Python rest api framework example
Python rest api framework example
Here's an example of a REST API framework using Python and Flask:
Firstly, we need to install the required packages:
pip install flask
pip install requests
Next, create a new Python file (e.g., app.py
) and add the following code:
from flask import Flask, jsonify, request
app = Flask(name)
Sample data
data = {
"users": [
{"id": 1, "username": "user1", "email": "[email protected]"},
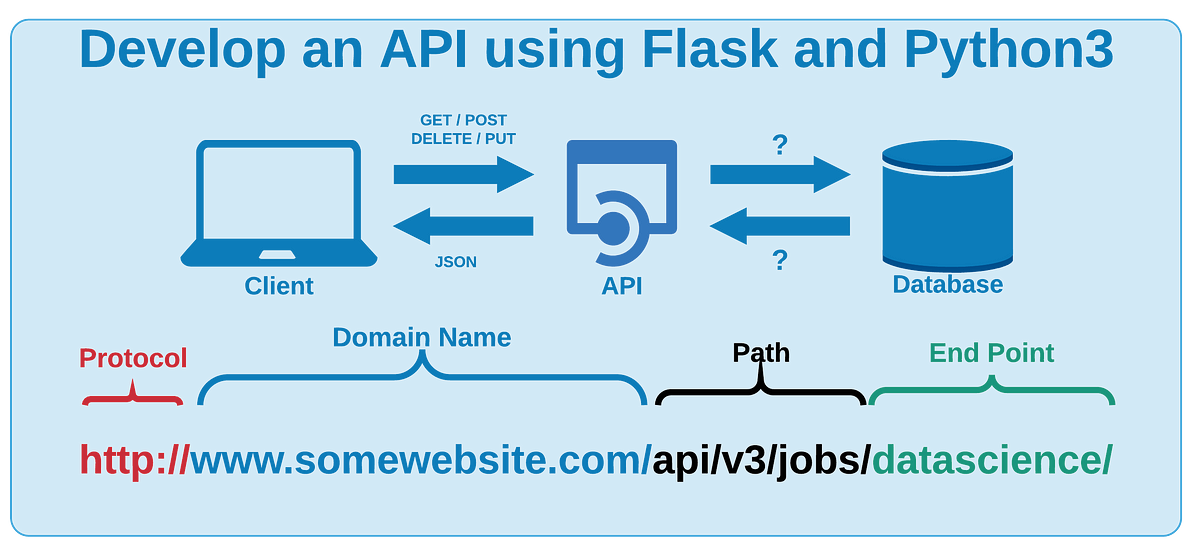
{"id": 2, "username": "user2", "email": "[email protected]"},
{"id": 3, "username": "user3", "email": "[email protected]"}
]
}
API endpoint for getting all users
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(data['users'])
API endpoint for getting a specific user by ID
@app.route('/users/int:user_id', methods=['GET'])
def get_user(user_id):
for user in data['users']:
if user['id'] == user_id:
return jsonify(user)
return jsonify({"error": "User not found"})
API endpoint for creating a new user
@app.route('/users', methods=['POST'])
def create_user():
new_user = {
"id": len(data['users']) + 1,
"username": request.get_json()['username'],
"email": request.get_json()['email']
}
data['users'].append(new_user)
return jsonify({"message": "User created successfully"})
API endpoint for updating a specific user
@app.route('/users/int:user_id', methods=['PUT'])
def update_user(user_id):
for i, user in enumerate(data['users']):
if user['id'] == user_id:
data['users'][i] = {
"id": user_id,
"username": request.get_json()['username'],
"email": request.get_json()['email']
}
return jsonify({"message": "User updated successfully"})
return jsonify({"error": "User not found"})
API endpoint for deleting a specific user
@app.route('/users/int:user_id', methods=['DELETE'])
def delete_user(user_id):
for i, user in enumerate(data['users']):
if user['id'] == user_id:
del data['users'][i]
return jsonify({"message": "User deleted successfully"})
return jsonify({"error": "User not found"})
if name == 'main':
app.run(debug=True)
In this example, we have five API endpoints:
/users
: Returns a list of all users. /users/<int:user_id>
: Returns the user with the given ID. /users
: Creates a new user and adds them to the list. /users/<int:user_id>
: Updates the user with the given ID. /users/<int:user_id>
: Deletes the user with the given ID.
To use these endpoints, you can make HTTP requests to the relevant URLs using tools like curl
or Postman. For example:
curl http://localhost:5000/users
Get a specific user by ID: curl http://localhost:5000/users/1
Create a new user: curl -X POST -H "Content-Type: application/json" -d '{"username": "newuser", "email": "[email protected]"}' http://localhost:5000/users
Update a specific user: curl -X PUT -H "Content-Type: application/json" -d '{"username": "updateduser", "email": "[email protected]"}' http://localhost:5000/users/1
Delete a specific user: curl -X DELETE http://localhost:5000/users/1
Note that this is just a simple example, and you would want to add error handling and other features depending on your use case.
Python REST client
Here is an introduction to using a Python REST client:
Python's built-in HTTP libraries make it easy to interact with RESTful APIs. In this response, I will cover the basics of creating a Python REST client and how to use it to send requests to a server.
What is a REST Client?
A REST (Representational State of Resource) client is a program that sends HTTP requests to a server in order to interact with a web-based API. The server should be designed to follow the principles outlined in Roy Fielding's 2000 doctoral dissertation, which defined REST as an architectural style.
Python's requests
Library
The most popular Python library for making HTTP requests is requests
. You can install it using pip:
pip install requests
Here are some basic usage examples of the requests
library:
To make a GET request, use the following code:
import requests
response = requests.get('http://www.example.com')
print(response.status_code) # Output: 200
print(response.text)
In this example, we're making a GET request to http://www.example.com
and printing out the status code (which should be 200 if everything goes as planned) and the response text.
To make a POST request, use the following code:
import requests
response = requests.post('http://www.example.com', data={'key': 'value'})
print(response.status_code)
In this example, we're making a POST request to http://www.example.com
with some key-value pairs in the request body.
To send data in JSON format, use the following code:
import requests
import json
data = {'key': 'value'}
response = requests.post('http://www.example.com', json=data)
print(response.json()) # Output: {'key': 'value'}
In this example, we're making a POST request to http://www.example.com
with some JSON data. The response will contain the same JSON data that was sent in the request.
To get the full response from the server (including headers and status code), use the following code:
import requests
response = requests.get('http://www.example.com')
print(response.headers) # Output: {'Content-Type': 'text/plain', ...}
print(response.status_code) # Output: 200
In this example, we're making a GET request to http://www.example.com
and printing out the response headers and status code.
To handle any errors that may occur when sending requests, you can use the following code:
import requests
try:
response = requests.get('http://www.example.com')
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
In this example, we're making a GET request to http://www.example.com
and trying to catch any exceptions that may occur during the process. If an error does occur, it will be printed out.
This is just a basic overview of how to use Python's requests
library to send HTTP requests. You can find more information on the official documentation: https://docs.python-requests.org/en/master/.