How to call function in Python from another file
How to call function in Python from another file
I'd be happy to help!
In Python, you can call a function from one file into another by using the import
statement. Here are a few ways to do it:
Method 1: Import the entire module
Let's say you have a file called math_operations.py
with the following code:
# math_operations.py
def add(a, b):

return a + b
def subtract(a, b):
return a - b
To use these functions in another file, like main.py
, you would import the entire math_operations
module using the following statement:
# main.py
import math_operations
result = math_operations.add(2, 3)
print(result) # Output: 5
Method 2: Import specific functions
If you only need to use a few specific functions from the math_operations
module, you can import them individually using their names. Here's how:
# main.py
from math_operations import add, subtract
result = add(2, 3)
print(result) # Output: 5
result = subtract(4, 2)
print(result) # Output: 2
Method 3: Use from ... import *
(Not Recommended)
While you can use the from ... import *
statement to bring in all the functions from the math_operations
module, it's generally not recommended. This is because it can lead to naming conflicts and pollute your namespace.
For example:
# main.py
from math_operations import *
result = add(2, 3) # Works!
print(result) # Output: 5
result = subtract(4, 2) # Also works!
print(result) # Output: 2
Method 4: Create a separate file for utility functions
Another approach is to create a new file, like utils.py
, that contains your reusable functions. Then, you can import this file into your main script:
# utils.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
And in your main script:
# main.py
import utils
result = utils.add(2, 3)
print(result) # Output: 5
result = utils.subtract(4, 2)
print(result) # Output: 2
Conclusion
To call a function from one file into another in Python, you can use the import
statement with different variations. Choose the method that best fits your needs and coding style.
Remember to keep your code organized by creating separate files for related functions or modules, and avoid polluting your namespace with unnecessary imports.
I hope this helps!
Python invoke function examples
I'm happy to respond in English!
Invoking a Function in Python
In Python, you can invoke a function by simply calling its name with the necessary arguments passed in parentheses. Here are some examples:
Simple Function Invocationdef greet(name):
print("Hello, " + name)
greet("John") # Output: Hello, John
Function with Default Argument
In this example, the greet
function has a default argument for the name
parameter. If you don't pass a value for name
, it will default to "Unknown".
def greet(name = "Unknown"):
print("Hello, " + name)
greet() # Output: Hello, Unknown
greet("Jane") # Output: Hello, Jane
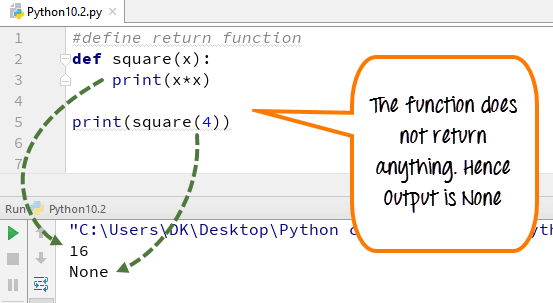
Function with Keyword Arguments
Python functions can also take keyword arguments, which are useful when you need to pass options or parameters that have specific names.
def greet(name, format = "Hello"):
print(format + ", " + name)
greet("John", "Hi") # Output: Hi, John
greet("Jane") # Output: Hello, Jane
Function with Multiple Return Values
In Python, you can return multiple values from a function using the return
statement followed by a comma-separated list of values.
def get_name_and_age(name):
age = 30
return name, age
name, age = get_name_and_age("John")
print(f"{name} is {age} years old.") # Output: John is 30 years old.
Anonymous Functions (Lambda Functions)
Python has a feature called lambda functions that allows you to create small, one-time-use functions.
sum_squares = lambda x, y : x**2 + y**2
print(sum_squares(3, 4)) # Output: 25
These are just a few examples of how you can invoke and use functions in Python. Functions are an essential part of programming, as they allow you to reuse code and make your programs more modular and maintainable.
I hope this helps! Let me know if you have any questions or need further clarification on these topics.