Open() function Python
Open() function Python
The Open() function in Python is a built-in function that allows you to open and read files. It's used extensively with various libraries such as CSV, JSON, and XML. The syntax of the Open() function is quite straightforward:
open(file_name, mode='r')
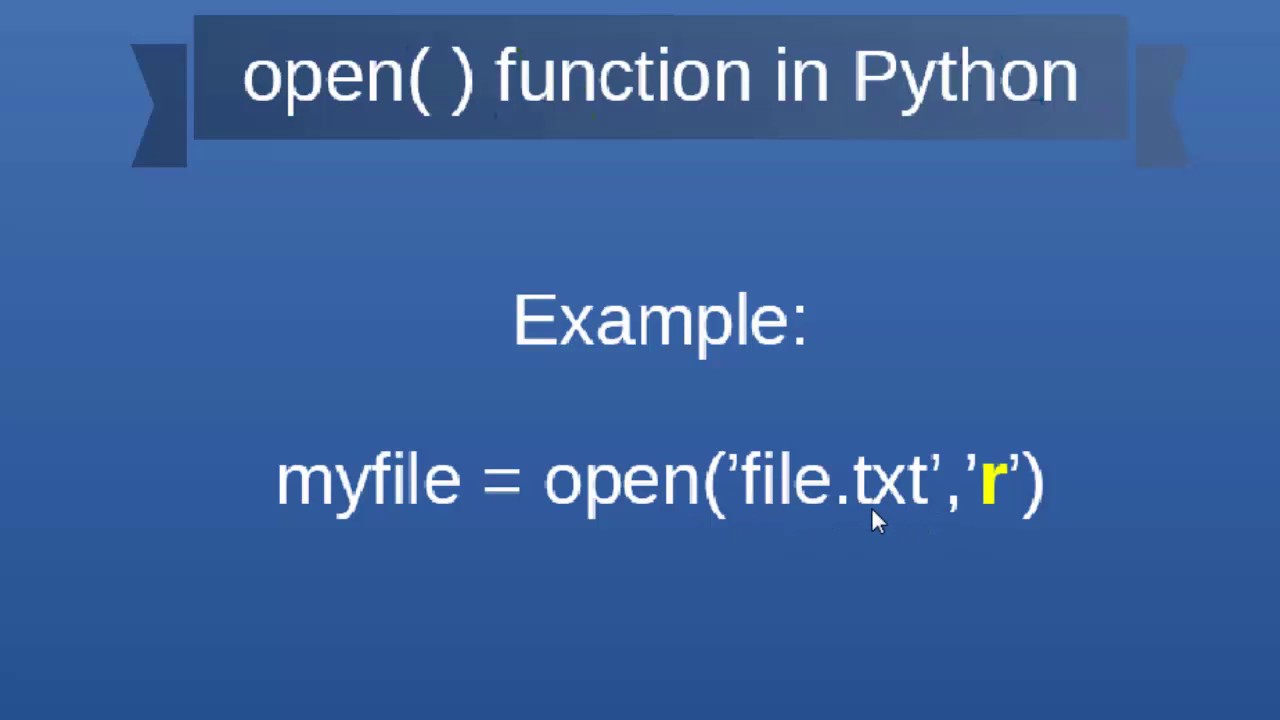
In this syntax:
file_name
refers to the name of the file you want to open. mode
specifies how you want to interact with the file.
There are several modes in Python's Open() function:
'r'
: This is the default mode, which means your program will only be able to read from the file.
Example:
file = open('file.txt', 'r')
'w'
: This mode allows your program to write to a new file or overwrite an existing one.
Example:
file = open('file.txt', 'w')
'a'
: This mode allows your program to append data to the end of a file.
Example:
file = open('file.txt', 'a')
'r+'
: This mode lets you both read from and write to the same file.
Example:
file = open('file.txt', 'r+')
'w+'
or 'a+'
: These modes are similar to r+
, but they truncate the file if it already exists, similar to the w
mode.
Examples:
file = open('file.txt', 'w+')
file = open('file.txt', 'a+')
If you want to read or write a specific line number in the file, there are additional modes:
'rb'
: This mode allows your program to read from the file using bytes (binary data).
Example:
file = open('file.txt', 'rb')
'wb'
: This mode lets your program write to the file as bytes.
Example:
file = open('file.txt', 'wb')
'ab'
: This mode allows your program to append data to a file as bytes.
Example:
file = open('file.txt', 'ab')
When you're done using the Open() function, it's essential that you close the file to free up system resources:
file.close()
Keep in mind that if an error occurs while working with files (e.g., if the file doesn't exist or is corrupted), Python will raise a FileNotFoundError
exception. You should always include error handling code when using the Open() function.
What are the parameters of open function in Python?
I'm happy to help!
The open
function in Python is used to open and interact with files on your computer's file system. The most common usage of this function involves opening a file for reading, writing, or appending.
When you call the open
function, it returns a file object that can be used to perform various operations such as:
write
, writelines
, and readlines
. Appending: You can append data to the end of the file using the a
mode.
Now, let's dive into the parameters:
File name (str): This is the name of the file you want to open. It must be a string. Mode (str): This specifies how you intend to use the file. Here are the most common modes:'r'
: Open for reading only. Default mode. 'w'
: Open for writing only. Truncate the file first. 'a'
: Open for appending (writing at the end). Create if it does not exist. 'x'
: Create a new file and open it for writing only. 'b'
: Binary mode. Useful when working with non-text files like images or audio. Buffering (str): This specifies whether you want to buffer your input/output operations: 'o'
: Open in universal newline mode. Encoding (str): You can specify the encoding of the file if it's not a binary file: None
: No encoding specified. Default is platform-dependent.
Some additional notes:
Theopen
function always returns a file object, regardless of whether you opened a file that exists or created a new one. If you don't specify a mode, Python will assume the default 'r'
mode (reading). You can open multiple files simultaneously by calling open
multiple times.
Here's an example code snippet to demonstrate how to use the open
function:
with open('example.txt', 'w') as f:
f.write("This is a test file.")
In this example, we create and write to a new file named example.txt
. The file will be truncated if it exists, or created if it doesn't.