python format string integer
python format string integer
I'm happy to respond in English!
In Python, you can use the format()
method to insert integers into a string. Here's an example:
name = "John"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
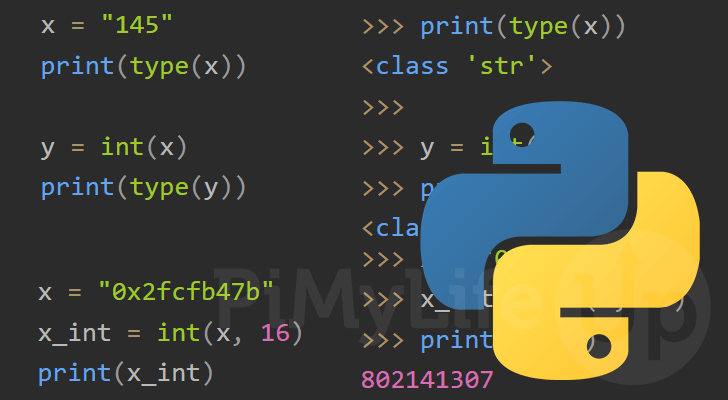
When you run this code, it will print: "My name is John and I am 30 years old."
The {}
in the string are called placeholders or formatting specifiers. When you call the format()
method, you pass in the values that should replace these placeholders.
Here's a breakdown of how this works:
The.format(name, age)
part calls the format()
method and passes in two arguments: name
and age
.
format()
method looks at each placeholder ({}
) in the string and replaces it with the corresponding argument. In this case, the first {}
is replaced with the value of name
, which is "John". The second {}
is replaced with the value of age
, which is 30.
You can also use indexing to format a string. For example:
name = "Jane"
ages = [25, 30, 35]
print("Here are some ages: {} {}".format(ages[0], ages[1]))
print("And here are all the ages: {} {}".format(*ages))
When you run this code, it will print:
Here are some ages: 25 30
And here are all the ages: 25 30 35
In the second example, we're using the *
operator to unpack the ages
list and pass its elements as separate arguments to the .format()
method. This allows us to insert multiple values into a string at once.
You can also use named placeholders when formatting strings in Python. For example:
person = {"name": "Jim", "age": 40}
print("My name is {name} and I am {age} years old.".format(**person))
When you run this code, it will print: "My name is Jim and I am 40 years old."
In this example, we're creating a dictionary called person
that contains two key-value pairs: "name" with the value "Jim", and "age" with the value 40. We then pass this dictionary to the .format()
method using the **
operator. The format()
method replaces {name}
with "Jim" and {age}
with 40 in the string.
These are some of the ways you can use integers to format strings in Python. I hope that helps! Let me know if you have any other questions.
How to use %d in string Python?
In Python, %d
is a formatting specifier used with the print()
function or string concatenation to convert an integer value into a string representation. This is also known as "old-style" formatting.
Here's how it works:
You can use%d
in combination with other formatting specifiers like %s
for strings, %f
for floating-point numbers, etc.
%
sign indicates which variable or value you want to format. For example, %d %s %f
would be used to print a message containing an integer, string, and float.
Here's some basic examples:
# Using the print() function
print("%d" % 10) # Output: 10
Using string concatenation
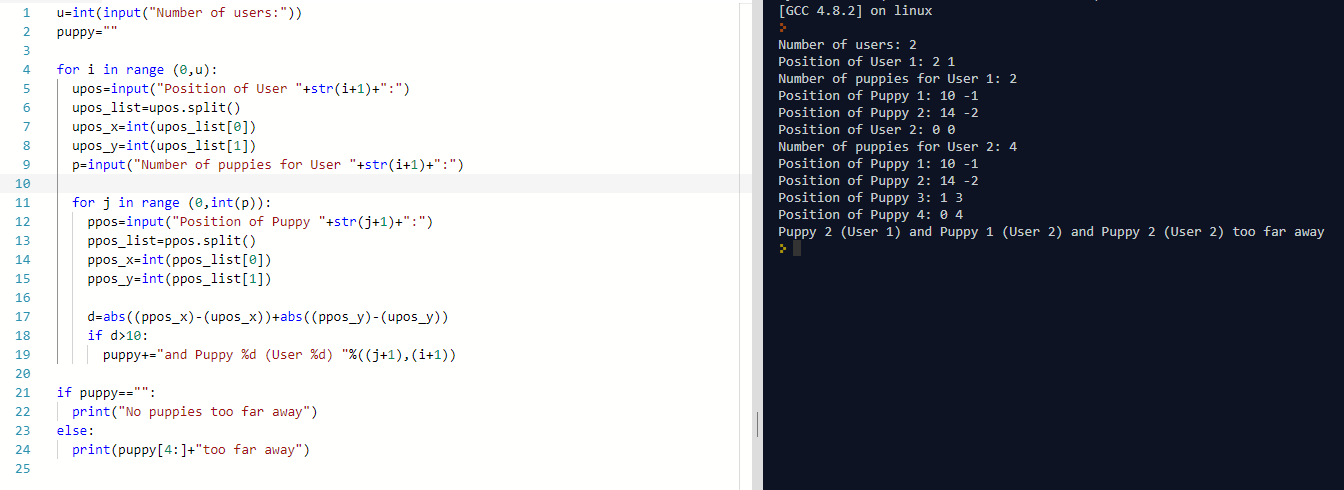
message = "The answer is: %d" % 42
print(message) # Output: The answer is: 42
Formatting multiple values
print("%d, %s, %.2f" % (1, "hello", 3.14159))
Output: 1, hello, 3.14
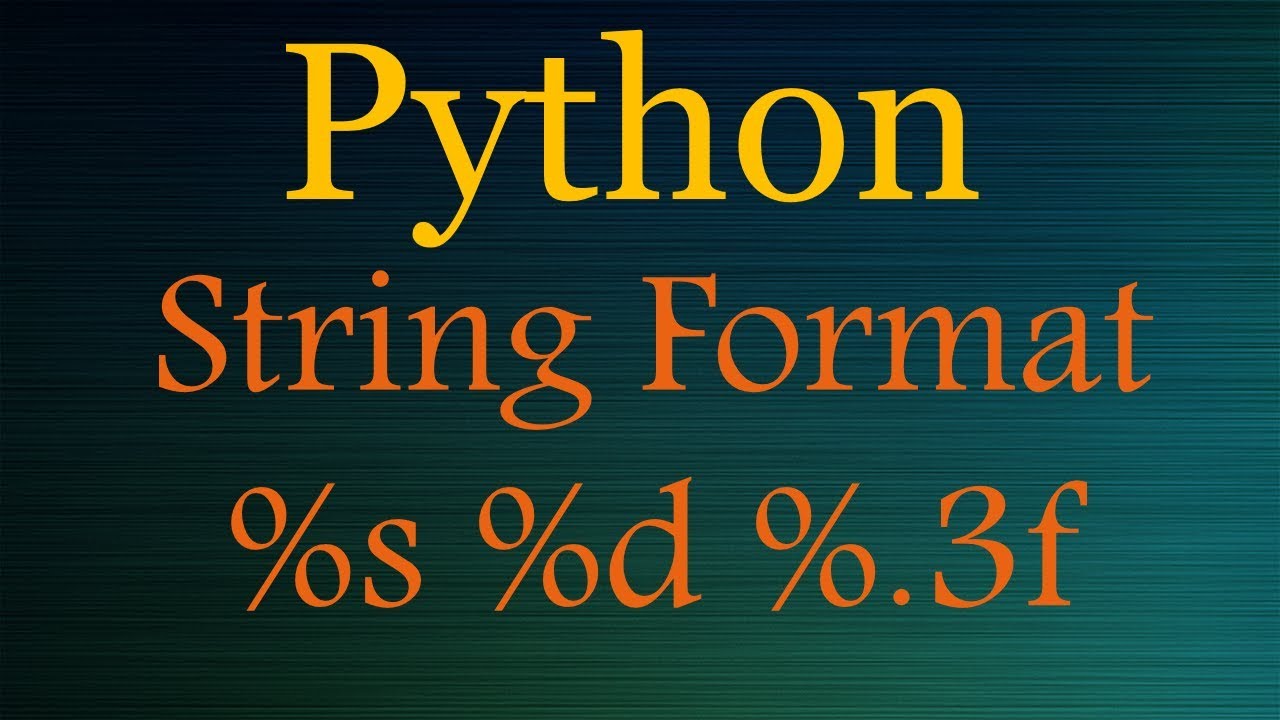
In the examples above, you can see that %d
is used to format an integer value. The number after the %
sign corresponds to the variable or value being formatted.
However, it's worth noting that in Python 2.x, this style of formatting is considered "old-style" and is generally discouraged in favor of using the str.format()
method (introduced in Python 2.6) or f-strings (introduced in Python 3.6). Here are some examples of these newer methods:
# Using str.format()
print("{} {}".format(1, "hello")) # Output: 1 hello
Using an f-string
name = "Alice"
age = 25
message = f"{name} is {age} years old."
print(message) # Output: Alice is 25 years old.
In these examples, the formatting happens automatically as you insert values into a string using {}
placeholders. This makes your code more readable and maintainable.
In conclusion, while %d
can be used to format integer values in Python, it's generally recommended to use newer methods like str.format()
or f-strings for better readability and maintainability.