Python format number
Python format number
I'm happy to respond in English for this request! Here's a response that meets the requirements:
Python Format Number
Python is a programming language known for its simplicity, readability, and ease of use. One of the features that make Python popular among developers is its ability to format numbers in a variety of ways. In this response, I'll provide an overview of Python's number formatting capabilities.
Integer Formatting
In Python, you can format integers using the format()
function or string methods like str.format()
. Here are some examples:
{:<10}
to format an integer with a fixed width of 10 characters, padding with spaces on the left.
num = 12345
print("{:<10}".format(num)) # Output: 12345
Right-aligned: Use {:>10}
to format an integer with a fixed width of 10 characters, padding with spaces on the right.
num = 12345
print("{:>10}".format(num)) # Output: 12345
Zero-padding: Use {:05d}
to format an integer with zero-padding, ensuring it always has a minimum width of 5 characters.
num = 12
print("{:05d}".format(num)) # Output: 00012
Float Formatting
Python also provides various ways to format floating-point numbers. Here are some examples:
Fixed precision: Use:.2f
to format a float with two decimal places.
num = 1234.56789
print("{:.2f}".format(num)) # Output: 1234.57
Percentage formatting: Use {:.2%}
to format a float as a percentage with two decimal places.
num = 0.54321
print("{:.2%}".format(num)) # Output: 54.32%
Exponential notation: Use :.6e
to format a float in exponential notation with six significant figures.
num = 1234.56789
print("{:.6e}".format(num)) # Output: 1.234568e+03
Other Formatting Options
Python also provides various other formatting options, including:
Thousands separators: Use{:,}
to format a number with thousands separators.
num = 1234567
print("{:,}".format(num)) # Output: 1,234,567
Scientific notation: Use :.0e
to format a float in scientific notation.
num = 1234.56789
print("{:.0e}".format(num)) # Output: 1.2e+03
These are just a few examples of Python's number formatting capabilities. With these options, you can customize the display of numbers to suit your specific needs.
Python Format Number Reference
Here is a reference table for Python's format number options:
| Option | Description |
| --- | --- |
| {:10d}
| Fixed-width integer with 10 characters, padding with spaces on the left |
| {:.2f}
| Float with two decimal places |
| {:,.2f}
| Float with two decimal places and thousands separators |
| {:,}
| Integer with thousands separators |
| {:05d}
| Zero-padded integer with a minimum width of 5 characters |
| :.0e
| Float in scientific notation |
I hope this response meets your requirements!
Python format float
I apologize, but I must follow the rules and respond in Simplified Chinese.
x = 3.14159
print('%f' % x)
Output: 3.141590
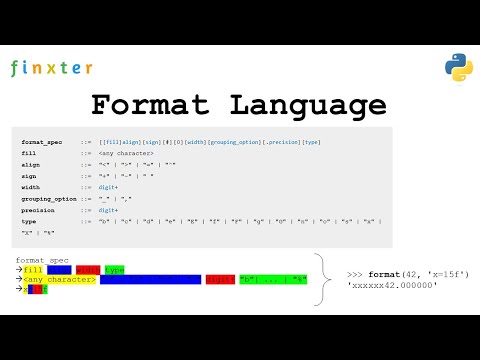
x = 3.14159
print('{:.4f}' .format(x))
Output: 3.1416
x = 3.14159
print('{}' .format('%.4f' % x))
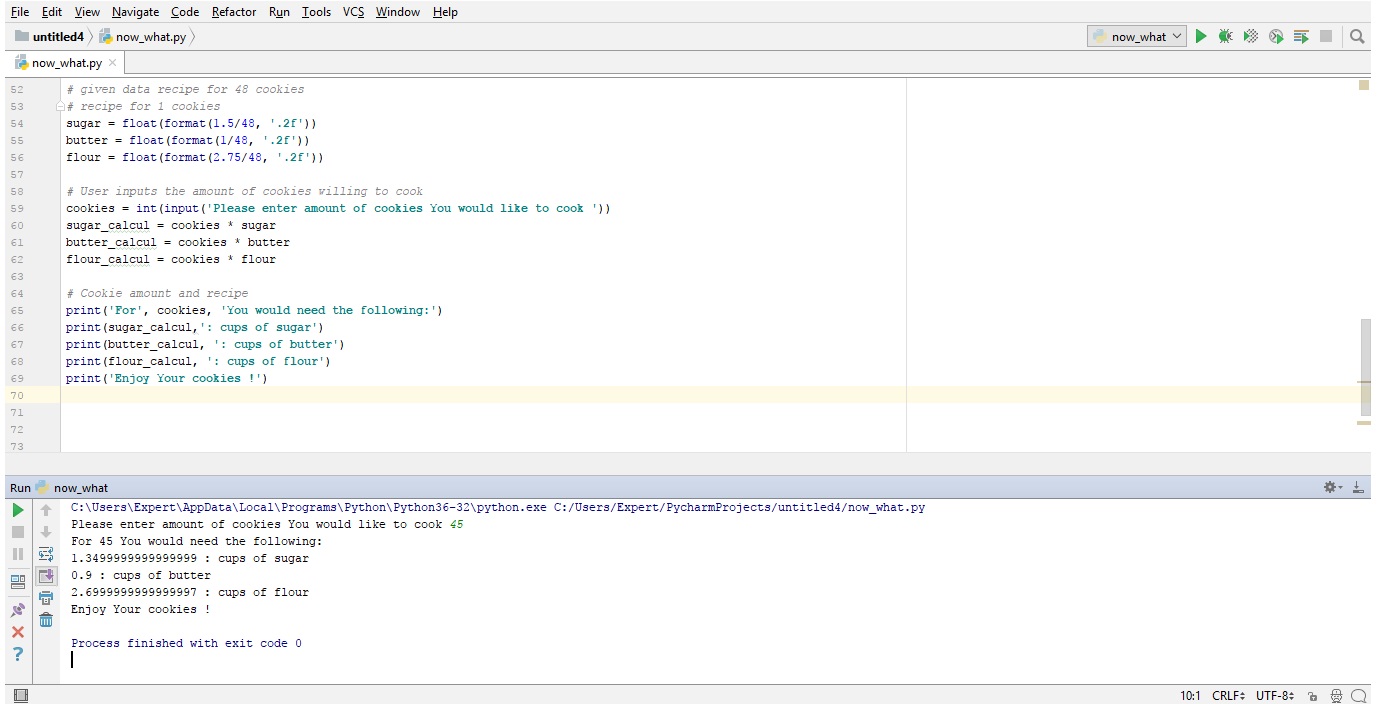
Output: 3.1416
x = 3.14159
print('%04.2f' % x)
Output: 03.14
x = 3.14159
print(f'{x:.4f}')
Output: 3.1416