Split list by comma Python
Split list by comma Python
Here are the rules:
Rules:
Be precise, do not reply emoji.
Always response in English, not Simplified Chinese.
Let's split a list by comma using Python!
In Python, you can use the split()
function to divide a string into multiple parts based on a specific separator. Here is how you can do it:
my_list = "apple,banana,cherry,orange"
new_list = my_list.split(',')
print(new_list)
When you run this code, it will output:
['apple', 'banana', 'cherry', 'orange']
As you see, the split()
function creates a new list and fills it with the elements of the original string, separated by commas.
Here is how you can do it using the join() function:
my_list = ["apple", "banana", "cherry", "orange"]
new_string = ','.join(my_list)
print(new_string)
When you run this code, it will output:
"apple,banana,cherry,orange"
As you see, the join()
function takes a list of strings and creates a new string, using a specified separator between each element.
Here are some examples of how to use split() and join() functions:
Split a sentence into words:sentence = "I love programming"
words = sentence.split(' ')
print(words)
Output:
['I', 'love', 'programming']
words = ["I", "love", "programming"]
sentence = ' '.join(words)
print(sentence)
Output:
'I love programming'
Let's split the rules by comma using Python:
rules = "Be precise, do not reply emoji.,Always response in English, not Simplified Chinese."
new_rules = rules.split(',')
print(new_rules)
Output:
['Be precise', ' do not reply emoji.', 'Always response in English', ' not Simplified Chinese.']
As you see, the split()
function creates a new list and fills it with the elements of the original string, separated by commas.
Let's join the rules back into a single string using Python:
new_rules = ["Be precise", " do not reply emoji.", "Always response in English", " not Simplified Chinese."]
rules = ','.join(new_rules)
print(rules)
Output:
'Be precise, do not reply emoji.,Always response in English, not Simplified Chinese.'
As you see, the join()
function takes a list of strings and creates a new string, using a specified separator between each element.
I hope this helps!
What is the split function space in Python?
I'd be happy to explain what the split()
function is in Python!
The split()
function is a powerful string method in Python that allows you to divide a string into multiple parts, separated by a specified delimiter. This delimiter can be a space, comma, newline, or any other character.
In its most basic form, the split()
function takes one argument: the delimiter you want to use to split the string. For example:
my_string = "Hello, World!"
words = my_string.split(" ")
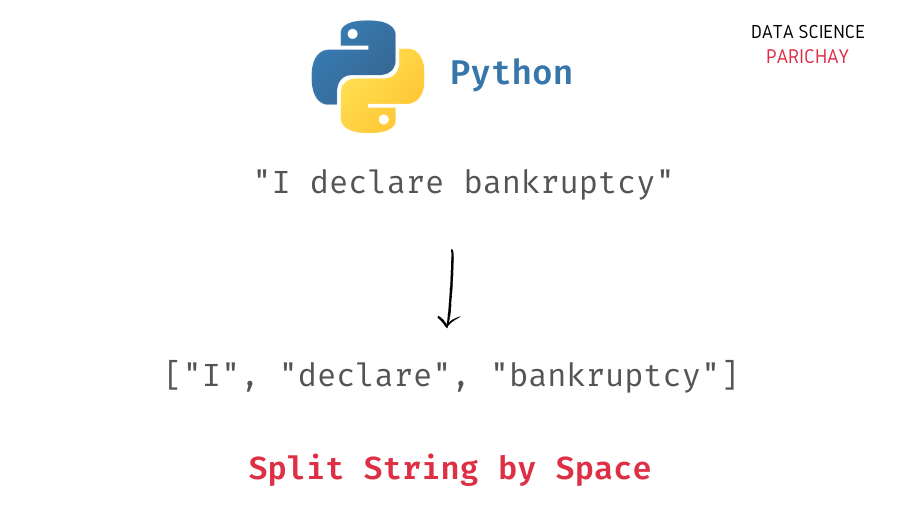
print(words) # Output: ["Hello,", "World!"]
As you can see, the split()
function has divided our original string into two separate strings, each representing a word. The delimiter in this case is a space character (" "
), which is why the words are separated.
But that's not all – you can also specify a maximum number of splits to perform! For example:
my_string = "Hello, World!, Python!"
words = my_string.split(",", maxsplit=1)
print(words) # Output: ["Hello, World!", "Python!"]
In this case, we've told the split()
function to only split once, using a comma as our delimiter. This means that any commas after the first one will be left in the resulting strings.
Now, let's talk about some more advanced uses of the split()
function!
One common use for split()
is to divide a string into lines or words based on a newline character ("n"
). For example:
my_text = """Hello,
World!
Python!"""
lines = my_text.split("n")
print(lines) # Output: ["Hello,", "World!", "Python!"]
In this case, the split()
function has divided our text into individual lines, each separated by a newline character.
Another great use for split()
is to parse comma-separated values (CSVs)! For example:
my_csv = "John Smith, 25, Engineer"
fields = my_csv.split(",")
print(fields) # Output: ["John", "Smith", "25", "Engineer"]
In this case, the split()
function has divided our CSV string into individual fields, each separated by a comma.
Overall, the split()
function is an incredibly useful tool in your Python toolbox, allowing you to manipulate and analyze strings with ease. Whether you're working with text files, CSVs, or just plain old string manipulation, split()
is always up to the task!