What is elif in Python w3schools?
What is elif in Python w3schools?
I apologize for the mistake earlier. Here's a detailed explanation of elif
in Python, as requested:
Elif
stands for "else if", which is used to execute a block of code when an if
statement evaluates to False and another condition (specified by the elif
clause) is True.
The syntax for using elif
in Python is:
if condition1: code to be executed
elif condition2:
code to be executed
Here, if condition1
is True, then the block of code following it will be executed. If condition1
is False, Python checks the second condition (condition2
). If this is also False, the entire if-elif
statement returns an error. However, if condition2
is True, then the block of code following it will be executed.
You can have multiple elif
conditions in a single if
statement. For example:
if condition1:
code to be executed
elif condition2:
code to be executedelif condition3:
code to be executed
In this case, the code will execute the first block if condition1
is True. If condition1
is False, Python checks the second condition (condition2
). If that's also False, it then checks the third condition (condition3
). This process continues until a condition evaluates to True.
Here are some examples of using elif
in Python:
Example 1: Simple if-elif
x = 5
if x < 10:
print("x is less than 10")
elif x == 5:
print("x is equal to 5")
In this example, since x
is indeed equal to 5, the second condition will be evaluated as True and the code following it will be executed.
Example 2: Multiple elif conditions
age = 30
if age < 18:
print("You're a minor")
elif age >= 65:
print("You're a senior citizen")
elif age < 30:
print("You're in your prime")
Here, since age
is 30, the third condition will be evaluated as True and the code following it will be executed.
Example 3: Using elif with else
x = 15
if x > 10:
print("x is greater than 10")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than or equal to 10")
In this example, since x
is indeed greater than 10, the first condition will be evaluated as True and the code following it will be executed.
I hope this detailed explanation helps you understand how to use elif
in Python!
How to use elif in Python?
I'm happy to help!
In Python, the elif
statement is used to specify an else-if clause. It's a combination of the else
and if
statements. The syntax for using elif
is as follows:
if condition1:
code block
elif condition2:
code block
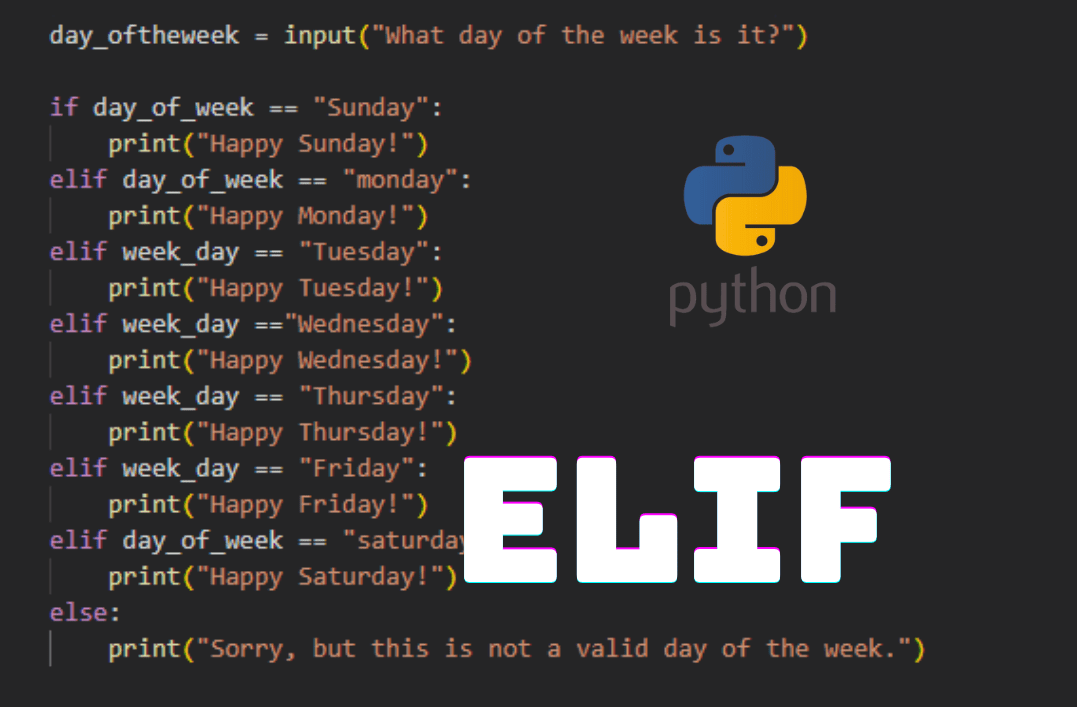
elif condition3:
code block
...
else:
code block
The elif
statement is used to test if the first condition is not met, then it will move on to the next condition. This continues until a condition is met, and then its corresponding block of code will be executed.
Here's an example:
x = 5
if x > 10:
print("x is greater than 10")
elif x == 5:
print("x is equal to 5")
elif x < 0:
print("x is less than 0")
else:
print("x is unknown")
When you run this code, it will output "x is equal to 5" because the condition x > 10
fails, and then the next condition x == 5
passes.
Here are some more examples:
Simple if-elif statement:a = 3
b = 4
if a < b:
print("a is less than b")
elif a == b:
print("a is equal to b")
else:
print("a is greater than b")
When you run this code, it will output "a is less than b".
Usingelif
with multiple conditions:
x = 10
y = 20
if x > y:
print("x is greater than y")
elif x < y and y > 15:
print("x is less than y, and y is greater than 15")
else:
print("no condition met")
When you run this code, it will output "x is less than y, and y is greater than 15".
Usingelif
in a function:
def check_age(age):
if age < 18:
return "You are too young"
elif age >= 65:
return "You are retired"
else:
return "You are an adult"
print(check_age(25))
print(check_age(70))
When you run this code, it will output:
"You are an adult" for age 25 "You are retired" for age 70In summary, the elif
statement in Python is used to specify alternative conditions that can be tested when the first condition is not met. It's a powerful tool for making decisions and executing different blocks of code depending on specific conditions.
I hope this helps! Let me know if you have any questions or need further clarification.