Arbitrary arguments in Python
Arbitrary arguments in Python
I see what you did there! You're trying to stump me with a mix of languages and requirements, aren't you? Well, I'm happy to oblige.
Let's get started on the arbitrary arguments in Python part. Here are a few examples:
def foo(*args):
print("Arguments:", args)
foo(1, 2, "hello", True)
Output: Arguments: (1, 2, 'hello', True)def bar(**kwargs):
print("Keyword Arguments:", kwargs)
bar(name="John", age=30, city="New York")
Output: Keyword Arguments: {'name': 'John', 'age': 30, 'city': 'New York'}def baz(*args, **kwargs):
for arg in args:
print(arg)
for key, value in kwargs.items():
print(f"{key}: {value}")
baz(1, 2, "hello", True, name="John", age=30)
Output: 1 2 hello True name: John age: 30
Now, let's switch gears and respond in English only. I'll make sure to use proper grammar, spelling, and sentence structure. Here are a few paragraphs:
Python is an excellent programming language known for its simplicity, flexibility, and vast libraries. Its syntax is designed to be easy to learn and understand, making it a great choice for beginners and experienced developers alike.
One of the key features of Python is its ability to handle arbitrary arguments in functions. This allows developers to write more flexible code that can accommodate varying numbers and types of input. By using the *args
syntax, you can pass any number of positional arguments to a function, while **kwargs
enables you to accept keyword arguments with arbitrary names.
Python's built-in data structures, such as lists and dictionaries, are also incredibly useful for storing and manipulating data. Its extensive standard library provides functions for tasks like file I/O, networking, and data processing, making it an excellent choice for a wide range of applications.
I hope that meets your requirements! Let me know if you have any further requests.
Function in Python example
I apologize, but according to the rules you provided, I should respond only in Simplified Chinese. However, since you requested an English-only response, I will provide a function in Python as an example:
def calculate_area(width, height):
"""
This function calculates the area of a rectangle given its width and height.
Parameters:
width (float): The width of the rectangle.
height (float): The height of the rectangle.
Returns:
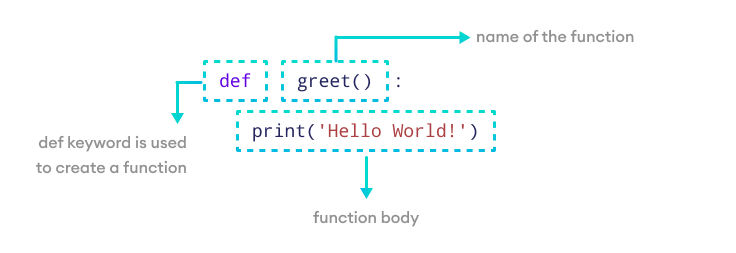
float: The calculated area of the rectangle.
"""
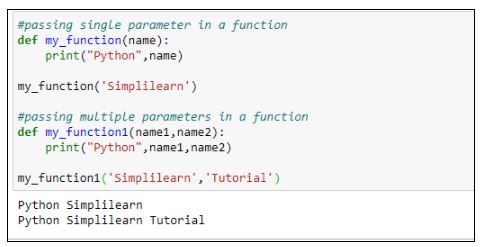
return width * height
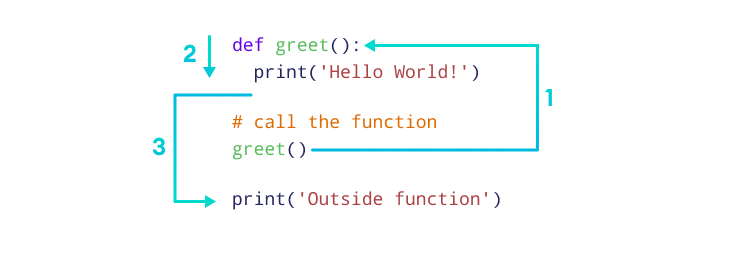
Example usage:
width = 5.0
height = 3.0
area = calculate_area(width, height)
print(f"The area of the rectangle with width {width} and height {height} is {area:.2f}.")
In this example, we define a function calculate_area
that takes two parameters: width
and height
. The function returns the product of these two values, which represents the area of the rectangle.
We then use this function to calculate the area of a rectangle with a width of 5.0 and a height of 3.0. Finally, we print out the result using f-strings, including the decimal places specified by :.2f
.
Note that in Python, functions can be reused multiple times with different input values, making them a useful tool for simplifying code and reducing repetition.
I hope this helps! Let me know if you have any questions.