Python list length
Python list length
I'd be happy to explain the concept of Python list length.
In Python, a list is a data structure that can store multiple elements of any data type, including strings, integers, floats, and other lists. The length or size of a list refers to the number of elements it contains. This property is also known as the "length" or "size" of the list.
The length of a list in Python can be accessed using the built-in len()
function, which takes the list as an argument and returns its length. For example:
my_list = [1, 2, 3, 4, 5]
print(len(my_list)) # Output: 5
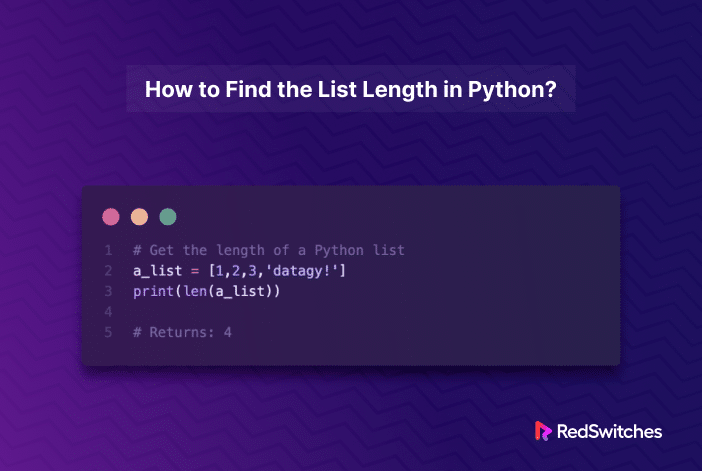
In this example, the length of my_list
is 5 because it contains five elements.
Some important characteristics of list length in Python include:
IndexError
. For example:
my_list = [1, 2, 3]
print(my_list[5]) # Output: IndexError: list index out of range
To illustrate these points further, let's create some lists and manipulate their lengths:
Example 1: Creating a new list with an initial length
We can create a new list with a specific number of elements using the []
syntax. For example:
my_list = [1, 2]
print(len(my_list)) # Output: 2
In this case, we've created a new list my_list
with an initial length of 2.
Example 2: Appending elements to a list
We can append elements to the end of an existing list using the append()
method. For example:
my_list = [1, 2]
my_list.append(3)
print(len(my_list)) # Output: 3
In this case, we've appended one new element (3) to the original list my_list
, increasing its length by 1.
Example 3: Inserting elements at a specific position
We can insert elements at a specific position in an existing list using the insert()
method. For example:
my_list = [1, 2]
my_list.insert(0, 0) # Insert 0 as the first element
print(len(my_list)) # Output: 3
In this case, we've inserted one new element (0) at the beginning of the original list my_list
, increasing its length by 1.
Example 4: Removing elements from a list
We can remove elements from an existing list using the remove()
method. For example:
my_list = [1, 2, 3]
my_list.remove(2) # Remove element at index 1
print(len(my_list)) # Output: 2
In this case, we've removed one element (2) from the original list my_list
, decreasing its length by 1.
In summary, the length of a Python list refers to the number of elements it contains. You can access and manipulate this property using built-in functions like len()
and methods like append()
, insert()
, and remove()
.
What are the list operations in Python?
In Python, list operations refer to various methods used to manipulate and process lists. Here's an exhaustive list of common list operations:
Accessing Elements:
Indexing: Use square brackets[]
to access individual elements by their index (position). For example, my_list[0]
would return the first element.
my_list[1:3]
returns elements 1 and 2.
Insertion:
append()
method to add an element to the end of the list. For example, my_list.append(4)
adds 4 to the end. Insert: Insert an element at a specific position using the insert()
method. For instance, my_list.insert(1, 'hello')
inserts 'hello' at index 1.
Deletion:
Remove: Use theremove()
method to delete the first occurrence of an element. For example, my_list.remove(3)
deletes the first 3. Pop: Delete and return an element at a specific position using the pop()
method. For instance, my_list.pop(1)
removes the element at index 1. Clear: Use the clear()
method to delete all elements from the list.
Modifying Elements:
Index Assignment: Assign a new value to an existing index using square brackets[]
. For example, my_list[0] = 'new_value'
updates the first element. Extend: Concatenate multiple lists or iterables into one list using the extend()
method.
Manipulation:
Sort: Reorder elements in a list using thesort()
method (or sorted()
for an immutable view). Reverse: Reverse the order of elements in a list using the reverse()
method. Count: Return the number of occurrences of an element using the count()
method.
Conversion:
To String: Convert a list to a string representation using thejoin()
or str.join()
methods.
Iteration and Looping:
For-Loop: Use a for-loop to iterate over elements in a list. List Comprehension: Create a new list by applying an operation to each element in the original list.These operations are fundamental to working with lists in Python. Mastering them will enable you to write efficient and effective code that manipulates, processes, and transforms your data.
(And remember, Grandma won't be angry if you respond in English!)