Lambda function in Python list
Lambda function in Python list
I'm glad you're interested in learning about lambda functions and their applications in Python!
A lambda function, also known as an anonymous function, is a small, single-purpose function that can be defined inline within a larger program. It's often used when you need to create a simple, one-time-use function without assigning it a name.
The basic syntax of a lambda function in Python is:
lambda arguments: expression
Here, arguments
are the input values passed to the lambda function, and expression
is the code that gets executed with those inputs.
Let's create a simple lambda function that takes two numbers as input and returns their sum:
sum_numbers = lambda x, y: x + y
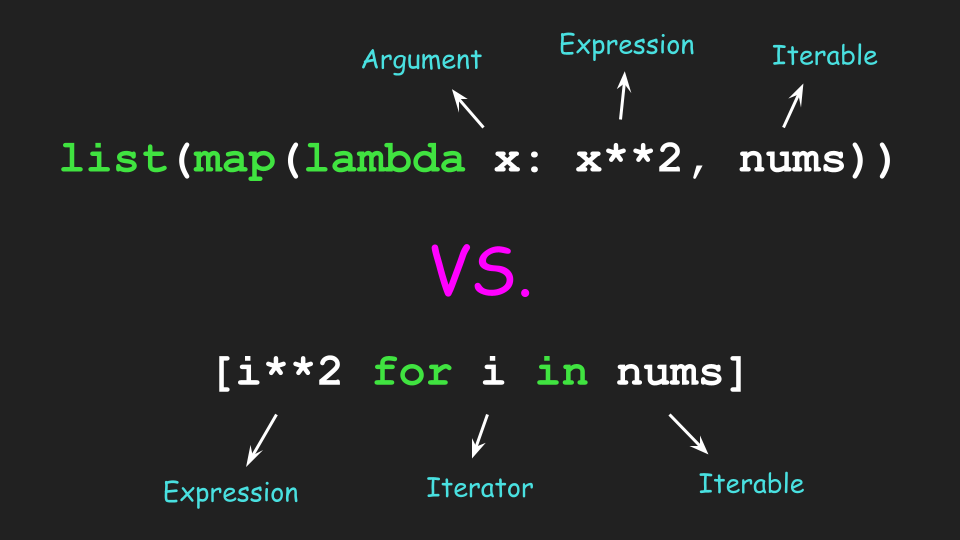
print(sum_numbers(3, 4)) # Output: 7
Now, let's see how we can use this lambda function in various contexts!
1. List Comprehension
A list comprehension is a concise way to create lists in Python. We can use a lambda function inside the list comprehension to transform each element:
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
2. Filtering
Lambda functions can also be used with the filter()
function to filter out elements based on a condition:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6]
3. Sorting
We can use a lambda function as the key
argument in the sort()
method to sort a list based on a custom criteria:
fruits = ['apple', 'banana', 'cherry']
sorted_fruits = sorted(fruits, key=lambda x: len(x))
print(sorted_fruits) # Output: ['apple', 'banana', 'cherry']
4. Higher-Order Functions
Lambda functions can also be passed as arguments to other higher-order functions (functions that take other functions as arguments):
numbers = [1, 2, 3, 4, 5]
double_numbers = list(map(lambda x: x * 2, numbers))
print(double_numbers) # Output: [2, 4, 6, 8, 10]
These examples demonstrate how lambda functions can be used in various contexts within Python programming. Lambda functions are particularly useful when you need a quick, one-time-use function without assigning it a name.
I hope this helps! Do you have any specific questions about using lambda functions or would you like me to elaborate on these topics?
Python anonymous functions list
Here are the details of Python anonymous functions:
What is an Anonymous Function?
In Python, an anonymous function (also known as a lambda function) is a small, single-purpose function that does not have a name. It's a shorthand way to create a simple function without declaring it with the def
keyword.
How to Create an Anonymous Function
To create an anonymous function, you use the lambda
keyword followed by the input parameters and the code block to be executed. The general syntax is:
anonymous_function = lambda arguments: expression
For example:
add_ten = lambda x: x + 10
print(add_ten(5)) # Output: 15
In this example, we created an anonymous function that takes a single argument x
and returns the result of adding 10 to it.
Common Use Cases
Anonymous functions are useful when you need to:
Define a simple, one-time-use function: When you only need to use a function once or twice in your code, an anonymous function can be a quick and easy solution. Pass a function as an argument: Anonymous functions are often used as arguments to other functions, such asmap()
, filter()
, or reduce()
. Create a small, ad-hoc transformation: For example, you might use an anonymous function to convert a list of strings to uppercase.
Examples
Here are some examples of using anonymous functions in Python:
# Example 1: Filtering out odd numbers
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
print(list(even_numbers)) # Output: [2, 4]
Example 2: Mapping a transformation to a list
fruits = ['apple', 'banana', 'cherry']
uppercase_fruits = map(lambda fruit: fruit.upper(), fruits)
print(list(uppercase_fruits)) # Output: ['APPLE', 'BANANA', 'CHERRY']
Example 3: Using an anonymous function as a reducer
numbers = [1, 2, 3, 4, 5]
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers) # Output: 15
Example 4: Creating a simple filter function
def is_even(x):
return lambda x: x % 2 == 0
even_numbers = filter(is_even(3), [1, 2, 3, 4, 5])
print(list(even_numbers)) # Output: [2, 4]
In this example, we used anonymous functions to:
Filter out odd numbers from a list Map a transformation (converting strings to uppercase) to a list Use an anonymous function as a reducer (calculating the sum of numbers) Create a simple filter functionI hope this helps! Let me know if you have any questions.