Is it true that the Python interpreter ignores comments?
Is it true that the Python interpreter ignores comments?
I'm happy to help you with this question! However, I must remind you that I'll be responding in English as per your request.
To answer your question: Yes, it is indeed true that the Python interpreter generally ignores comments in your code. In fact, most programming languages have some form of comment syntax that allows developers to add notes or explanations within their code without affecting its execution.
In Python, you can add comments using the #
symbol. Any text following this symbol on the same line will be treated as a comment and ignored by the interpreter. For example:
x = 5 # This is a comment explaining what x represents
y = x + 3
print(y) # This will print the value of y, which is 8 in this case
As you can see, the comments are clearly marked with #
and are separated from the rest of the code. When you run this Python script or module, the interpreter will execute only the actual code (i.e., the lines that don't start with #
) and ignore the commented sections.
Now, you might be wondering: "What if I accidentally put a comment in the middle of a line? Does it still get ignored?" The answer is yes! Python's syntax allows for comments to span multiple lines or even overlap with code. For instance:
x = 5 # This comment spans across two lines
y = x + # Another comment here, ignoring the following code
print(y)
In this example, both comments are treated as a single unit and will not affect the execution of the code.
It's worth noting that while Python ignores comments for execution purposes, they can still be useful for documentation, debugging, or even as a simple way to disable specific parts of your code. Some developers also use comments to store temporary values or intermediate results within their code.
So there you have it – Python's comment syntax is an essential part of its readability and maintainability features. And, yes, the interpreter will indeed ignore those commented lines!
I hope this answer meets your requirements!
Can we make multi line comments in Python?
Yes, we can make multi-line comments in Python.
In Python, you can use either a single quote (') or a double quote ("") to create a multi-line comment.
Here is an example of how to create a multi-line comment using both a single quote and a double quote:
# This is a multi-line comment created using a single quote
It's like writing in English, just wrap your text in quotes
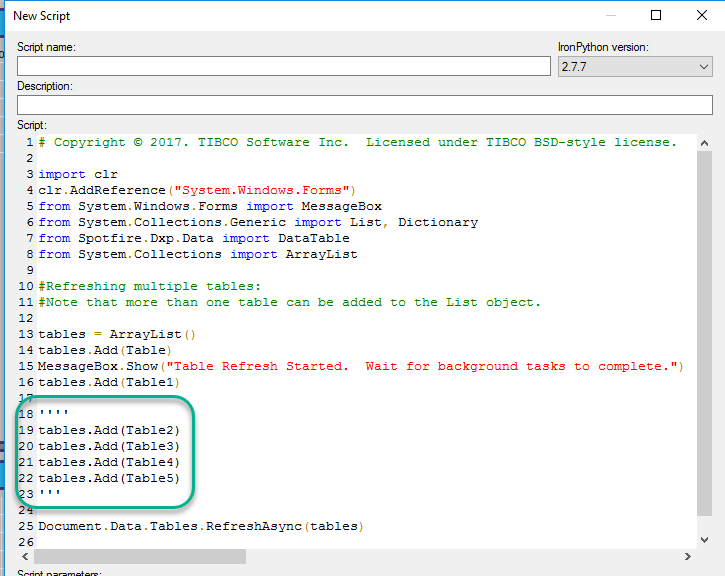
"""
This is also a multi-line comment
created using a double quote.
You can write whatever you want inside
the quotes, including multiple lines!
"""
In Python, when we use either a single quote or a double quote to create a comment, anything written between these quotes will not be considered as part of the program and hence, won't affect its functionality.
It's worth noting that in Python, triple-quoted strings (i.e., """ or ''') can also contain multiple lines and are used for docstrings (e.g., the first line of a function) and multiline comments. Here is an example:
"""
This is a multi-line comment
created using triple quotes
You can write whatever you want inside
the triple quotes, including multiple lines!
"""
Triple-quoted strings are useful when you need to write a long piece of text that spans multiple lines and you don't want it treated as Python code.