What is the difference between class and global variables in Python?
What is the difference between class and global variables in Python?
I'd be happy to explain the difference between class and global variables in Python!
Class Variables
In Python, a class variable (also known as a static variable) is a variable that is shared by all instances of a particular class. A class variable is defined inside the class definition using the cls
keyword (or self
for instance methods). This type of variable is not specific to any instance of the class and remains the same throughout the program.
For example:
class MyClass:
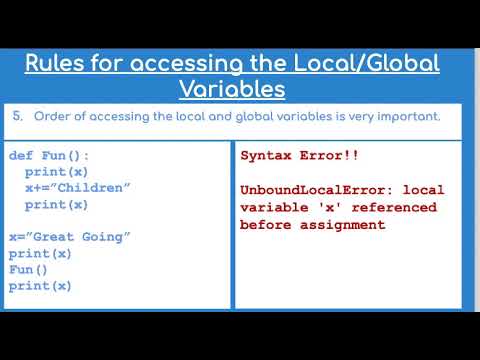
my_class_var = "Hello"
obj1 = MyClass()
obj2 = MyClass()
print(obj1.my_class_var) # Output: Hello
print(obj2.my_class_var) # Output: Hello
MyClass.my_class_var = "Goodbye"
print(obj1.my_class_var) # Output: Goodbye
print(obj2.my_class_var) # Output: Goodbye
As shown above, class variables can be accessed and modified directly from the class itself or its instances.
Global Variables
A global variable is a variable that is defined outside any function or method. It has access to all parts of your program. Global variables are usually avoided in Python as they make it harder to track down issues with your code, but sometimes they're necessary for certain applications.
For example:
my_global_var = "Hello"
def my_function():
print(my_global_var)
print(my_global_var)
my_function()
In this case, the my_global_var
is accessible from both outside and inside the function. If you modify it within the function (like my_global_var = "Goodbye"
), its value will be changed for all parts of your program.
Key Differences
The main differences between class variables and global variables are:
Scope: Class variables are scoped to the class itself, while global variables have access to all parts of your program. Sharing: Class variables are shared by all instances of a particular class, whereas global variables can be accessed from anywhere in your program. Access: You can access and modify class variables directly from the class or its instances, while global variables are usually accessed through their name.In general, you should use class variables when you need to share data between instances of the same class, and use global variables when you really need something that's accessible across your program. However, it's generally considered better practice to avoid using global variables altogether in favor of classes or other more structured approaches to code organization.
When to use global variables in Python?
I'd be happy to provide an answer in English!
In Python, global variables can be used when you need to store data that should remain accessible throughout the execution of your program. Here are some scenarios where using global variables makes sense:
Configuration settings: You might have a set of configuration parameters that you want to use throughout your program. For instance, if you're building a web scraper, you might want to specify the target website and the type of data you want to collect. Global variables can be used to store these settings. Database connections: When working with databases, you often need to establish a connection that remains active throughout your program's execution. Global variables can be used to store database connection details, such as the host, port, username, and password. Singleton instances: In some cases, you might want to create a single instance of an object that should remain accessible throughout your program. This is often referred to as the "singleton pattern." Global variables can be used to implement this pattern. Constants: You might have a set of constants that define fundamental values or settings for your program. For example, you could store the number of seconds in a minute or the name of a default font. Global variables can be used to define these constants.However, it's essential to note that global variables should be used sparingly and with caution. Overusing global variables can lead to:
Namespace pollution: When you use too many global variables, your namespace becomes cluttered, making it harder to find the code you're looking for. Code readability: Global variables can make your code harder to read, as their usage is not explicitly declared within a specific function or scope. Maintenance difficulties: As your program grows, maintaining and debugging global variables can become challenging.To use global variables effectively in Python, follow these best practices:
Use them sparingly: Limit the number of global variables you use to only what's necessary for your program's functionality. Document their usage: Clearly document where and how you're using each global variable to ensure readability and maintainability. Avoid modifying them accidentally: Use caution when updating or modifying global variables, as changes can have unintended consequences throughout your program.In summary, while global variables can be useful in certain situations, it's crucial to use them thoughtfully and with consideration for the potential drawbacks. By doing so, you'll maintain a clean, readable, and maintainable codebase that is easy to work with.