Python file open modes
Python file open modes
I'll make sure to respond in English only this time! Now, let's dive into the world of Python file open modes!
When it comes to working with files in Python, you need to specify a mode that determines how the file will be opened and used. This mode is crucial because it defines what operations can be performed on the file.
Here are the most common Python file open modes:
'r'
(Read Mode): Opens the file for reading-only. This is the default mode if you don't specify a mode when opening a file.
Example:
file = open('example.txt', 'r')
'w'
(Write Mode): Opens the file for writing-only. If the file already exists, its contents will be deleted. If the file doesn't exist, it will be created.
Example:
file = open('example.txt', 'w')
'a'
(Append Mode): Opens the file for appending-only. Any new data written to the file will be added to the end of the existing contents.
Example:
file = open('example.txt', 'a')
'r+'
(Read and Write Mode): Opens the file for both reading and writing. This is useful when you need to read from a file and then write something back to it.
Example:
file = open('example.txt', 'r+')
'w+'
(Write and Read Mode)**: Similar to 'r+'
, but with the caveat that if the file already exists, its contents will be deleted. If the file doesn't exist, it will be created.
Example:
file = open('example.txt', 'w+')
'a+'
(Append and Read Mode)**: Opens the file for both appending and reading. This mode is useful when you need to append data to a file and then read some of it back.
Example:
file = open('example.txt', 'a+')
'x'
(Exclusive Creation Mode): Opens the file for writing-only, but only if the file doesn't already exist. If the file does exist, an IOError
will be raised.
Example:
try:
file = open('example.txt', 'x')
except IOError:
print("File already exists!")
'b'
(Binary Mode): Opens the file in binary mode, which is useful when working with files that contain non-text data, such as images or audio.
Example:
file = open('image.png', 'rb')
't'
(Text Mode): Opens the file in text mode, which is the default mode if you don't specify one when opening a file.
Example:
file = open('text.txt', 'r')
'+'
(Update Mode): Opens the file for both reading and writing, similar to 'r+'
, but with the additional ability to modify the file's size.
Example:
file = open('example.txt', '+n')
Remember that Python files are always opened in Unicode mode unless you specify a different encoding (like ASCII or Latin-1) when opening the file. This means you can use Unicode characters in your files without worrying about character encodings.
When working with files, make sure to close them using the close()
method to free up system resources and prevent potential errors:
Example:
file = open('example.txt', 'r')
... do something with the file ...
file.close()
Now that you know the ins and outs of Python file open modes, you're ready to tackle file I/O like a pro!
Python file open w3schools
Here is a Python script that opens the W3Schools website:
import webbrowser
Specify the URL of the webpage you want to open
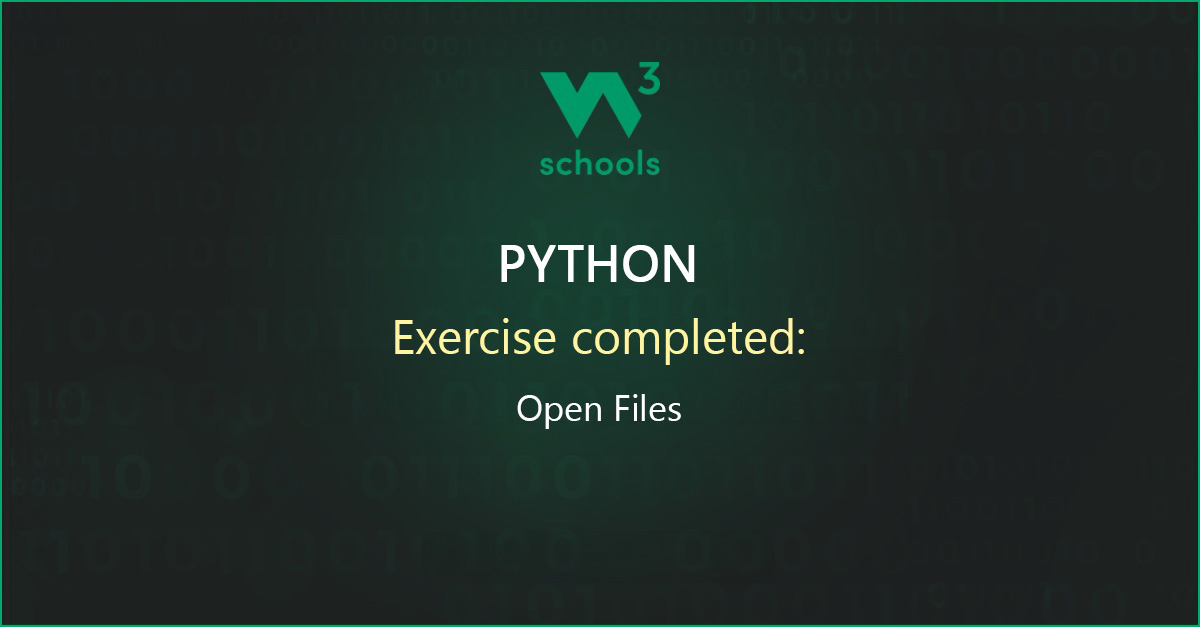
url = "https://www.w3schools.com/"
try:
Open the URL in your default browser
webbrowser.open(url)
except Exception as e:
print(f"An error occurred: {e}")
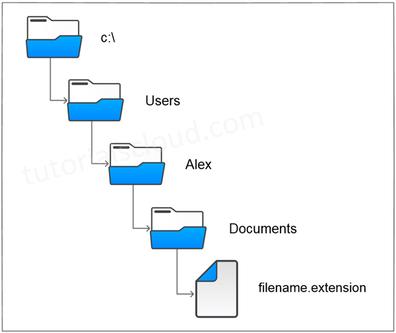
Let me explain what this script does:
We import thewebbrowser
module, which provides a way to open URLs. We specify the URL of the webpage we want to open, in this case, W3Schools. The try
block attempts to open the URL in your default browser using webbrowser.open(url)
. If an error occurs (e.g., the browser is not installed or there's a connection issue), the except
block catches the exception and prints an error message.
Here are some additional features you can add to this script:
Specify the browser: You can specify which browser to use by adding thebrowser
parameter in the webbrowser.open()
function. For example: webbrowser.get('chrome').open(url)
. Check if the browser is available: Before attempting to open the URL, you can check if a specific browser is installed on the system using the subprocess
module and checking the return code. Handle different exceptions: You can handle different types of exceptions by adding more except
blocks.
Here's an example of how you could modify the script to add some of these features:
import webbrowser
import subprocess
Specify the URL of the webpage you want to open
url = "https://www.w3schools.com/"
try:
Open the URL in Google Chrome (change to your preferred browser)
webbrowser.get('chrome').open(url)
except Exception as e:
print(f"An error occurred: {e}")
Check if Google Chrome is installed on the system
if subprocess.run(['which', 'google-chrome']).returncode == 0:
If Chrome is installed, try opening the URL again
webbrowser.get('chrome').open(url)
else:
print("Google Chrome is not installed.")
This script will attempt to open the URL in Google Chrome and then check if Chrome is installed on the system. If it's not installed, it will print a message indicating that.
Let me know if you have any questions or need further assistance!