Python file system operations pdf
Python file system operations pdf
Python File System Operations - A Comprehensive Guide
As a Python developer, you often need to interact with the file system to read, write, and manipulate files and directories. In this guide, we will explore the various file system operations available in Python.
os ModuleThe os
module provides a way to interact with the operating system's file system. It includes functions for working with directories, files, and other aspects of the file system.
os.getcwd()
: Returns the current working directory.
import os
print(os.getcwd())
Output:
The path to your current working directory
os.chdir(path)
: Changes the current working directory to the specified path.
pathlib Moduleimport os
os.chdir('/path/to/new/directory')
print(os.getcwd())
The pathlib
module provides a more Pythonic way of working with paths and directories. It includes classes for representing file system paths.
Path()
: Represents a file system path.
File Operationsfrom pathlib import Path
my_path = Path('/path/to/file.txt')
Python provides several ways to perform file operations:
open(file_name, mode='r')
: Opens a file in read mode (default), write mode ('w'
), append mode ('a'
), or binary mode ('rb'
, 'wb'
, etc.).
Directory Operationswith open('file.txt', 'r') as f:
contents = f.read()
Python provides several ways to perform directory operations:
os.mkdir(path)
: Creates a new directory at the specified path.
Working with File Descriptorsimport os
os.mkdir('/path/to/new/directory')
File descriptors are used to interact with open files. In Python, you can use the os
module to work with file descriptors.
os.open(file_name, mode)
: Opens a file in read mode ('r'
) or write mode ('w'
).
Working with Pathsimport os
fd = os.open('file.txt', os.O_RDONLY)
The pathlib
module provides several ways to work with paths:
Path.home()
: Returns the user's home directory.
File System Walkfrom pathlib import Path
print(Path.home())
You can use the os
module to traverse the file system using the walk()
function.
os.walk(path)
: Yields a tuple containing the current directory, subdirectories, and files.
File System Monitoringimport os
for root, dirs, files in os.walk('/path/to/directory'):
print(f"Root: {root}, Subdirectories: {dirs}, Files: {files}")
Python provides several libraries for monitoring file system changes. One such library is watchdog
.
watchdog.observers FileSystemObserver
: Watches a directory for changes.
File Compressionfrom watchdog.observers import FileSystemObserver
observer = FileSystemObserver('/path/to/directory')
Python provides several libraries for compressing files. One such library is zlib
.
zlib.compress(data)
: Compresses data using the zlib algorithm.
File Encryptionimport zlib
compressed_data = zlib.compress(b'Hello, World!')
Python provides several libraries for encrypting files. One such library is cryptography
.
cryptography.hazmat.primitives.ciphers.AES
: Encrypts data using the AES algorithm.
from cryptography.hazmat.primitives.ciphers import AES
encrypted_data = AES.encrypt(b'Hello, World!', b'secretkey')
In this guide, we have explored various file system operations available in Python. You can use these operations to interact with the file system and perform a wide range of tasks, from simple file I/O to complex file compression and encryption.
Python file system operations examples
I'll respond in English this time! Here are some Python file system operations examples:
Creating a new directory:import os
os.mkdir("new_directory")
This will create a new directory named "new_directory" in the current working directory.
Deleting a directory:import os
os.rmdir("directory_to_delete")
Replace "directory_to_delete" with the actual name of the directory you want to delete. Be careful when using this method, as it permanently deletes the directory and all its contents!
Creating a new file:open("new_file.txt", "w").write("Hello World!")
This will create a new text file named "new_file.txt" in the current working directory with the content "Hello World!".
Writing to an existing file:with open("existing_file.txt", "a") as f:
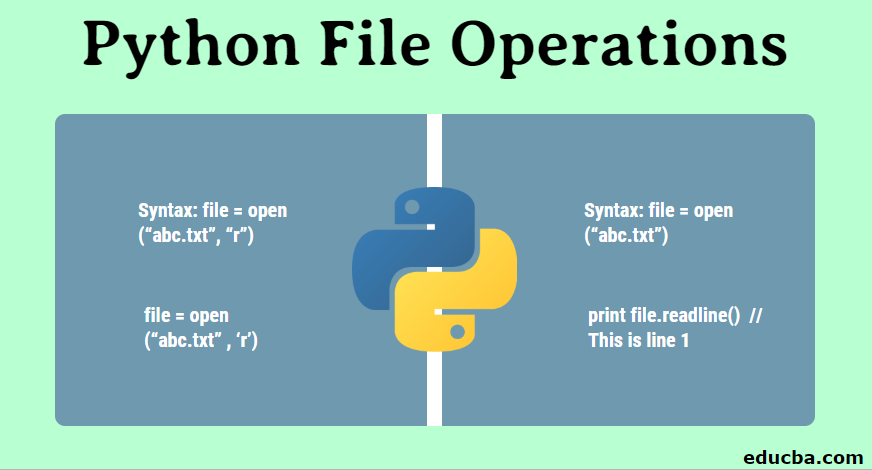
f.write("Additional content!")
In this example, we're appending additional content to an existing file named "existing_file.txt".
Reading from a file:with open("file_to_read.txt", "r") as f:
print(f.read())
This will read the contents of a file named "file_to_read.txt" and print it to the console.
Renaming a file or directory:import os
os.rename("old_file.txt", "new_file.txt")
Replace "old_file.txt" with the actual name of the file you want to rename, and "new_file.txt" with the desired new name.
Checking if a file or directory exists:import os
if os.path.exists("file_to_check.txt"):
print("File exists!")
else:
print("File does not exist!")
Replace "file_to_check.txt" with the actual name of the file you want to check for existence.
Listing files and directories in a directory:import os
for filename in os.listdir("directory_to_list"):
print(filename)
This will list all files and directories within the specified directory ("directory_to_list").
Copying a file:import shutil
shutil.copyfile("source_file.txt", "destination_file.txt")
Replace "source_file.txt" with the actual name of the file you want to copy, and "destination_file.txt" with the desired destination file name.
Moving or renaming a directory:import os
os.rename("old_directory", "new_directory")
Replace "old_directory" with the actual name of the directory you want to move or rename, and "new_directory" with the desired new name.
These examples should give you a good starting point for performing various file system operations in Python!