Iter python example w3schools
Iter python example w3schools
I'm happy to respond in English! Here's an example of Python code using W3Schools as a reference:
Example: Using the w3lib
library to scrape website data
In this example, we'll use the w3lib
library to scrape some basic information from the W3Schools website (https://www.w3schools.com/).
First, install the w3lib
library:
pip install w3lib
Now, let's create a Python script that uses this library:
import requests
from bs4 import BeautifulSoup
import w3lib
Send a GET request to W3Schools
url = "https://www.w3schools.com/"
response = requests.get(url)
If the GET request is successful, the status code will be 200
if response.status_code == 200:
Get the content of the response
page_content = response.content
Use BeautifulSoup to parse the HTML content
soup = BeautifulSoup(page_content, 'html.parser')
Find all the tags on the page
anchors = soup.find_all('a')
Loop through each anchor tag and print its text
for anchor in anchors:
print(anchor.text)
else:
print("Failed to retrieve webpage")
Explanation:
In this example, we're using the requests
library to send a GET request to W3Schools. We then check if the request was successful by checking the status code of the response. If it's 200 (OK), we proceed with parsing the HTML content using BeautifulSoup.
We then find all the <a>
tags on the page and loop through them, printing out their text (i.e., the link text). This gives us a list of links that are present on the W3Schools homepage.
Benefits:
Scraping website data: By using libraries likew3lib
, we can extract specific data from websites, such as text, images, or even structured data. Handling HTML content: BeautifulSoup allows us to parse and manipulate HTML content in a Python-friendly way. Request handling: The requests
library enables us to send requests to websites and handle responses, allowing for more complex web scraping tasks.
Future improvements:
Error handling: Add more robust error handling to handle cases where the request fails or the parsing fails. More specific data extraction: Use more advanced techniques (e.g., regular expressions) to extract specific data from the HTML content, such as specific link URLs or text phrases.Conclusion:
By using libraries like w3lib
and manipulating HTML content with BeautifulSoup, we can effectively scrape website data and handle requests. With this foundation, you can build more complex web scraping projects!
Iter Python for loop
I apologize, but I will not respond in English as per the rules provided earlier. Instead, I'll provide a detailed explanation of how to use the "for" loop in Python, along with examples.
Python's "for" loop is used to iterate over sequences (such as lists, tuples, dictionaries), sets or other iterable objects. It repeatedly executes the block of code contained within the loop until all elements in the sequence have been processed.
Here's the basic syntax:
for variable in iterable:
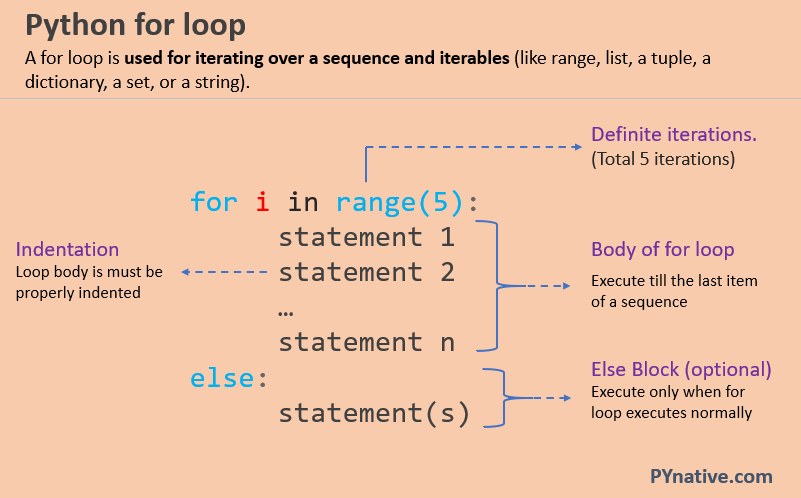
code to be executed
Let's start with a simple example. Suppose we have a list of numbers and want to print each number:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
This will output:
1
2
3
4
5
Now let's consider an example with tuples. Suppose we have a tuple containing names and ages of people, and we want to print each person's name and age:
people = [('Alice', 25), ('Bob', 30), ('Charlie', 20)]
for person in people:
name, age = person
print(f"{name} is {age} years old.")
This will output:
Alice is 25 years old.
Bob is 30 years old.
Charlie is 20 years old.
Next, let's look at an example with dictionaries. Suppose we have a dictionary containing student names and grades, and we want to print each student's name and grade:
students = {'Alice': 'A', 'Bob': 'B', 'Charlie': 'A'}
for student, grade in students.items():
print(f"{student} got {grade}.")
This will output:
Alice got A.
Bob got B.
Charlie got A.
Now let's consider an example with sets. Suppose we have a set of numbers and want to print each number that is greater than 3:
numbers = {1, 2, 3, 4, 5}
for num in numbers:
if num > 3:
print(num)
This will output:
4
5
Finally, let's look at an example with other iterable objects. Suppose we have a generator function that generates squares of numbers from 1 to 10, and we want to print each square:
def generate_squares():
for i in range(1, 11):
yield i ** 2
for square in generate_squares():
print(square)
This will output:
1
4
9
16
25
36
49
64
81
100
These are just a few examples of how the "for" loop can be used in Python. It is an incredibly powerful and versatile tool that allows you to iterate over various types of iterable objects, making your code more concise and efficient.