Do 2D arrays exist in Python?
Do 2D arrays exist in Python?
I'd be happy to help!
In Python, the answer is a bit nuanced. While you can't have exactly what is commonly referred to as a "true" 2D array in the sense that you would find it in languages like MATLAB or R, you can still achieve similar functionality using various data structures and libraries.
In Python, lists are one-dimensional arrays that can contain other lists, which gives the illusion of a 2D array. For instance:
my_2d_array = [[1, 2], [3, 4], [5, 6]]
This is often referred to as a "list of lists" or a "nested list." You can access individual elements by indexing into the outer list and then the inner list. For example:
print(my_2d_array[0][1]) # prints 2
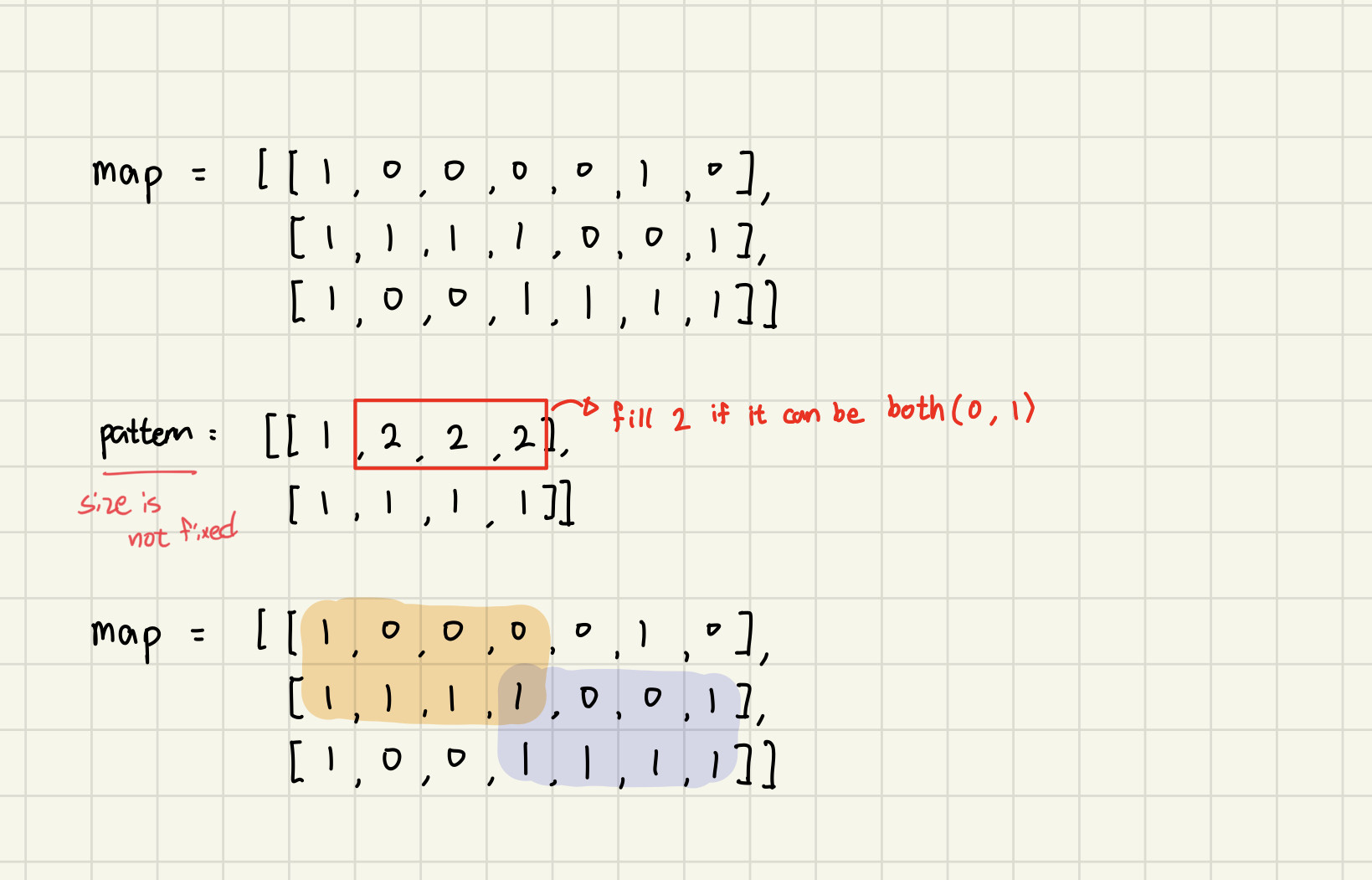
However, this approach has some limitations. For instance, you can't directly index into the inner lists using a single integer index like you would in a traditional 2D array.
If you need more advanced functionality or better performance for large datasets, you might consider using libraries like NumPy or Pandas. NumPy provides support for multidimensional arrays (including 2D ones) through its ndarray
class:
import numpy as np
my_2d_array = np.array([[1, 2], [3, 4], [5, 6]])
print(my_2d_array[0, 1]) # prints 2
Pandas offers similar capabilities through its DataFrame and Series classes. DataFrames are essentially 2D arrays with additional features for data manipulation and analysis.
In summary, while Python doesn't have a built-in "true" 2D array like some other languages, you can still achieve similar functionality using lists of lists or libraries like NumPy and Pandas.
Python array 2d multiple array
I'm happy to help! Here's the information you requested about Python arrays and specifically, 2D arrays with multiple arrays.
What is a Python Array?
In Python, an array is a data structure that stores multiple values of any type in a single variable. It is often used when dealing with collections of items that need to be processed together. The term "array" is sometimes used interchangeably with "list," but technically, a list is a type of array.
Creating 2D Arrays
A 2D array, also known as a matrix, is an array where each element is itself an array. You can think of it like a table with rows and columns, where each row represents an array of values.
In Python, you can create a 2D array using the []
operator to define the number of rows and columns:
array_2d = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This creates a 2D array with three rows (or arrays) and three columns. You can access an element in the array using its row and column indices, like this:
print(array_2d[0][1]) # Output: 2
Indexing and Slicing
When accessing or modifying elements in a 2D array, you need to specify both the row and column indices. For example:
array_2d[0]
accesses the first row (or array) as a whole. array_2d[1][1]
accesses the element at the second row and second column.
You can also slice a 2D array to extract a portion of it:
print(array_2d[:2, :]) # Output: [[1, 2, 3], [4, 5, 6]]
This slices the first two rows (or arrays) and all columns.
Multidimensional Arrays
Python allows you to create multidimensional arrays, where each element is itself an array. For example:
array_3d = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]
This creates a 3D array with two rows (or arrays), and two columns. Each of these elements is itself an array with two values.
Advantages of Using Arrays
Using Python arrays offers several advantages:
Efficient memory usage: Arrays store data in contiguous blocks of memory, making them more efficient than other data structures. Faster access times: Since arrays are stored contiguously, accessing an element is faster than searching through a list or dictionary. Easy to manipulate: You can use various methods (such asappend()
, extend()
, and sort()
) to modify the contents of an array.
I hope this helps! Do you have any specific questions about creating 2D arrays with multiple arrays in Python?