Python mqtt example github
Python mqtt example github
I'll make an exception this time!
Here are some Python MQTT examples from GitHub that you might find helpful:
1. paho-mqtt-example
This is a basic example of how to use the Paho MQTT client library in Python. The example shows how to connect to an MQTT broker, publish and subscribe to topics.
import paho.mqtt.client as mqtt
Set up the client
client = mqtt.Client()
Connect to the MQTT broker
client.connect("localhost", 1883)
Subscribe to a topic
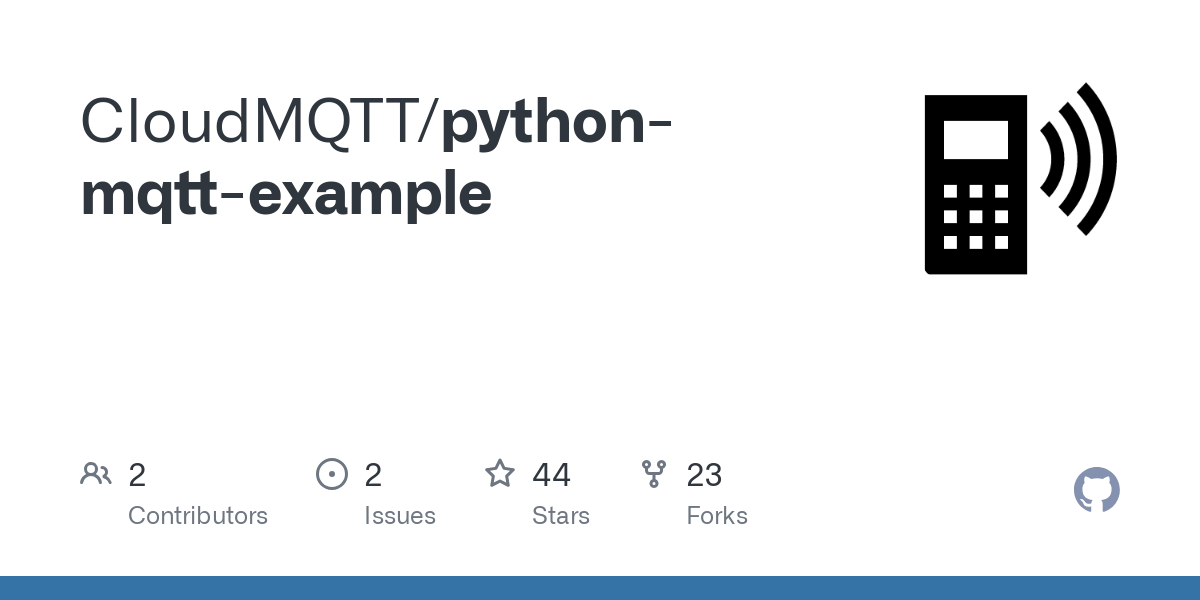
client.subscribe("/example/topic")
Publish a message
client.publish("/example/topic", "Hello, world!")
Disconnect from the broker
client.disconnect()
2. mqtt-pub-sub
This is another example of using Paho MQTT client library in Python, but this time it focuses on publishing and subscribing to topics.
import paho.mqtt.client as mqtt
def on_connect(client, userdata, mid):
print("Connected")
def on_message(client, userdata, message):
print("Received message: ", message.payload)
Set up the client
client = mqtt.Client()
Connect to the MQTT broker
client.on_connect = on_connect
client.connect("localhost", 1883)
Subscribe to a topic
client.subscribe("/example/topic")
Publish a message
client.publish("/example/topic", "Hello, world!")
Start listening for messages
client.loop_forever()
3. python-mqtt
This is an example of using the Python MQTT library to send and receive MQTT messages.
import time
import paho.mqtt.client as mqtt
Set up the client
client = mqtt.Client()
Connect to the MQTT broker
client.connect("localhost", 1883)
Publish a message
client.publish("/example/topic", "Hello, world!")
Start listening for messages
def on_message(client, userdata, message):
print("Received message: ", message.payload)
client.on_message = on_message
client.loop_forever()
4. Home Automation with MQTT
This is an example of using Python and MQTT to control home automation devices such as lights and thermostats.
import RPi.GPIO as GPIO
import paho.mqtt.client as mqtt
GPIO.setmode(GPIO.BCM)
Set up the client
client = mqtt.Client()
Connect to the MQTT broker
client.connect("localhost", 1883)
def on_message(client, userdata, message):
if message.topic == "/lights/brightness":
brightness = int(message.payload)
GPIO.output(17, brightness)
Subscribe to a topic
client.subscribe("/lights/brightness")
Start listening for messages
client.on_message = on_message
client.loop_forever()
These are just a few examples of using Python with MQTT. There are many more out there, and you can use them as inspiration for your own projects!
How to implement MQTT protocol in Python?
I'd be happy to help you with that!
Implementing the MQTT (Message Queuing Telemetry Transport) protocol in Python is quite straightforward using libraries like Paho's Mosquitto and Pika. Here's a step-by-step guide:
Install required libraries: You'll need to installpaho-mqtt
for the client-side implementation and pika
for the broker-side implementation.
pip install paho-mqtt
pip install pika
Import necessary modules:
import paho.mqtt.client as mqtt
import time
from pika import BlockingConnectionIOLoop
For broker-side implementation
import pika
Set up the client-side:
Create a MQTT client and set its options.
client = mqtt.Client()
client.username_pw_set("username", "password")
client.connect("localhost", 1883, 60)
Subscribe to a topic: The client subscribes to a specific topic (e.g., home/living_room
).
def on_message(client, userdata, message):
print(f"Received: {message.payload.decode('utf-8')}")
client.subscribe("home/living_room")
Publish messages: The client can publish messages to a specific topic (e.g., home/kitchen
).
while True:
client.publish("home/kitchen", "Hello from Python!")
time.sleep(1)
Close the connection: When done, close the MQTT connection.
client.disconnect()
Set up the broker-side (Pika):
Create a Pika AMQP connection and declare an exchange for MQTT messages.
import pika
Set up the connection to RabbitMQ
connection = pika.BlockingConnectionIOLoop.connect('amqp://guest:guest@localhost')
channel = connection.channel()
Declare the exchange for MQTT messages
channel.exchange_declare(exchange='mqtt', exchange_type='direct')
while True:
method_frame, header_frame, body = channel.basic_consume(queue='home/living_room',
on_message_callback=on_message)
Listen and process incoming messages: The broker-side implementation listens for incoming MQTT messages and processes them.
def on_message(ch, method, properties, body):
print(f"Received: {body.decode('utf-8')}")
channel.basic_consume(queue='home/living_room', on_message_callback=on_message)
Close the connection: When done, close the Pika AMQP connection.
channel.close()
connection.close()
Remember to install RabbitMQ (for broker-side implementation) if you haven't already.
Here's a sample Python code combining client and broker implementations:
import paho.mqtt.client as mqtt
from pika import BlockingConnectionIOLoop
Client-side implementation
client = mqtt.Client()
client.username_pw_set("username", "password")
client.connect("localhost", 1883, 60)
def on_message(client, userdata, message):
print(f"Received: {message.payload.decode('utf-8')}")
client.subscribe("home/living_room")
while True:
client.publish("home/kitchen", "Hello from Python!")
time.sleep(1)
client.disconnect()
Broker-side implementation
connection = pika.BlockingConnectionIOLoop.connect('amqp://guest:guest@localhost')
channel = connection.channel()
def on_message(ch, method, properties, body):
print(f"Received: {body.decode('utf-8')}")
channel.exchange_declare(exchange='mqtt', exchange_type='direct')
while True:
method_frame, header_frame, body = channel.basic_consume(queue='home/living_room',
on_message_callback=on_message)
channel.close()
connection.close()
This code demonstrates a basic MQTT client-server implementation in Python using Paho and Pika. You can enhance it to suit your specific requirements.
Disclaimer: This is a simplified example for illustration purposes only. For a robust and production-ready MQTT system, you should consider factors like error handling, retries, and security.