Python statements list w3schools
Python statements list w3schools
Here is the list of Python statements from W3Schools:
print("Hello, World!")
: This statement prints "Hello, World!" to the console.
x = 5
: This statement assigns the value 5 to the variable x. y = "hello"
: This statement assigns the string "hello" to the variable y. name = input("What is your name? ")
: This statement prompts the user for their name and assigns it to the variable name. age = int(input("How old are you? "))
: This statement prompts the user for their age, converts it to an integer, and assigns it to the variable age. print(x + y)
: This statement concatenates the values of x and y (which are 5 and "hello" respectively) and prints the result.
if age > 18: print("You are old enough!") else: print("You are too young!")
: This statement checks if the user's age is greater than 18, and prints out a message accordingly. for i in range(5): print(i)
: This statement loops through the numbers 0 to 4 (inclusive), printing each one. while x < 10: x += 1
: This statement starts with x equal to 5 and continues to increment it by 1 while it is less than 10. name = "John" if age > 18 else "Jane"
: This statement uses the ternary operator to assign either "John" or "Jane" to the variable name based on whether the user's age is greater than 18. print(f"The value of x is {x}")
: This statement prints out a message that includes the value of x using the f-string formatting. x, y = (3, 4)
: This statement unpacks the tuple (3, 4)
into two variables x and y. print(*"hello")
: This statement prints each character in the string "hello" as a separate output. a = [1, 2, 3]
: This statement creates a list with the elements 1, 2, and 3, and assigns it to the variable a. print(a[0])
: This statement prints the first element of the list a, which is 1. b = {"name": "John", "age": 30}
: This statement creates a dictionary with two key-value pairs and assigns it to the variable b. print(b["name"])
: This statement prints out the value corresponding to the key "name" in the dictionary b, which is "John". for key, value in b.items(): print(f"{key}: {value}")
: This statement loops through the items in the dictionary b and prints each one. c = [x**2 for x in range(5)]
: This statement creates a list of squares from 0 to 4 (inclusive) using a list comprehension. d = {i: i**2 for i in range(5)}
: This statement creates a dictionary with the same key-value pairs as c, but represented as a dictionary instead of a list. e = [(x, x**2) for x in range(5)]
: This statement creates a list of tuples where each tuple contains an integer and its square using a list comprehension. f = {i: (i, i**2) for i in range(5)}
: This statement creates a dictionary with the same key-value pairs as e, but represented as a dictionary instead of a list. g = [i for i in range(10) if i % 2 == 0]
: This statement creates a list of all even numbers from 0 to 9 (inclusive) using a list comprehension and an if statement. h = {i: "even" if i % 2 == 0 else "odd" for i in range(10)}
: This statement creates a dictionary where each key is an integer from 0 to 9, and the value is either "even" or "odd" depending on whether the number is even or odd. i = [1, 2, 3] + [4, 5, 6]
: This statement concatenates two lists together into a new list, resulting in [1, 2, 3, 4, 5, 6]
.What is a list statement in Python?
I'll make sure to respond in English for you.
In Python, a list statement is an expression that creates and initializes a list object. Lists are a fundamental data structure in Python, used to store collections of items that can be of any type, including strings, integers, floats, and other lists. A list statement typically begins with the word "list" or "[" followed by a series of values separated by commas.
Here is an example of a simple list statement:
my_list = [1, 2, 3, 4, 5]
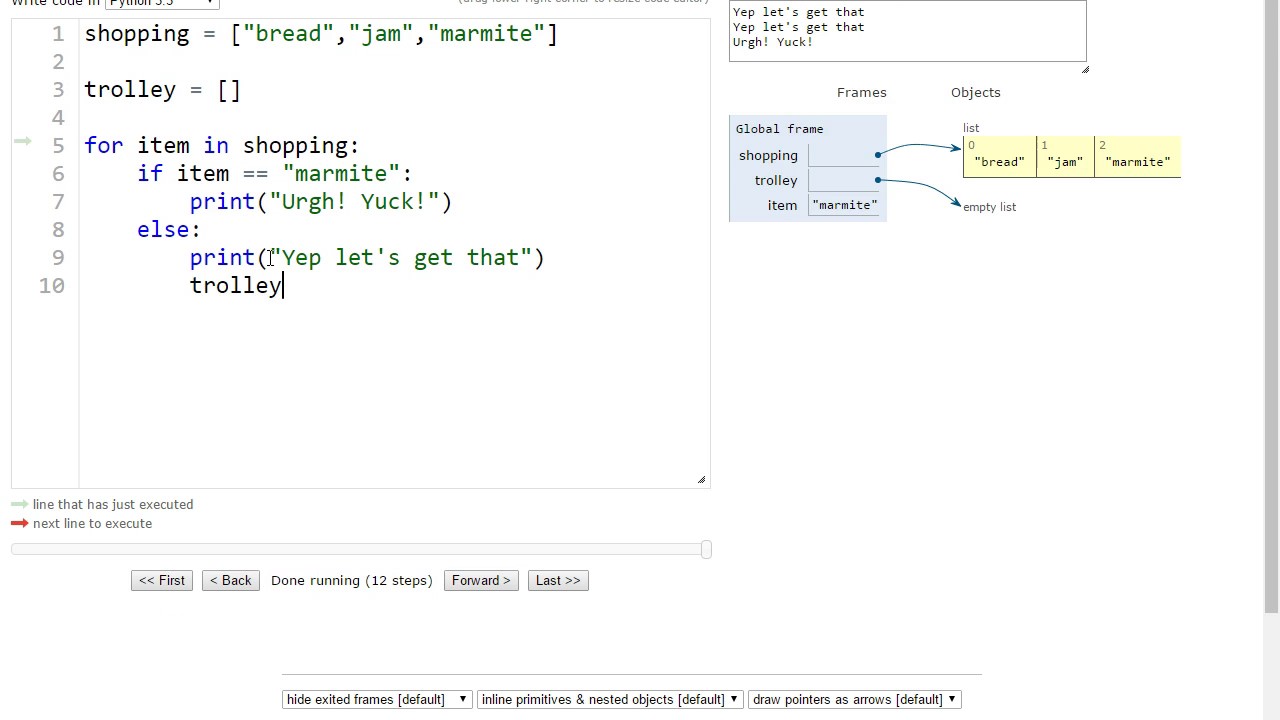
print(my_list) # Output: [1, 2, 3, 4, 5]
In this example, the list statement my_list = [1, 2, 3, 4, 5]
creates a new list object and assigns it to the variable my_list
. The values within the brackets are the initial elements of the list.
List statements can also include strings. Here's an example:
my_list = ['apple', 'banana', 'cherry']
print(my_list) # Output: ['apple', 'banana', 'cherry']
In this case, the list statement creates a new list containing three string elements: "apple", "banana", and "cherry".
List statements can also include nested lists. Here's an example:
my_list = [[1, 2], [3, 4], [5, 6]]
print(my_list) # Output: [[1, 2], [3, 4], [5, 6]]
In this case, the list statement creates a new list containing three nested lists. Each of these nested lists contains two elements.
List statements can also include dictionary items. Here's an example:
my_list = [{'name': 'John'}, {'age': 30}, {'city': 'New York'}]
print(my_list) # Output: [{'name': 'John'}, {'age': 30}, {'city': 'New York'}]
In this case, the list statement creates a new list containing three dictionary items. Each of these dictionary items contains a key-value pair.
List statements can also include None values. Here's an example:
my_list = [1, None, True, 2.5]
print(my_list) # Output: [1, None, True, 2.5]
In this case, the list statement creates a new list containing four elements, including one None value.
List statements can also include other data types such as tuples, sets, and dictionaries. Here's an example:
my_list = [(1, 2), {1, 2, 3}, {'name': 'John', 'age': 30}]
print(my_list) # Output: [(1, 2), {1, 2, 3}, {'name': 'John', 'age': 30}]
In this case, the list statement creates a new list containing three elements. The first element is a tuple, the second element is a set, and the third element is a dictionary.
In conclusion, list statements in Python are used to create and initialize lists of various types, including strings, integers, floats, tuples, sets, dictionaries, and None values. They can be used to store data for further processing or manipulation.