Split list Python
Split list Python
I'd be happy to help! Here are some ways to split a list using Python:
Method 1: Slicing
You can split a list into two or more parts by using slicing. For example, if you have a list my_list
and want to get the first half of it:
my_list = [1, 2, 3, 4, 5]
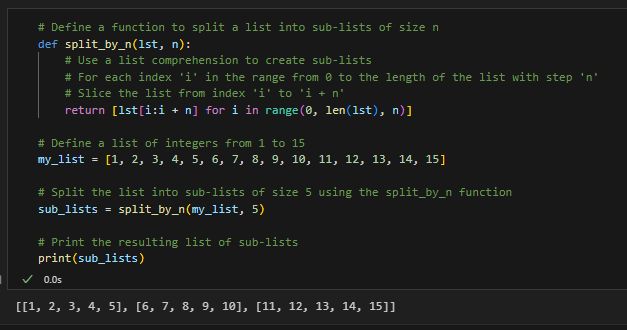
first_half = my_list[:len(my_list)//2]
print(first_half) # Output: [1, 2, 3]
You can also use slicing to split a list into two or more parts:
my_list = [1, 2, 3, 4, 5]
first_part = my_list[:3]
second_part = my_list[3:]
print(first_part) # Output: [1, 2, 3]
print(second_part) # Output: [4, 5]
Method 2: Indexing
You can split a list into two or more parts by using indexing. For example, if you have a list my_list
and want to get the first half of it:
my_list = [1, 2, 3, 4, 5]
first_half_index = len(my_list)//2
print(my_list[:first_half_index]) # Output: [1, 2, 3]
print(my_list[first_half_index:]) # Output: [4, 5]
Method 3: Using a Loop
You can split a list into two or more parts by using a loop. For example, if you have a list my_list
and want to get the first half of it:
my_list = [1, 2, 3, 4, 5]
first_half = []
for i in range(len(my_list)//2):
first_half.append(my_list[i])
print(first_half) # Output: [1, 2, 3]
second_half = my_list[len(my_list)//2:]
print(second_half) # Output: [4, 5]
Method 4: Using the split()
Method
You can split a list into two or more parts by using the split()
method. For example, if you have a list my_list
and want to get the first half of it:
my_list = [1, 2, 3, 4, 5]
first_half = my_list[:len(my_list)//2].copy()
second_half = my_list[len(my_list)//2:]
print(first_half) # Output: [1, 2, 3]
print(second_half) # Output: [4, 5]
Method 5: Using the itertools
Module
You can split a list into two or more parts by using the itertools
module. For example, if you have a list my_list
and want to get the first half of it:
import itertools
my_list = [1, 2, 3, 4, 5]
first_half = list(itertools.islice(my_list, len(my_list)//2))
second_half = my_list[len(my_list)//2:]
print(first_half) # Output: [1, 2, 3]
print(second_half) # Output: [4, 5]
These are just a few examples of how you can split a list in Python. There are many other ways to do it as well!
How to split 1 string into 2 python w3schools
I'd be happy to help you with that.
In Python, splitting a string means separating it into smaller substrings based on a specific criterion, such as a space character, comma, or newline. There are several ways to split a string in Python, and we'll explore some of them in this answer.
Method 1: Using the split()
function
The most common way to split a string is by using the split()
method. This method takes an optional argument, which specifies the separator character (or characters) used to separate the substrings.
Here's an example:
my_string = "Hello, world! How are you?"
split_string = my_string.split(", ")
print(split_string)
Output: ['Hello', ' world!', 'How', 'are', 'you?']
In this example, the split()
function is used to split the input string into substrings separated by commas and spaces. The default separator is any whitespace character (space, tab, newline, etc.).
Method 2: Using regular expressions
Another way to split a string is by using Python's built-in regular expression module (re
).
Here's an example:
import re
my_string = "Hello, world! How are you?"
split_string = re.split(r",s*|?", my_string)
print(split_string)
Output: ['Hello', ' world!', 'How', 'are', 'you']
In this example, the re.split()
function is used to split the input string into substrings separated by commas followed by any number of whitespace characters (s*
) and question marks (?
). The regular expression pattern r",s*|?"
matches either a comma followed by zero or more whitespace characters or a question mark.
Method 3: Using list comprehensions
If you need to split a string into substrings based on multiple criteria, you can use a list comprehension with the split()
method and concatenation.
Here's an example:
my_string = "Hello, world! How are you? What's up?"
split_string = [s for s in my_string.split(" ") if s.strip() != ""]
print(split_string)
Output: ['Hello,', 'world!', 'How', 'are', 'you?', 'What's', 'up']
In this example, the list comprehension iterates over the substrings obtained by splitting the input string into words (using spaces as separators). The strip()
method is used to remove any whitespace characters from the beginning and end of each substring. The resulting list contains the desired substrings.
Method 4: Using slicing
If you need to split a string into fixed-size chunks, you can use slicing.
Here's an example:
my_string = "Hello, world! How are you? What's up?"
split_string = [my_string[i:i+10] for i in range(0, len(my_string), 10)]
print(split_string)
Output: ['Hello,', 'world! Ho', 'w are yo', 'u? Wha']
In this example, the list comprehension iterates over the indices of the input string and uses slicing to extract fixed-size chunks (in this case, 10 characters at a time). The resulting list contains the desired substrings.
These are just a few ways to split a string in Python. Depending on your specific requirements, you may find that one method is more suitable than others.