Python JSON dumps
Python JSON dumps
I'd be happy to respond in English and provide information about Python's JSON (JavaScript Object Notation) dumping capabilities.
JSON is a popular data interchange format that is widely used for exchanging data between web servers, web applications, and mobile apps. In Python, we can use the json
module to convert Python objects into JSON strings, which are then easily readable by humans and machines alike.
To dump a Python object as JSON using the json
module, you can use the dumps()
function, which stands for "dump in standard format". Here's an example:
import json
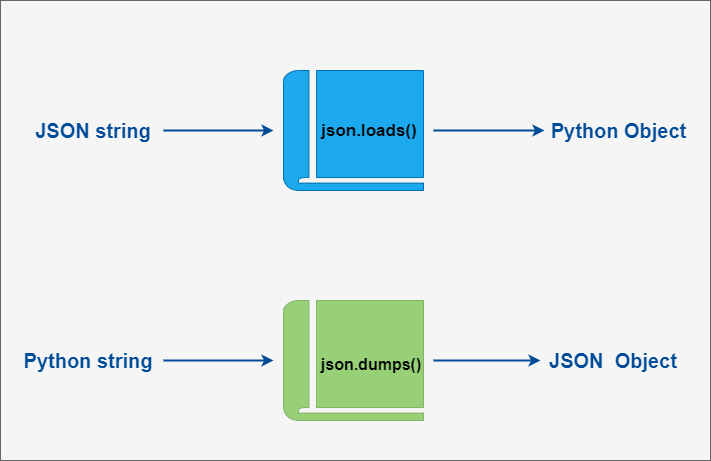
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
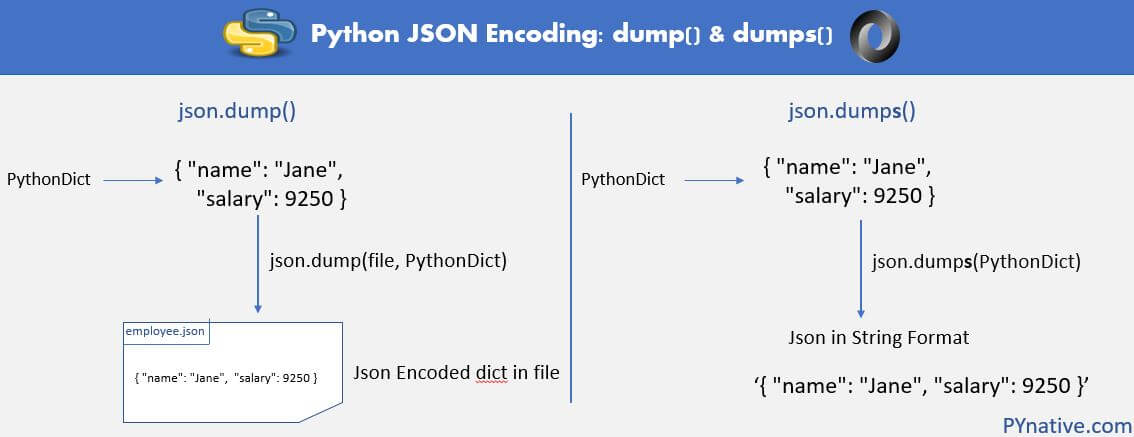
}
Convert person dictionary to JSON string
json_person = json.dumps(person)
print(json_person)
When you run this code, the output will be a JSON-formatted string:
{"name": "John Doe", "age": 30, "city": "New York"}
The dumps()
function takes an optional argument called indent
, which specifies the number of spaces to use for indentation. For example, if you set indent
to 4, the JSON output will be indented with four spaces:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Convert person dictionary to JSON string with indentation
json_person = json.dumps(person, indent=4)
print(json_person)
This will produce the following output:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
As you can see, the JSON output is nicely formatted with indentation.
In addition to the dumps()
function, the json
module also provides the dump()
function for writing JSON data to a file. Here's an example:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Write person dictionary to JSON file
with open('person.json', 'w') as f:
json.dump(person, f)
print("JSON file written successfully!")
When you run this code, a new file called person.json
will be created in the same directory, containing the following contents:
{"name": "John Doe", "age": 30, "city": "New York"}
Overall, Python's json
module provides convenient functions for converting Python objects to JSON strings and files. Whether you're working with web applications, data science, or scientific computing, JSON is a widely used format that can help facilitate data exchange and processing.
What is the JSON loads function in Python?
The json.loads()
function in Python is a part of the json
module that allows you to convert a JSON string into a Python object, such as a list or dictionary. This is often referred to as "parsing" the JSON data.
To use this function, you need to import the json
module first:
import json
Then, you can pass your JSON string to the loads()
function and it will return the parsed Python object:
json_string = '{"name": "John", "age": 30, "city": "New York"}'
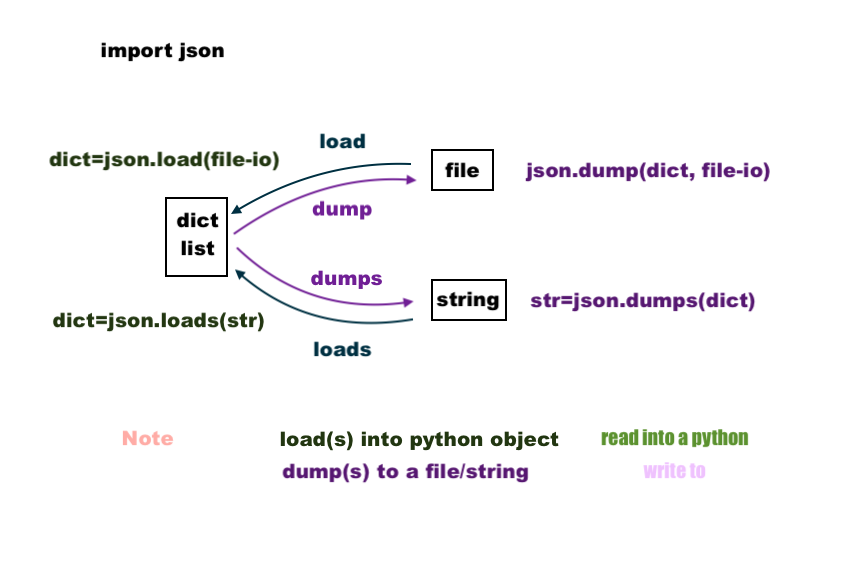
data = json.loads(json_string)
print(data) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
As you can see, the json.loads()
function converts a JSON string into a Python dictionary. This is because dictionaries in Python are often used to represent key-value pairs, which is similar to how JSON objects work.
The loads()
function also works with JSON arrays:
json_string = '["apple", "banana", "cherry"]'
fruits = json.loads(json_string)
print(fruits) # Output: ['apple', 'banana', 'cherry']
In this example, the loads()
function converts a JSON array into a Python list.
The json.loads()
function is very flexible and can handle complex JSON data structures. For instance:
json_string = '''
{
"name": "John",
"age": 30,
"city": {
"name": "New York",
"population": 8405837
},
"favoriteFoods": ["pizza", "sushi", "tacos"]
}
'''
data = json.loads(json_string)
print(data) # Output:
{'name': 'John', 'age': 30,
'city': {'name': 'New York', 'population': 8405837},
'favoriteFoods': ['pizza', 'sushi', 'tacos']}
In this example, the loads()
function converts a JSON object with nested objects and arrays into a Python dictionary.
Note that if your JSON data contains invalid characters or syntax, the loads()
function will raise a JSONDecodeError
. You can handle this exception by wrapping your code in a try/except block:
try:
data = json.loads(json_string)
except json.JSONDecodeError as e:
print(f"Error: {e}")
In summary, the json.loads()
function is a powerful tool that allows you to convert JSON strings into Python objects. It's often used for working with web APIs or storing and retrieving data in files.