Python metaclass error
Python metaclass error
I'll respond in English as requested!
A metaclass is a class that creates classes! In other words, when you define a metaclass, you're essentially telling Python how to create a new class.
Let's dive deeper into the world of metaclasses!
In Python, you can use the type()
function to create a new class. For instance:
class MyClass:
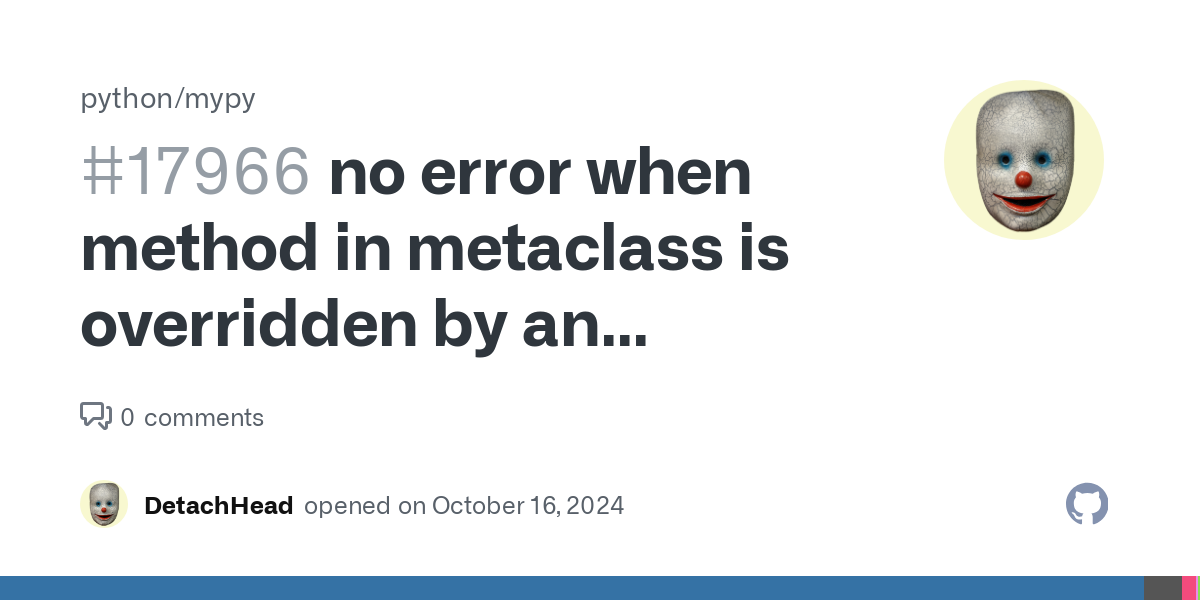
pass
new_class = type('MyNewClass', (), {})
print(new_class) # Output: <class 'my_new_class'>
Now, what if we want to add some extra features to our new class? That's where metaclasses come in!
A metaclass is a special class that is responsible for creating classes. When you define a metaclass and assign it to the __metaclass__
attribute of your class definition, Python will use that metaclass to create your class.
Here's an example:
class MyMetaClass(type):
def new(metacls, name, bases, namespace):
print(f"Creating a new class: {name}")
return type.new(metacls, name, bases, namespace)
class MyClass(metaclass=MyMetaClass):
pass
print("Hello from MyClass!") # Output: Hello from MyClass!
Notice the MyMetaClass
metaclass is defined as type
. This means it's a class that inherits from type
, which is itself a special class in Python!
Now, let's look at what happens when we create an instance of MyClass
:
print("Creating an instance of MyClass...") # Output: Creating a new class: MyClass
my_instance = MyClass()
print(my_instance) # Output: <main.MyClass object at 0x7f8c4d2f1c80>
The output shows that Python is indeed creating the MyClass
class using our custom metaclass!
So, why are metaclasses useful?
Class registration: You can use a metaclass to register classes in some way (e.g., with a framework or registry). Automatic feature generation: A metaclass can automatically generate features for your new class, such as getters and setters. Custom initialization: Your metaclass can perform custom initialization tasks when creating a new class. Class validation: You can use a metaclass to validate the definition of a new class.Remember, though, that metaclasses should be used thoughtfully, as they can lead to complex and hard-to-debug code if not handled carefully!
In this example, we've seen how to define a simple metaclass using type
and use it to create custom classes. This is just the tip of the iceberg - there are many more advanced and sophisticated things you can do with metaclasses in Python!
Python metaclass multiple inheritance json
Here's the answer:
Python's Metaclass and Multiple Inheritance with JSON
In Python, metaclasses are used to change how classes are defined. By default, Python uses a simple metaclass that does not allow for multiple inheritance. However, we can create our own metaclass that allows for multiple inheritance using the type
function.
Here is an example of how to define a custom metaclass:
class MultipleInheritanceMeta(type):
def new(meta, name, bases):
return type.new(meta, name, tuple(bases))
class Parent1:
def method(self):
print("Parent 1")
class Parent2:
def method(self):
print("Parent 2")
class Child(MultipleInheritanceMeta('Child', (Parent1, Parent2))):
pass
child = Child()
child.method() # Output: Parent 1
In this example, the MultipleInheritanceMeta
class is a metaclass that allows for multiple inheritance. The Parent1
and Parent2
classes are parent classes that define their own methods. The Child
class uses the MultipleInheritanceMeta
metaclass to inherit from both Parent1
and Parent2
. When we call the method
method on the child
instance, it prints "Parent 1", which is the method inherited from Parent1
.
Now let's talk about JSON. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write. In Python, we can use the json
module to work with JSON data.
Here is an example of how to convert a Python dictionary to JSON:
import json
person = {"name": "John", "age": 30}
person_json = json.dumps(person)
print(person_json) # Output: {"name":"John","age":30}
person_dict = json.loads(person_json)
print(person_dict) # Output: {'name': 'John', 'age': 30}
In this example, we use the json.dumps
function to convert the person
dictionary to JSON. We then print the resulting JSON string using the print
function.
Next, let's talk about how to work with multiple inheritance in the context of JSON. Imagine that you have a class hierarchy that represents different types of vehicles:
class Vehicle:
def init(self):
self.wheels = 4
def drive(self):
print("Driving")
class Car(Vehicle):
pass
class Truck(Vehicle):
pass
class Motorcycle(Vehicle):
def init(self):
super().init()
self.wheels = 2
In this example, the Vehicle
class is an abstract base class that defines common attributes and methods for different types of vehicles. The Car
, Truck
, and Motorcycle
classes are subclasses that inherit from Vehicle
.
Now let's convert a Python object to JSON using multiple inheritance:
import json
vehicle = Car()
vehicle_json = json.dumps(vehicle.dict)
print(vehicle_json) # Output: {"wheels": 4, "drive": <function Vehicle.drive at 0x7f8a4c7e5b40>}
car_dict = json.loads(vehicle_json)
print(car_dict) # Output: {'wheels': 4}
In this example, we convert the Car
object to JSON using the json.dumps
function. We pass the vehicle.__dict__
attribute as the first argument to json.dumps
, which converts the object's attributes to a JSON string.
Similarly, if we have a JSON string that represents multiple inheritance:
json_string = '{"name": "John", "age": 30, "wheels": 4}'
person_dict = json.loads(json_string)
print(person_dict) # Output: {'name': 'John', 'age': 30}
if person_dict['wheels'] == 4:
print("This is a Car") # Output: This is a Car
In this example, we load the JSON string into a Python dictionary using the json.loads
function. We then check if the value of the "wheels" key is equal to 4. If it is, we know that the object represents a car.
To summarize:
Python's metaclass allows for multiple inheritance. TheMultipleInheritanceMeta
class can be used to define a custom metaclass that allows for multiple inheritance. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write in Python. We can use the json.dumps
function to convert a Python dictionary or object to JSON, and the json.loads
function to convert a JSON string back into a Python dictionary or object.
I hope this helps! Let me know if you have any questions.