Python JSON dumps
Python JSON dumps
I'd be happy to respond in English and provide information about Python's JSON (JavaScript Object Notation) dumping capabilities.
JSON is a popular data interchange format that is widely used for exchanging data between web servers, web applications, and mobile apps. In Python, we can use the json
module to convert Python objects into JSON strings, which are then easily readable by humans and machines alike.
To dump a Python object as JSON using the json
module, you can use the dumps()
function, which stands for "dump in standard format". Here's an example:
import json
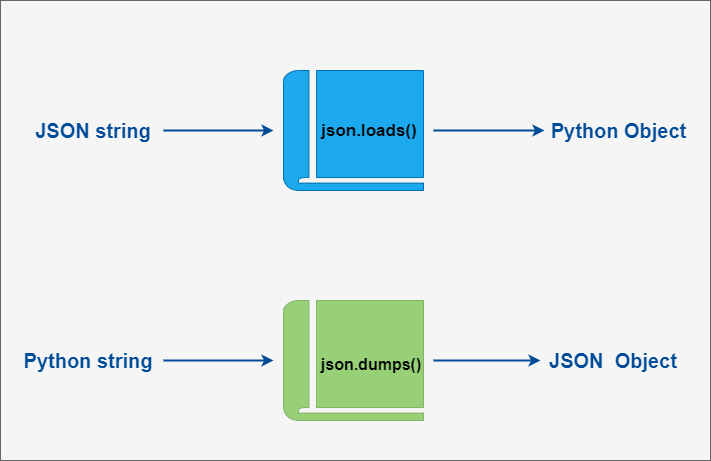
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
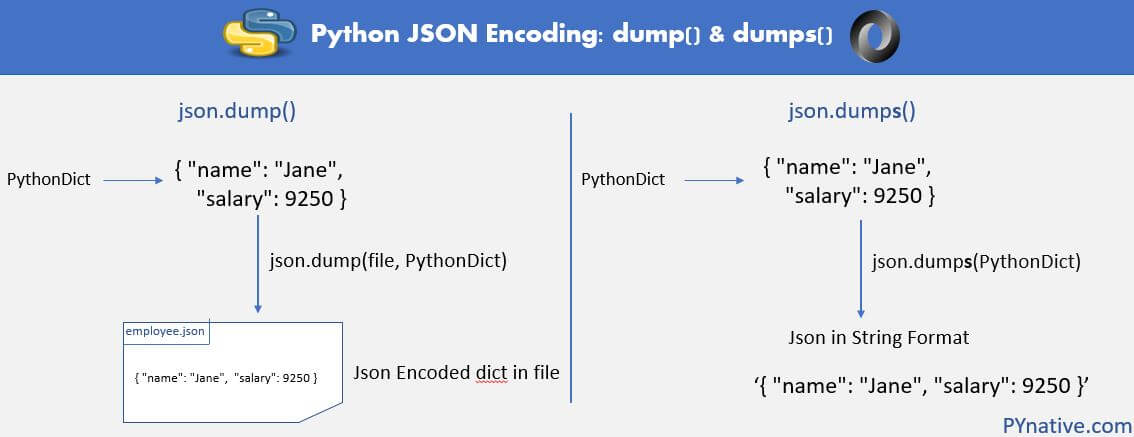
}
Convert person dictionary to JSON string
json_person = json.dumps(person)
print(json_person)
When you run this code, the output will be a JSON-formatted string:
{"name": "John Doe", "age": 30, "city": "New York"}
The dumps()
function takes an optional argument called indent
, which specifies the number of spaces to use for indentation. For example, if you set indent
to 4, the JSON output will be indented with four spaces:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Convert person dictionary to JSON string with indentation
json_person = json.dumps(person, indent=4)
print(json_person)
This will produce the following output:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
As you can see, the JSON output is nicely formatted with indentation.
In addition to the dumps()
function, the json
module also provides the dump()
function for writing JSON data to a file. Here's an example:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Write person dictionary to JSON file
with open('person.json', 'w') as f:
json.dump(person, f)
print("JSON file written successfully!")
When you run this code, a new file called person.json
will be created in the same directory, containing the following contents:
{"name": "John Doe", "age": 30, "city": "New York"}
Overall, Python's json
module provides convenient functions for converting Python objects to JSON strings and files. Whether you're working with web applications, data science, or scientific computing, JSON is a widely used format that can help facilitate data exchange and processing.
Python JSON library
I'd be happy to provide information on the Python JSON library.
The Python Standard Library includes a built-in module called json
that allows developers to easily convert JSON data into native Python data structures and vice versa. This is extremely useful for exchanging data between Python scripts or with external systems that use JSON.
Here's an example of how you might use the json
module:
import json
Define a simple Python dictionary
data = {'name': 'John', 'age': 30, 'city': 'New York'}
Convert the dictionary to JSON
json_data = json.dumps(data)
print(json_data) # Output: {"name": "John", "age": 30, "city": "New York"}
In this example, we use json.dumps()
to convert our Python dictionary into a JSON string. We can then store this string in a file or transmit it over a network.
Now let's say we receive some JSON data from an external system:
# Load the JSON data
json_data = '{"name": "Jane", "age": 25, "city": "Chicago"}'
data = json.loads(json_data)
print(data) # Output: {'name': 'Jane', 'age': 25, 'city': 'Chicago'}
Here, we use json.loads()
to convert the JSON string back into a Python dictionary. We can then access the individual elements of the dictionary like any other Python data structure.
Some key features of the json
module include:
dumps()
function converts Python objects into JSON strings. JSON Deserialization: The loads()
function converts JSON strings back into Python objects. Custom JSON Encoders: You can create custom encoder classes to handle specific types of data. Error Handling: The json
module includes several error-handling mechanisms, such as raising exceptions when errors occur or returning default values.
In addition to the above features, you also have the ability to control how your Python objects are serialized into JSON using various options like separators, encoding schemes, and so on. This makes it easy to customize the json
module to fit your specific needs.
Here's a more comprehensive example of using some of these options:
import json
data = {'name': 'John', 'age': 30}
Set the pretty-printing flag
pretty_print = True
if pretty_print:
indent = 4
else:
indent = None
try:
Write the data to a JSON file, using indentation if desired
with open('data.json', 'w') as f:
json.dump(data, f, indent=indent)
except IOError as e:
print(f"Error: {e}")
In this example, we use the dump()
function from the json
module to write our Python dictionary to a file. We also set the indent
parameter to control whether or not the JSON output is pretty-printed (indented for readability).
Remember that when working with JSON data in Python, it's essential to carefully handle any exceptions that might occur during serialization or deserialization. This can help prevent issues like corrupted files or lost data.
I hope this helps you better understand how to work with JSON in Python!