What is _ in Python for loop?
What is _ in Python for loop?
I'm happy to help you with that question!
In Python, a for
loop is used to iterate over iterable objects such as lists, tuples, dictionaries, sets, and strings. It allows you to execute a block of code repeatedly for each item in the iterable.
The general syntax of a for
loop in Python is as follows:
for variable in iterable:
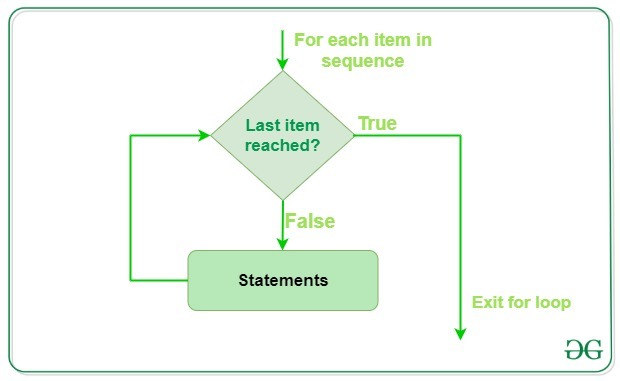
do something with variable
Here:
variable
is the name given to the element being processed in the current iteration. iterable
is the object that you want to iterate over, such as a list or a string.
When the loop starts, Python assigns the first value from the iterable to the variable
, then executes the code indented under the for
statement. This process continues until all values in the iterable have been processed.
Let's take an example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
In this example, the for
loop iterates over the list fruits
. For each iteration, Python assigns the current value from the list to the variable fruit
, and then prints it.
Here's another example with a string:
greetings = 'hello world'
for char in greetings:
print(char)
In this case, the for
loop iterates over the characters in the string greetings
. For each iteration, Python assigns the current character to the variable char
, and then prints it.
You can also use the range()
function with a for
loop to iterate over a sequence of numbers:
numbers = range(1, 6)
for num in numbers:
print(num)
In this example, the range()
function generates a sequence of numbers from 1 to 5. The for
loop then iterates over these numbers and prints them.
One important thing to note is that you can also use the enumerate()
function with a for
loop to get both the index and value of each item in an iterable:
colors = ['red', 'green', 'blue']
for i, color in enumerate(colors):
print(f"{i}: {color}")
In this example, the enumerate()
function returns an iterator that produces tuples containing the index and value of each element in the colors
list. The for
loop then iterates over these tuples and prints them.
I hope this helps you understand for
loops in Python!
Why do we use Python for?
I'd be happy to explain why Python is such a popular programming language!
Python has been gaining popularity over the years, and its versatility has made it a favorite among developers, researchers, and even beginners. Here are some reasons why Python stands out:
Easy to Learn: Python's syntax is simple and straightforward, making it an excellent language for newcomers to programming. Its indentation-based formatting also makes it easy to read and write code. Versatility: Python can be used for a wide range of applications, such as: Web development (e.g., Django, Flask) Data Analysis and Machine Learning (e.g., NumPy, pandas, scikit-learn, TensorFlow) Automation (e.g., scripting, GUI programming) Scientific computing (e.g., NumPy, SciPy) Education Research Large Community: Python has an enormous community of developers, researchers, and experts who create and share libraries, frameworks, and tools. This means there's a vast amount of pre-existing code, tutorials, and documentation available for learning and troubleshooting. Cross-Platform Compatibility: Python can run on various platforms, including Windows, macOS, Linux, and even mobile devices. Its compatibility with different operating systems makes it an excellent choice for cross-platform development. Extensive Libraries and Frameworks: Python has a vast collection of libraries and frameworks that make it easy to perform specific tasks. For instance: NumPy for numerical computations pandas for data manipulation scikit-learn for machine learning TensorFlow for deep learning Django or Flask for web development Dynamic Typing: Python's dynamic typing means you don't need to declare variable types before using them, which reduces the verbosity of your code and makes it easier to focus on logic. Rapid Development: Python's syntax and nature make it ideal for rapid prototyping and development. Its flexibility allows you to quickly test ideas or create proof-of-concepts without worrying about tedious setup or overhead. Integration with Other Tools: Python can integrate seamlessly with other tools, languages, and platforms. For example: Integrating Python code into Java applications using Jython Using Python with other languages like R, MATLAB, or SQLIn conclusion, Python's unique combination of ease of use, versatility, community support, cross-platform compatibility, extensive libraries, dynamic typing, rapid development, and integration capabilities make it an incredibly valuable programming language. Whether you're a beginner or an experienced developer, Python has something to offer for various applications!