How to read file line by line using for loop in Python?
How to read file line by line using for loop in Python?
I'd be happy to explain how to read a file line by line using a for loop in Python!
Reading files line by line is a common task in many programming languages, including Python. In this case, we'll use the open()
function and the for
loop to iterate over each line in the file.
Let's start with an example. Suppose you have a text file named "example.txt" with the following content:
Hello, world!
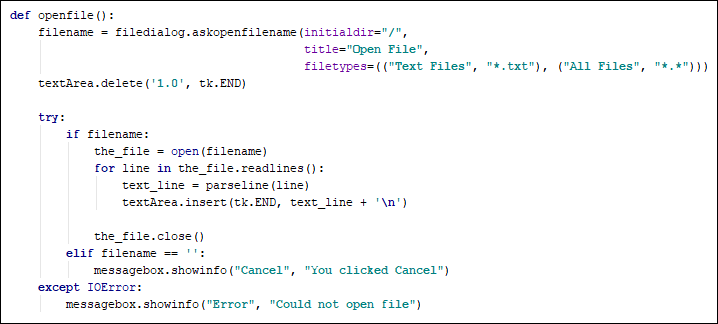
This is just a test.
It has multiple lines.
To read this file line by line using a for loop, we'll follow these steps:
Open the file: Use theopen()
function to open our text file. This function requires two parameters: the filename and mode ('r' for reading). We can store the file object in a variable like file
.
with open('example.txt', 'r') as file:
Read the file line by line using a for loop
pass
Read the file line by line: Use the for
loop to iterate over each line in the file. We can use the readline()
method of our file object to read one line at a time.
with open('example.txt', 'r') as file:
for line in file:
Process the current line
pass
Process the lines: Inside the loop, we can print each line using print(line.strip())
. The strip()
method removes any leading or trailing whitespace.
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
Here's our final code:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
Now, when you run this Python script, it will read the lines from your text file and display each line separately. For example, the output would be:
Hello, world!
This is just a test.
It has multiple lines.
That's it! We've successfully read our text file line by line using a for loop in Python.
How to read file line by line in while loop Python?
Here's a step-by-step guide on how to read a file line by line in Python using a while
loop.
Step 1: Open the File
To start, you need to open the file you want to read. You can do this using the built-in open()
function in Python. The syntax is open(filename, mode)
, where filename
is the name of your file and mode
is the way you want to open it. In this case, we'll use 'r'
as our mode, which means we're opening the file for reading.
Here's how to do it:
file = open('example.txt', 'r')
Replace 'example.txt'
with your own file name.
Step 2: Read the File Line by Line
Now that we have our file open, let's read it line by line. We'll use a while
loop to do this. The idea is that we keep reading lines from the file as long as there are more lines to read. When we've reached the end of the file, the loop will exit.
Here's how to do it:
line = file.readline()
while line:
Do something with each line
print(line.strip())
line = file.readline()
Let's break this down:
file.readline()
reads the next line from the file. If there are no more lines, it returns an empty string (''
). The while
loop continues as long as line
is not empty. Inside the loop, we print each line using print(line.strip())
. The strip()
method removes any leading or trailing whitespace from the line. We then read the next line and repeat the process until there are no more lines.
Step 3: Close the File
When you're done reading the file, don't forget to close it! This is important because files can be locked by other processes if they remain open. To close a file, simply use the close()
method:
file.close()
Here's the complete code:
file = open('example.txt', 'r')
line = file.readline()
while line:
print(line.strip())
line = file.readline()
file.close()
This is how you read a file line by line in Python using a while
loop. Remember to replace 'example.txt'
with your own file name!
Tips and Variations
You can also use thefor
loop instead of a while
loop: for line in file:
. If your file has a specific format, you might want to split each line using the split()
method or regular expressions. For larger files, it's a good idea to process each line as you read it rather than storing them all in memory at once.
I hope this helps! Let me know if you have any questions.