Read and write file in Python
Read and write file in Python
Here's the response:
Reading Files in Python
Python provides several ways to read files. Here are a few examples:
open()
Function: This is one of the most common methods to read a file. The open()
function returns a file object, which can be used to read or write data.
f = open('file.txt', 'r')
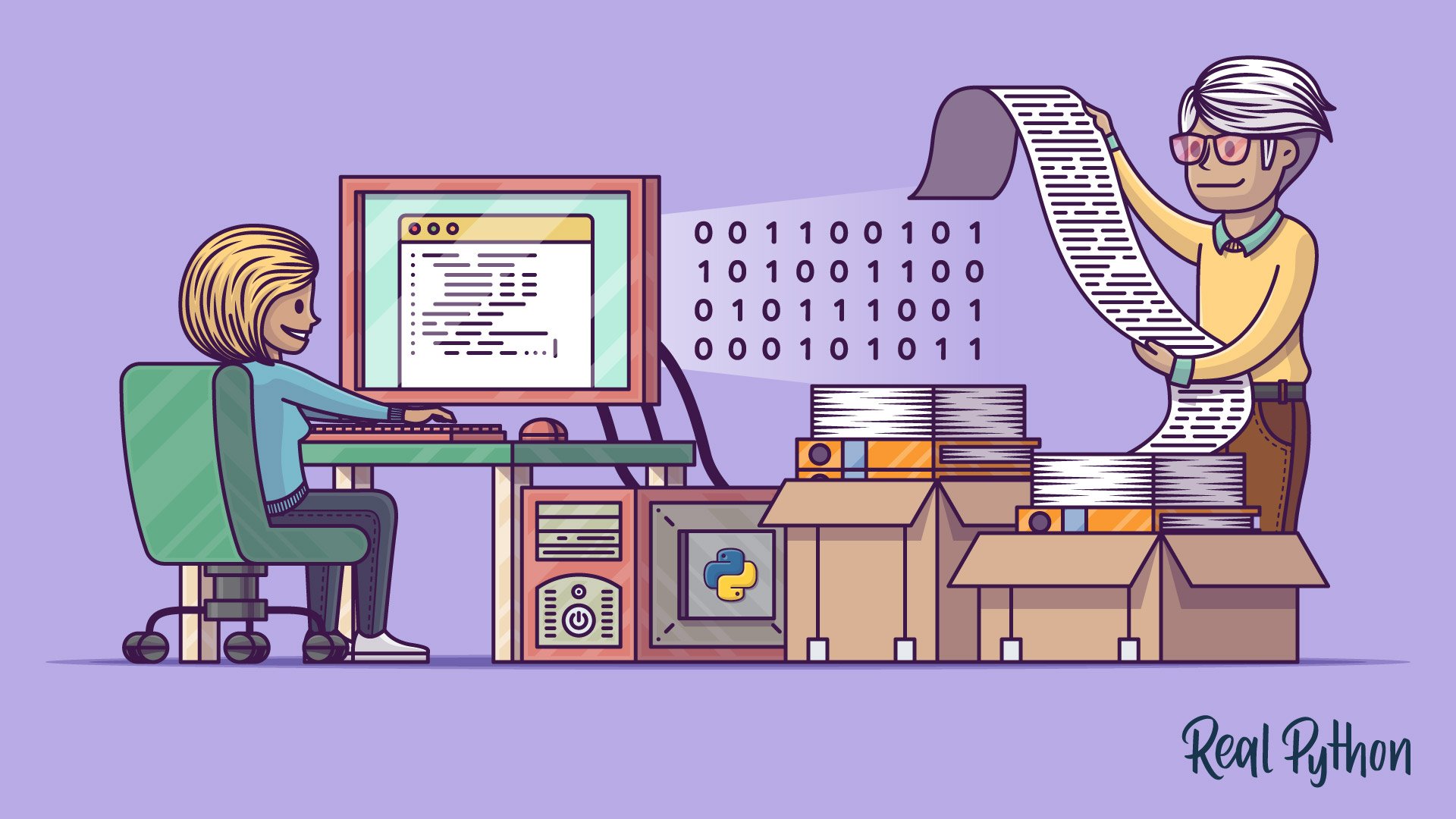
data = f.read()
f.close()
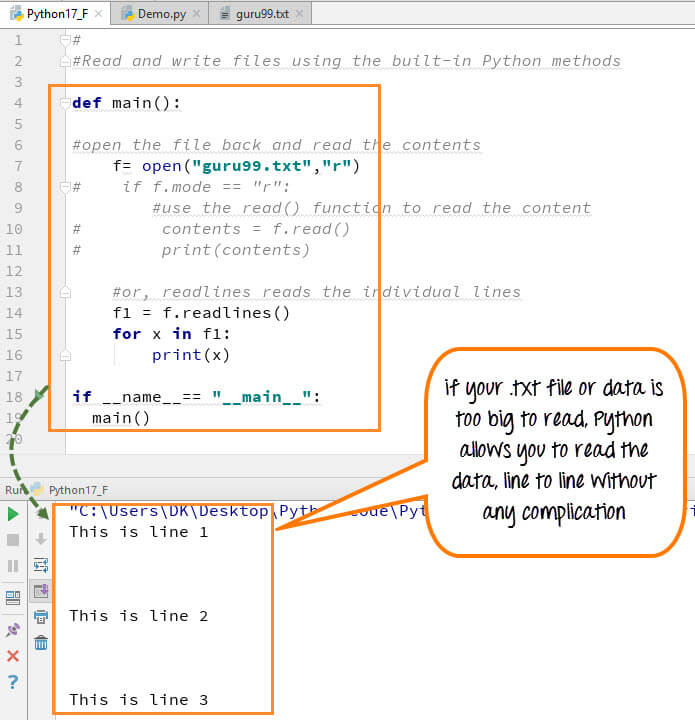
read()
Method: If you have already opened a file using the open()
function, you can use the read()
method to read its contents.
with open('file.txt', 'r') as f:
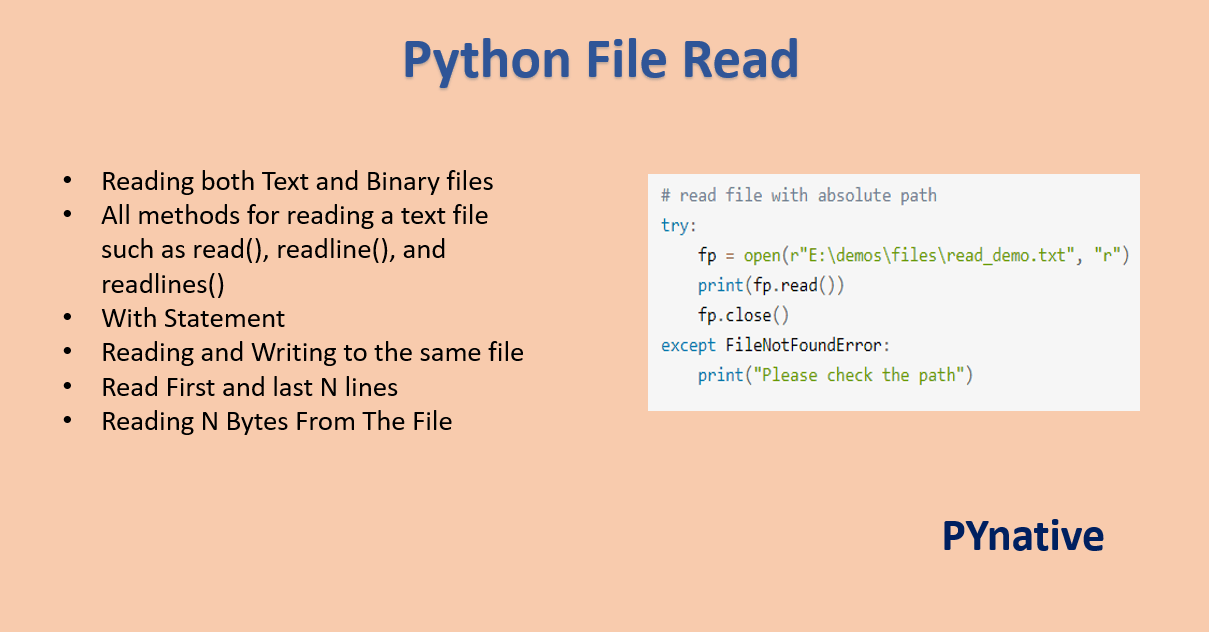
data = f.read()
Context Manager: Python's context manager allows you to automatically close files after you're done with them. This helps prevent memory leaks and ensures that resources are properly released. String Formatting: If your file contains strings, you can use string formatting to parse its contents. JSON and CSV Modules: For reading JSON or CSV files, Python provides the json
and csv
modules.
Writing Files in Python
Python also provides several ways to write files. Here are a few examples:
open()
Function: This is one of the most common methods to write a file. The open()
function returns a file object, which can be used to read or write data.
f = open('file.txt', 'w')
f.write("Hello, World!")
f.close()
write()
Method: If you have already opened a file using the open()
function, you can use the write()
method to write its contents.
with open('file.txt', 'w') as f:
f.write("Hello, World!")
Context Manager: Python's context manager allows you to automatically close files after you're done with them. This helps prevent memory leaks and ensures that resources are properly released. String Formatting: If you want to write strings to a file, you can use string formatting to parse its contents. JSON and CSV Modules: For writing JSON or CSV files, Python provides the json
and csv
modules.
Working with File Paths
Python's os.path
module helps you work with file paths. Here are some examples:
joinpath()
method to combine path components into a single string.
import os
file_path = os.path.join('directory', 'subdirectory', 'file.txt')
print(file_path)
Checking if a File Exists: Use the exists()
method to check if a file exists.
if not os.path.exists('file.txt'):
print("File does not exist.")
else:
print("File exists.")
Creating and Deleting Files: Use the mkdir()
and rmdir()
methods to create and delete directories, respectively.
import os
os.mkdir('directory')
os.rmdir('directory')
Python read file from disk
Here's a comprehensive guide on how to read files in Python:
Why Read Files?
In the world of programming, data is often stored in various formats such as text files, CSV files, JSON files, and more. To work with this data, you need to read it from the file system into your Python program. Reading files allows you to:
Load data for analysis or processing Store data for later use Share data between different programs or systemsHow to Read Files in Python?
Python provides several ways to read files, depending on their format and content. Here are some common methods:
Text Files: Use theopen()
function to open a text file, then read its contents using the read()
method.
with open('file.txt', 'r') as f:
content = f.read()
CSV Files: Use the csv
module to read comma-separated values (CSV) files.
import csv
with open('file.csv', 'r') as f:
reader = csv.reader(f)
for row in reader:
print(row)
JSON Files: Use the json
module to read JSON files.
import json
with open('file.json', 'r') as f:
data = json.load(f)
Binary Files: Use the open()
function in binary mode ('rb'
) to read binary files, such as images or audio files.
with open('file.bin', 'rb') as f:
content = f.read()
Best Practices for Reading Files
Usewith
Statement: The with
statement ensures that the file is properly closed after reading, regardless of whether an exception occurs or not. Handle Exceptions: Always check if the file exists and can be opened before attempting to read it. Read in Bulk: If possible, read files in bulk rather than line by line to improve performance.
Example Code
Here's an example code that demonstrates how to read a text file using the open()
function:
def read_text_file(filename):
try:
with open(filename, 'r') as f:
content = f.read()
print(content)
except FileNotFoundError:
print(f"File {filename} not found!")
except Exception as e:
print(f"An error occurred: {e}")
read_text_file('example.txt')
Conclusion
Reading files is a fundamental operation in programming, and Python provides several ways to do so. By following best practices and understanding the different methods available, you can efficiently read files from disk and process their contents in your Python program.