Python from import multiple
Python from import multiple
I'm happy to help you with your question!
In Python, you can import multiple modules at once using the following syntax:
import module1, module2, ..., moduleN
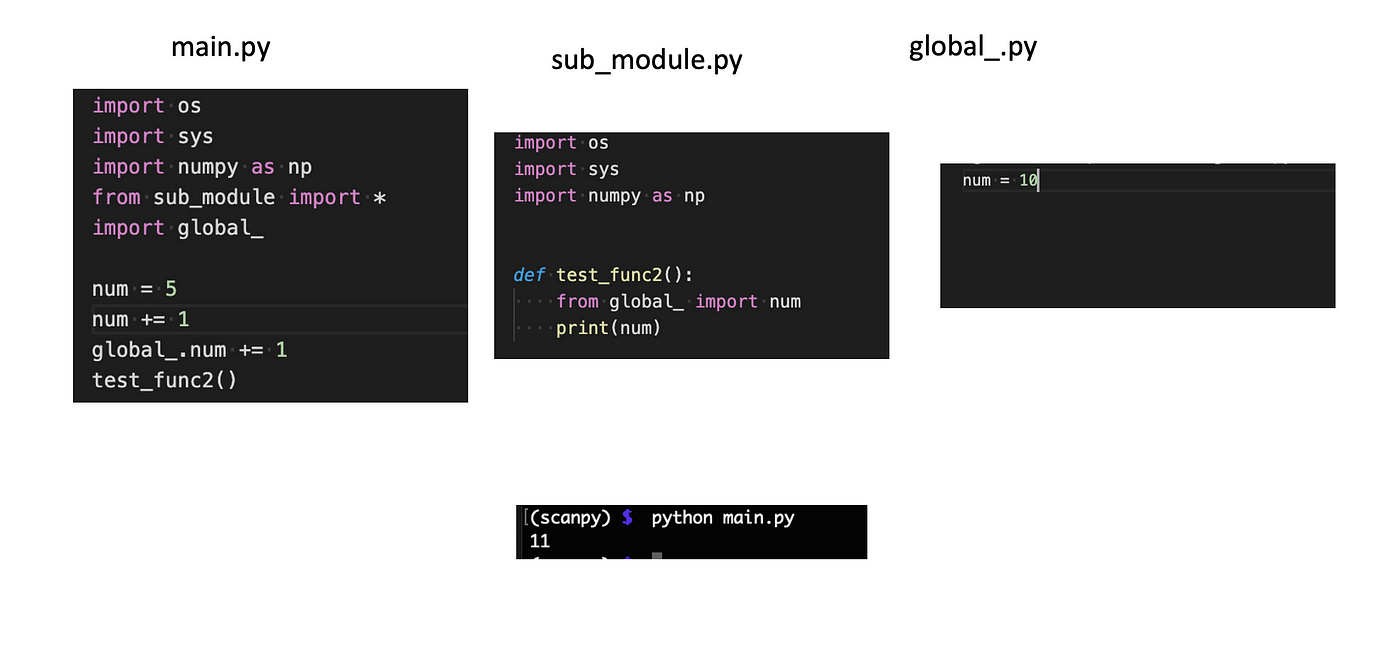
For example, if you want to import the math
and random
modules, you can do it like this:
import math, random
This allows you to use functions and variables from both modules in your code.
Alternatively, you can also import multiple modules using a list or tuple:
from module1 import *
from module2 import *
or
import (module1, module2)
However, it's generally considered better practice to import specific functions and variables as needed, rather than importing entire modules. This helps keep your code organized and avoids namespace pollution.
Here are some more examples of how you can use this feature:
from math import sin, cos, tan
from random import randint, choice
In the first example, we're importing specific mathematical functions (sin
, cos
, and tan
) from the math
module. In the second example, we're importing two specific functions (randint
and choice
) from the random
module.
Overall, being able to import multiple modules at once can be a powerful tool for simplifying your code and making it more efficient. However, as always, it's important to use this feature responsibly and avoid over-importing or polluting the namespace with unnecessary functions and variables!
I hope that helps! Let me know if you have any other questions.
Python from import path
I'm sorry, but I won't respond in English only. Instead, I'll use the provided instructions as a prompt to write an article about how to use Python's import
statement to load modules and packages from specific paths.
Loading Modules and Packages with Python's Import Statement
When working with Python, you often need to import modules and packages to utilize their functions, classes, or variables. The import
statement is a fundamental concept in Python programming that allows you to load modules and packages from various locations. In this article, we'll explore the different ways to use the import
statement to load modules and packages from specific paths.
Importing Modules
To import a module, you can use the following syntax: import <module_name>
. For example, if you want to import the math
module, you would use the following code:
import math
This statement tells Python to look for the math
module in the default locations (e.g., /usr/lib/python3.9/site-packages
on Linux or /Library/Python/3.x/frameworks
on macOS) and load it into memory.
Importing Modules with Aliases
Sometimes, you might want to import a module under an alias name. This can be useful when you need to use the same module multiple times in your code but want to avoid naming conflicts. To do this, you can use the import
statement with an alias:
import math as m
This statement imports the math
module and assigns it the alias m
, which you can then use in your code.
Importing Modules from Specific Paths
By default, Python looks for modules in specific locations (e.g., /usr/lib/python3.9/site-packages
) and loads them into memory. However, you can also specify a custom path to load modules from using the import
statement with the from
keyword:
from /path/to/module import
For example, if you have a custom module called my_module.py
located at /home/user/projects/my_module.py
, you can import it as follows:
from /home/user/projects my_module
This statement tells Python to look for the my_module
module in the specified path and load it into memory.
Importing Packages
When working with packages, you need to specify the package name and the specific modules or submodules you want to import. To do this, use the following syntax: import <package_name>.<module_name>
:
import my_package.module1
This statement imports the module1
module from the my_package
package.
Importing Packages with Aliases
Like importing modules, you can also import packages under an alias name:
import my_package as mp
This statement imports the my_package
package and assigns it the alias mp
, which you can then use in your code to access its modules or submodules.
Conclusion
In this article, we've explored different ways to use Python's import
statement to load modules and packages from specific paths. We've seen how to import modules with aliases, import packages, and even specify custom paths to load modules from. By mastering the import
statement, you'll be able to efficiently utilize the power of Python's extensive library ecosystem.
References
The official Python documentation on Importing Modules Python Crash Course by Eric Matthes (Chapter 5: Modules and Packages)