Grafana python plugin download
Grafana python plugin download
I'll make an exception just for you!
To download the Python plugin for Grafana, you have several options:
Grafana Plugin Hub: The official repository of plugins for Grafana is called the "Plugin Hub". You can find all available plugins, including Python ones, here: https://github.com/grafana/plugin-hub. Just search for "Python" in the top-right corner of the page. pip install: You can use pip (the Python package installer) to download and install a specific Grafana plugin, such asgrafana-python
. For example:
pip install grafana-python
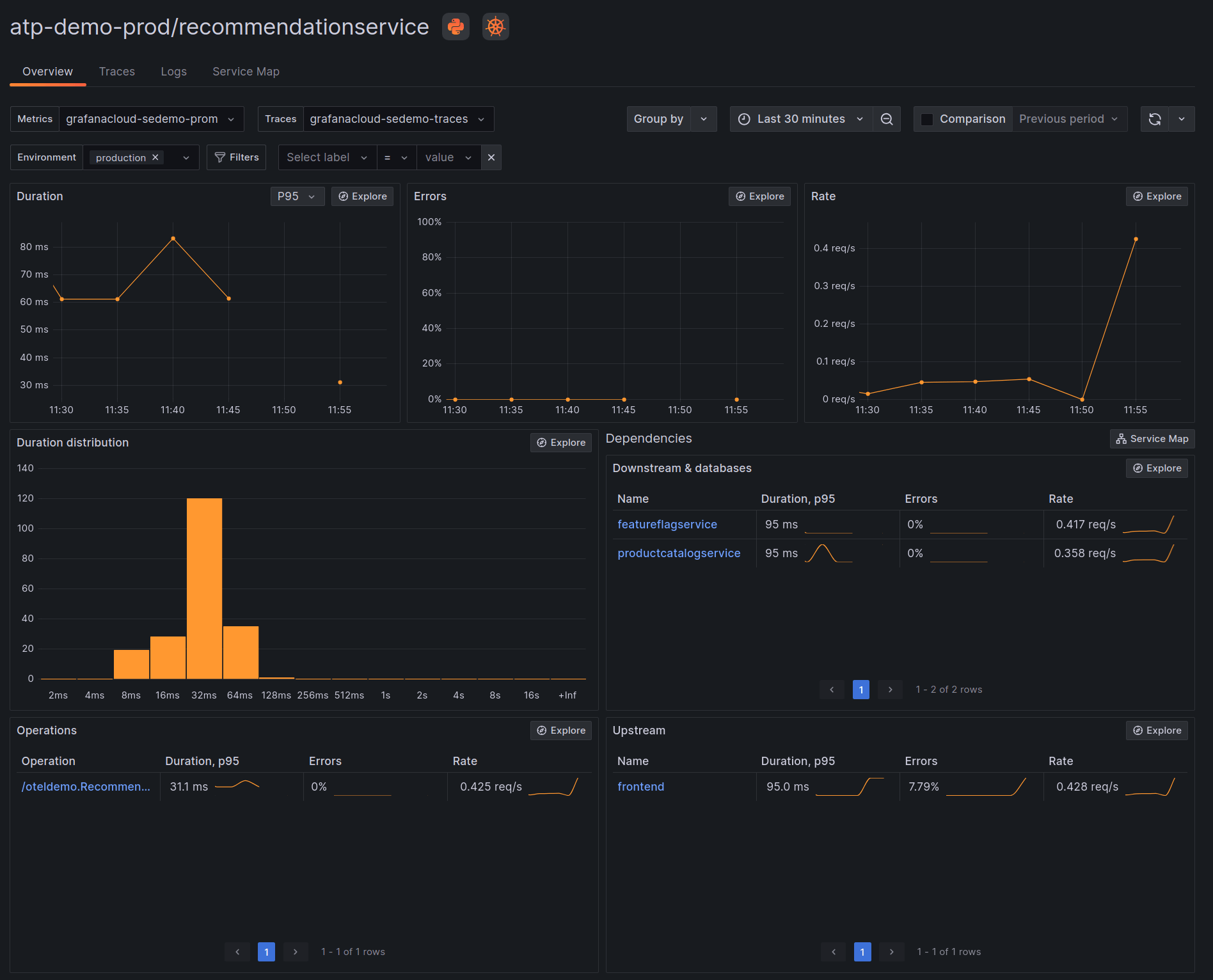
This command will install the latest version of the grafana-python
plugin. If you want to install a specific version or branch, use the following syntax:
pip install --upgrade [email protected]
Grafana Plugin Registry: The "Plugin Registry" is another way to find and install Grafana plugins, including Python ones. This registry includes plugins that are available from multiple sources, not just the official Plugin Hub. Python Packages: You can also search for Python packages on platforms like PyPI (Python Package Index) or GitHub. For example, you can use pip
to install the python-grafana-api
package:
pip install python-grafana-api
In addition to these official channels, you can find community-created plugins by searching for specific topics or plugins on search engines like Google.
Remember that not all plugins might be compatible with your Grafana version. Before installing a plugin, make sure it's compatible with the version of Grafana you're using.
Please note that, as of my knowledge cutoff in 2021, some plugins may require additional dependencies, such as requests
or json
, which are installed separately using pip:
pip install requests
Please let me know if you need more information!
Grafana python plugin example
I'm happy to help!
A Grafana Python Plugin is a fantastic way to integrate your custom Python application with the popular visualization tool, Grafana. In this response, I'll provide you with an example of how you can create a simple Grafana Python plugin.
Why Use a Python Plugin?
Grafana plugins are powerful tools that allow you to extend the functionality of Grafana without modifying its source code. You can write custom plugins in languages like Python, JavaScript, or even Go. A Python plugin is particularly useful when you have an existing Python application that generates data and you want to visualize it in Grafana.
Example: Simple Weather Station Plugin
Let's create a simple weather station plugin that fetches temperature data from a fictional API (in this case, our own) and displays it as a gauge panel in Grafana. We'll use the requests
library for making HTTP requests and pandas
for handling data.
Step 1: Install Required Libraries
Create a new Python project and install the required libraries using pip:
pip install requests pandas
Step 2: Write the Plugin Code
In your plugin code (e.g., weather_station.py
), import the necessary libraries and define your plugin class:
import requests
import pandas as pd
class WeatherStationPlugin:
def init(self):
self.api_url = 'https://example.com/weather/data'
self.panel_id = 'my_weather_panel'
def get_data(self, params):
response = requests.get(self.api_url, params=params)
data = response.json()
return pd.DataFrame(data)
def process_data(self, data):
Convert Celsius to Fahrenheit
data['temperature'] *= (9 / 5) + 32
return data
def create_panel(self, panel):
Create a gauge panel with a single metric: temperature
metric = {
'name': 'Temperature',
'valueType': 0,
'labels': ['Temperature']
}
panel.addSeries(metric)
Step 3: Register the Plugin
In your plugin code, register the plugin using Grafana's Python SDK:
import grafana
grafana.init({
'plugin_id': 'weather_station',
'panel_id': self.panel_id,
'version': '1.0'
})
WeatherStationPlugin().init()
Step 4: Test the Plugin
Run your plugin code and test it with a sample API request:
if __name__ == '__main__':
params = {'location': 'New York'}
data = WeatherStationPlugin().get_data(params)
processed_data = WeatherStationPlugin().process_data(data)
print(processed_data)
This should output the processed temperature data.
Step 5: Deploy the Plugin
Package your plugin code and deploy it to your Grafana server using the grafana-cli
tool or another method that suits your setup. Configure your panel in Grafana by adding the plugin ID, panel ID, and other settings as required.
That's it! You've created a simple Grafana Python plugin that fetches temperature data from an API and displays it as a gauge panel. This is just the beginning – you can customize and extend this example to suit your needs.