python context manager example
python context manager example
Here is an example of using a context manager in Python:
Let's say we have a file that we want to open and write some data to, but we also want to make sure that the file gets properly closed even if an error occurs.
Here is how you might do this without using a context manager:
try:
file = open('test.txt', 'w')
file.write('Hello World!')
except Exception as e:
print(f'An error occurred: {e}')
finally:
if 'file' in locals():
file.close()
This is a bit clumsy, and we have to make sure to close the file whether an exception occurs or not. This can be problematic if exceptions occur frequently, because it can cause unexpected behavior.
Using a context manager simplifies this process:
from io import StringIO
from contextlib import closing
with closing(StringIO()) as f:
try:
f.write('Hello World!')
except Exception as e:
print(f'An error occurred: {e}')
In this example, we use the contextlib
module's closing
function to create a new context manager that automatically closes its argument when it is exited. We then open our file in this new context using the StringIO()
class from the io
module.
When we enter the with
block, Python calls our context manager's __enter__
method to set up the context. This is where we would normally open a file or do some other setup work. When we exit the with
block, regardless of whether an exception occurred or not, Python calls our context manager's __exit__
method to tear down the context. In this case, this means that Python will automatically close the file for us.
This makes our code cleaner and easier to read, because it avoids having a big block of error handling code at the end of our program.
Here is another example where we are using context manager with a file:
from contextlib import contextmanager
@contextmanager
def open_file(filename):
try:
file = open(filename, 'r')
yield file
finally:
if 'file' in locals():
file.close()
with open_file('test.txt') as f:
data = f.read()
In this case, we are using a context manager to open and close a file. This is useful if you want to make sure that your file gets closed regardless of whether an exception occurs or not.
Remember that yield
keyword in Python means "this function can be used as a generator".
python context manager class
Here's a detailed explanation of how to create a context manager class in Python:
In Python, context managers are used to ensure that resources such as files, connections, and other objects are properly cleaned up after use. A context manager is an object that defines the runtime context for a block of code.
To create a context manager class, you need to define the __enter__
and __exit__
methods in your class. These two methods work together to provide the setup and teardown logic for the context manager.
Here's a basic example of how to create a simple context manager class:
class FileContextManager:
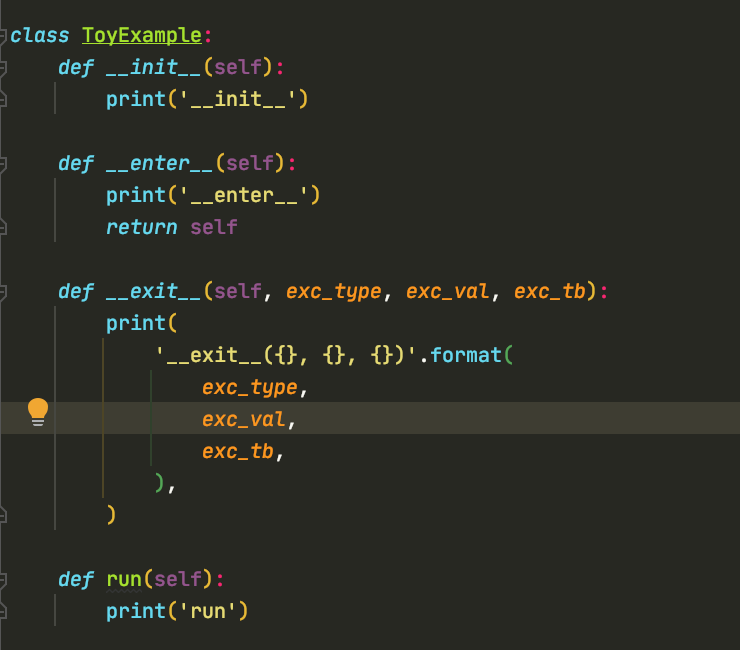
def init(self, filename):
self.filename = filename
self.file_handle = None
def enter(self):
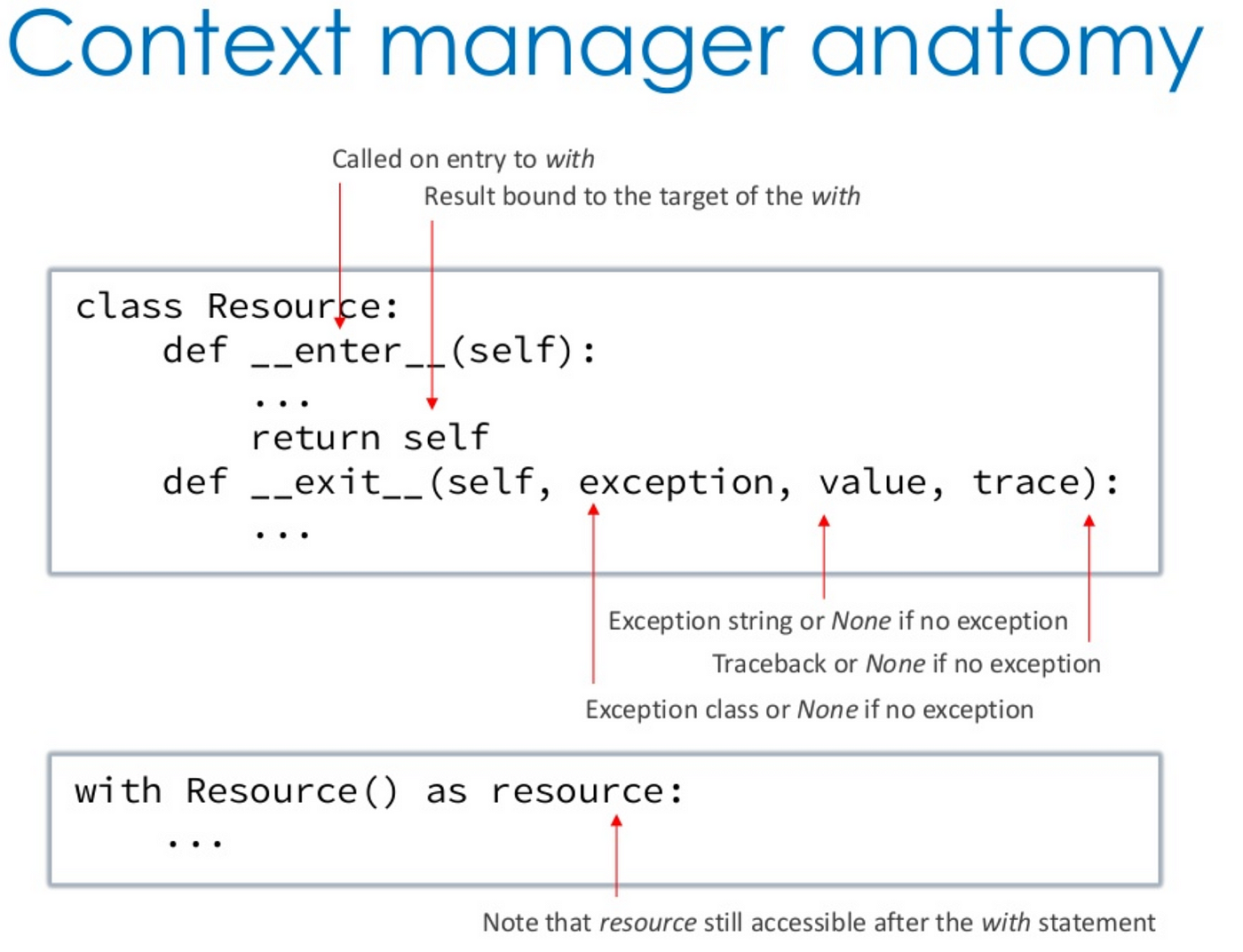
try:
self.file_handle = open(self.filename, 'r')
except Exception as e:
raise ValueError(f"Failed to open file {self.filename}: {str(e)}")
return self.file_handle
def exit(self, exc_type, exc_val, tb):
if self.file_handle is not None:
try:
self.file_handle.close()
except Exception as e:
print(f"Failed to close file {self.filename}: {str(e)}")
Usage example
with FileContextManager('test.txt') as file:
Use the file handle here
In this example, the FileContextManager
class opens a file and returns the file handle when you enter the context (__enter__
). When you exit the context (__exit__
), it closes the file. This ensures that the file is always properly closed, even if an exception occurs.
Here are some key points to note about context managers:
1. __enter__
: This method sets up the runtime context for your code block. It usually opens files, establishes connections or performs any other setup tasks required before running the code within the with statement.
2. __exit__
: This method cleans up the resources set up by __enter__
. It usually closes files, releases connections, or performs any other teardown tasks needed after the code block finishes execution.
3. The with
statement is used to create a context manager. Within this with
statement, you can use the returned object (in this case, the file handle) as if it were in a try-finally block.
4. If any exception occurs within the with statement, Python will automatically call the __exit__
method.
You can apply this concept to various situations like network connections, database queries, or even ensuring that certain tasks are executed before and after a code block.