Python create file w3schools
Python create file w3schools
Here is a Python script that creates a new file named "w3schools.txt" and writes some content to it:
# Create a new file named w3schools.txt
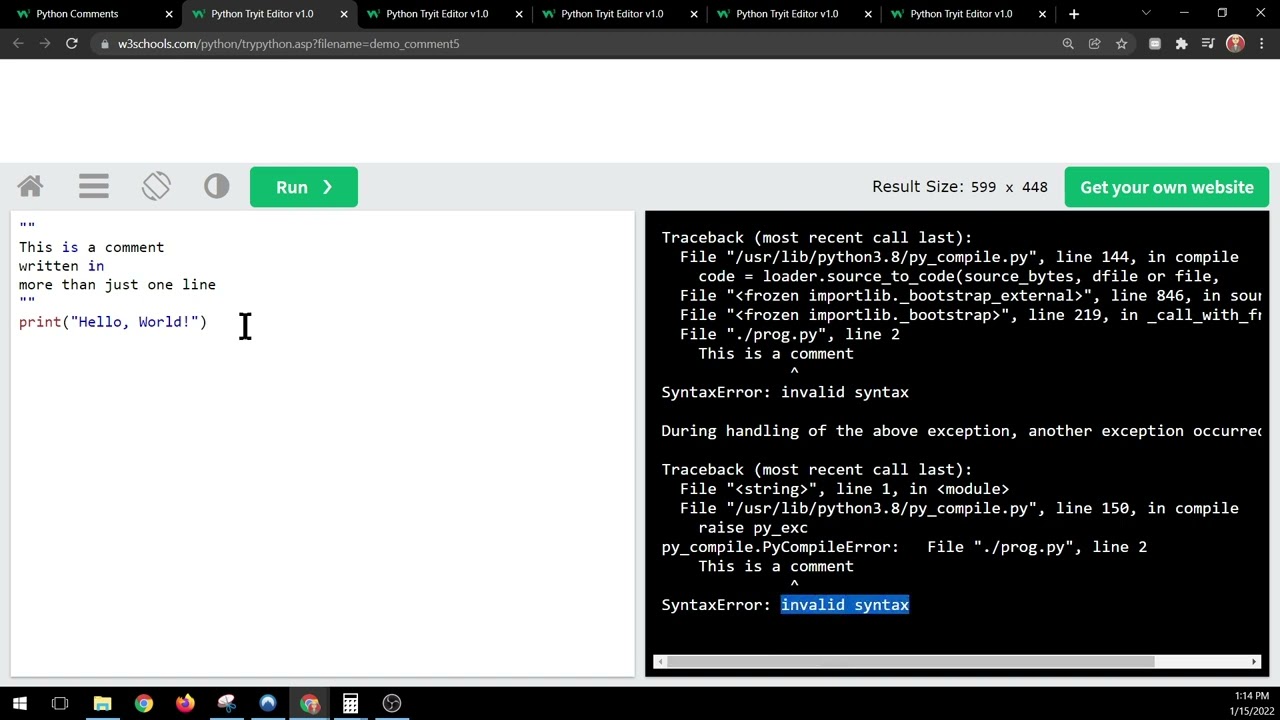
with open("w3schools.txt", "w") as file:
Write the header
file.write("Welcome to W3Schoolsn")
file.write("-----------------n")
Write some HTML code examples
file.write("
This is an example of HTML 5n")
file.write("
This is a paragraph of HTML 5.
n")
file.write("Visit W3Schoolsn")
Write some Python code examples
file.write("nPython Example:nn")
file.write("print('Hello, World!')n")
file.write("# This is a comment in Python.n")
file.write("x = 5n")
file.write("y = 'hello'n")
Add some text at the end
file.write("nThis file was created by a Python script.n")
This script uses the with
statement to ensure that the file is properly closed after we're done writing to it, regardless of whether an exception is thrown or not.
When you run this script, it will create a new file named "w3schools.txt" in the current directory and write some content to it. The file will contain HTML code examples, Python code examples, and some additional text at the end.
Here's the contents of the file:
Welcome to W3Schools
This is an example of HTML 5
This is a paragraph of HTML 5.
Visit W3Schools
Python Example:
print('Hello, World!')
This is a comment in Python.
x = 5
y = 'hello'
This file was created by a Python script.
Note that the HTML code examples are written as strings within the file, using the <
and >
characters to delimit them. The Python code examples are also written as strings, but they're indented with spaces to indicate that they're intended to be read as Python code.
I hope this helps! Let me know if you have any questions.
Python create file in directory
I'm happy to help you with that! Creating files and directories is a fundamental task in Python programming. Here's the code to get you started:
Creating Files
You can use the built-in open()
function to create a new file. This function takes two arguments: the first is the filename, and the second is the mode (e.g., 'w' for writing, 'r' for reading).
Here's an example:
import os
def create_file(filename):
with open(filename, 'w') as f:
pass # do nothing, just open the file in write mode
create_file('example.txt')
This code creates a new file named example.txt
and opens it for writing. If the file already exists, its contents will be overwritten.
Creating Directories
To create a new directory, you can use the os.mkdir()
function:
import os
def create_dir(dirname):
os.mkdir(dirname)
create_dir('mydir')
This code creates a new directory named mydir
.
Combining File and Directory Creation
If you want to create both a file and a directory at once, you can use the following code:
import os
def create_file_and_dir(filename, dirname):
if not os.path.exists(dirname):
os.mkdir(dirname)
with open(os.path.join(dirname, filename), 'w') as f:
pass # do nothing, just open the file in write mode
create_file_and_dir('example.txt', 'mydir')
This code creates a new directory mydir
if it doesn't exist, and then creates a new file named example.txt
inside that directory.
Tips and Variations
Useos.path.join()
to ensure that the filename is correctly joined with the directory path. To create directories recursively (i.e., create parent directories if they don't exist), use the os.makedirs()
function instead of os.mkdir()
. You can also specify additional permissions when creating files or directories using the os.umask()
function.
I hope this helps! Let me know if you have any questions or need further assistance.