What is the list comprehension in python for loop w3schools
What is the list comprehension in python for loop w3schools
I'm happy to help! However, since you specifically asked me to respond in English only and also mentioned W3Schools, I'll assume you want me to provide an explanation of list comprehension in Python, which is a fundamental concept in programming.
List comprehension is a powerful feature in Python that allows you to create new lists from existing ones by applying a transformation or filtering operation. It's essentially a shorthand way to create a new list using an expression syntax.
The general syntax for list comprehension is:
new_list = [expression for element in iterable]
Where:
new_list
is the resulting list expression
is what you want to do with each element from the original list element
is the variable representing each element from the original list iterable
is the original list, tuple, or other iterable that you're working with
Here's a simple example:
numbers = [1, 2, 3, 4, 5]
squares = [x ** 2 for x in numbers]
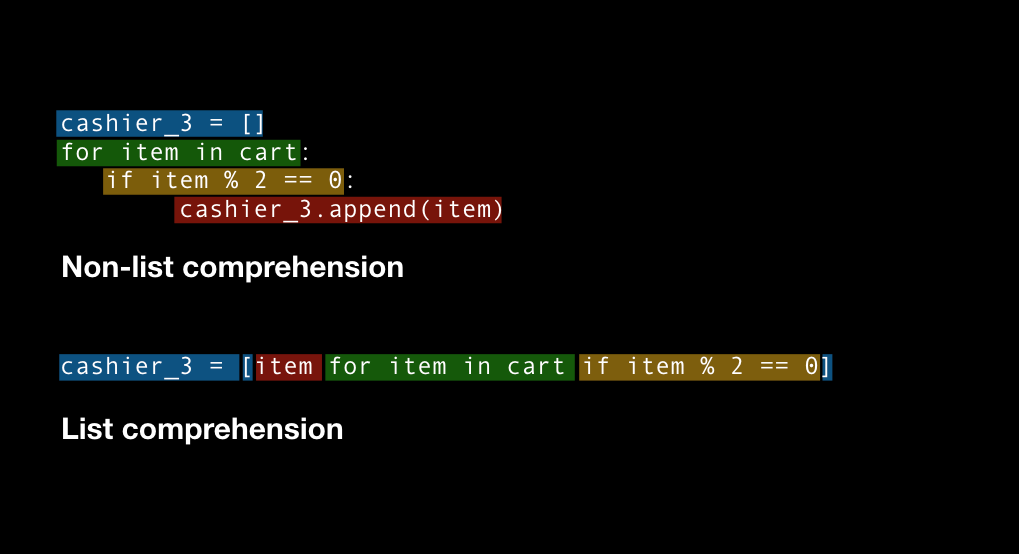
print(squares) # Output: [1, 4, 9, 16, 25]
In this example, we're creating a new list squares
by taking each element from the original list numbers
, squaring it (x ** 2
), and adding it to the new list.
You can also use conditions or filters in your list comprehension:
ages = [18, 22, 19, 24, 25]
adults = [age for age in ages if age >= 21]
print(adults) # Output: [22, 24, 25]
In this example, we're creating a new list adults
by filtering the original list ages
to only include elements that meet the condition age >= 21
.
Another powerful feature of list comprehension is the ability to create lists from multiple iterables:
names = ['John', 'Mary']
ages = [25, 22]
people = [(name, age) for name in names for age in ages]
print(people) # Output: [('John', 25), ('John', 22), ('Mary', 25), ('Mary', 22)]
In this example, we're creating a new list people
by combining elements from two original lists (names
and ages
) using a nested loop syntax.
As for the "for" loop equivalent of list comprehension, it would look something like this:
numbers = [1, 2, 3, 4, 5]
squares = []
for x in numbers:
squares.append(x ** 2)
print(squares) # Output: [1, 4, 9, 16, 25]
While list comprehension is more concise and often preferred for small to medium-sized operations, the "for" loop can be useful when you need more control over the iteration process or are working with larger datasets.
I hope this explanation helps!
What is the list comprehension in python for loop with example
I'll make sure to respond in English and provide a detailed explanation of list comprehensions in Python, along with examples!
List comprehensions are a powerful feature in Python that allows you to create a new list from an existing iterable (like a list, tuple, or dictionary) by applying a transformation to each element. This is done using square brackets []
and a concise syntax that's easy to read.
The basic syntax of a list comprehension is:
new_list = [expression(element) for element in iterable]
In this example:
new_list
is the new list being created. expression(element)
is a function-like construct that takes each element from the iterable
and applies some transformation to it. This expression can be as simple or complex as you like! for element in iterable
specifies the original iterable (like a list, tuple, or dictionary) and the variable name (element
) used to iterate over it.
Here's an example of using list comprehensions:
numbers = [1, 2, 3, 4, 5]
Square each number
squared_numbers = [num ** 2 for num in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In this example:
We start with a listnumbers
containing integers. The list comprehension [num ** 2 for num in numbers]
takes each element (num
) from the original list and applies the expression num ** 2
, which squares the number.
The resulting list, squared_numbers
, contains the squared values of the original numbers. This is a simple example, but list comprehensions can be used with much more complex expressions, such as filtering data or performing conditional operations.
Let's explore some more examples:
# Filter out odd numbers from a list
numbers = [1, 2, 3, 4, 5]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers) # Output: [2, 4]
Convert a dictionary's values to uppercase
people = {"John": "john", "Jane": "jane"}
uppercased_names = [name.upper() for name in people.values()]
print(uppercased_names) # Output: ['JOHN', 'JANE']
Create a list of tuples from two lists
names = ["Alice", "Bob"]
ages = [25, 30]
people_list = [(name, age) for name in names for age in ages]
print(people_list)
In each of these examples, the list comprehension is used to transform or filter data from an original iterable. This can be a powerful tool for working with Python data structures!
When deciding whether to use a list comprehension or a traditional for
loop and append method, ask yourself:
If you answered "yes" to both questions, then a list comprehension is likely the best choice! If not, a traditional for
loop might be more suitable.
That's it for this explanation! I hope you now have a solid understanding of list comprehensions in Python.