has to guess a number between 1 and 100. This project is an excellent way to learn about random numbers, conditional statements, and loops.
Source Code:
# guessing_game.py
import random
def get_user_guess():
while True:
try:
user_guess = int(input("Enter your guess (between 1 and 100): "))
if 1 <= user_guess <= 100:
return user_guess
else:
print("Invalid input! Try again.")
except ValueError:
print("Invalid input! Try again.")
def game_loop():
secret_number = random.randint(1, 100)
attempts = 0
while True:
user_guess = get_user_guess()
attempts += 1
if user_guess < secret_number:
print("Too low!")
elif user_guess > secret_number:
print("Too high!")
else:
print(f" Congratulations! You found the number in {attempts} attempts.")
break
if name == "main":
game_loop()
Hangman Game: Create a simple hangman game where the user has to guess a word by suggesting letters. This project is an excellent way to learn about lists, conditional statements, and loops.
Source Code:
# hangman.py
import random
def load_words(filename):
with open(filename, "r") as file:
words = [line.strip() for line in file]
return random.choice(words)
def display_word(word, guessed_letters):
print("Current Word:", end=" ")
for letter in word:
if letter in guessed_letters:
print(letter, end=" ")
else:
print("_", end=" ")
print()
def get_user_input(guessed_letters):
while True:
try:
user_guess = input("Enter a letter: ").strip().upper()
return [user_guess] + guessed_letters
except ValueError:
print("Invalid input! Try again.")
def game_loop():
word = load_words("words.txt")
guessed_letters = []
while True:
display_word(word, guessed_letters)
user_input = get_user_input(guessed_letters)
if user_input[0] in word:
guessed_letters = user_input
else:
print("Oops! That letter is not in the word. Try again!")
if "_" not in display_word(word, guessed_letters):
print(f" Congratulations! You found the word: {word}.")
break
if name == "main":
game_loop()
These projects are just a starting point for beginners. As you progress, you'll find that each project builds upon previous skills and knowledge. Remember to practice regularly and have fun with your coding journey!
Can you make projects with Python?
I'd be happy to help!
Python is an excellent language for creating a wide range of projects, and its versatility has made it a popular choice among developers and non-developers alike. Here are some examples of projects you can create using Python:
Web Development: Python can be used as a backend technology to build web applications using frameworks such as Django or Flask. You can create custom web apps with a user-friendly interface, handling database interactions, and more. Data Analysis and Visualization: Python is particularly well-suited for data analysis and visualization tasks, thanks to libraries like Pandas, NumPy, and Matplotlib. You can collect and process large datasets, create interactive dashboards, and even build predictive models. Machine Learning and AI: Python has an extensive range of libraries and tools dedicated to machine learning and artificial intelligence, including TensorFlow, Keras, OpenCV, and scikit-learn. With these, you can train your own neural networks, classify images, or create chatbots that understand natural language processing.These are just a few examples of the many exciting projects you can undertake using Python. The language's versatility, ease of use, and extensive library support make it an excellent starting point for beginners and experienced developers alike!