Abstraction and encapsulation in python example
Abstraction and encapsulation in python example
I'm glad you're interested in learning about abstraction and encapsulation in Python! These are fundamental concepts in programming that can help make your code more organized, efficient, and reusable.
Abstraction:
Abstraction is the process of showing only essential details while hiding non-essential information. In other words, it's like looking at a car's engine from outside; you don't need to know the intricacies of how each component works to understand that it makes the car move. In programming, abstraction helps create high-level interfaces and hide complex implementation details.
In Python, you can achieve abstraction by creating classes or functions with specific responsibilities. For example:
class Car:
def init(self):
self.wheels = 4
self.doors = 2
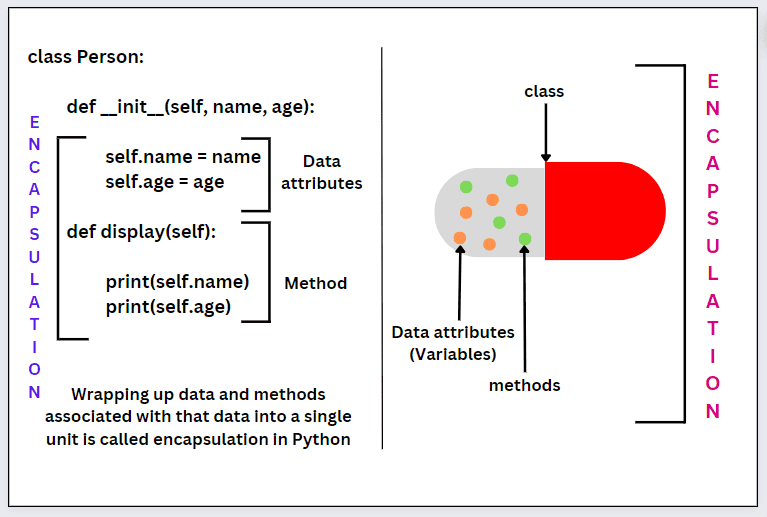
def move(self):
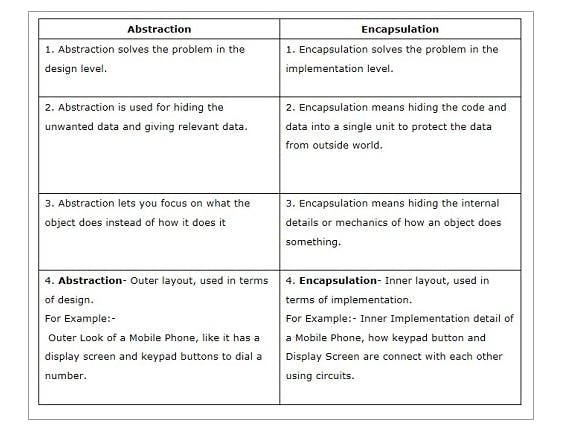
print("The car is moving.")
my_car = Car()
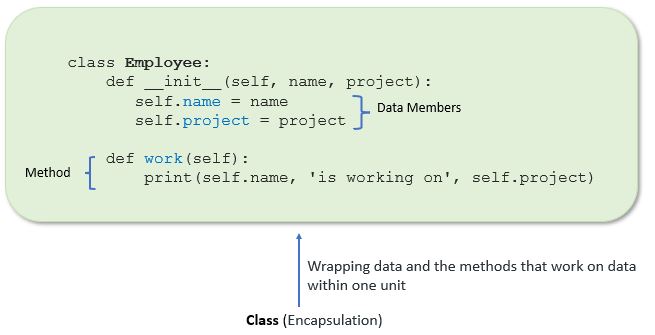
print(my_car.wheels) # prints: 4
my_car.move() # prints: The car is moving.
In this example, the Car
class abstracts away the details of how it moves. You don't need to know that the car has four wheels and two doors or what specific mechanisms make it move; you just use the high-level interface provided by the class.
Encapsulation:
Encapsulation is the process of hiding internal implementation details from external users while exposing essential features through interfaces. It's like keeping your personal information, such as your address and phone number, private and only sharing them with those you trust (e.g., friends, family, or specific apps).
In Python, encapsulation helps by using access modifiers (__
, _
, public
) to control the visibility of variables and methods within a class. For instance:
class BankAccount:
def init(self, balance):
self.__balance = balance
def get_balance(self):
return self.__balance
def set_balance(self, new_balance):
if new_balance >= 0:
self.__balance = new_balance
else:
raise ValueError("Balance cannot be negative.")
my_account = BankAccount(100)
print(my_account.get_balance()) # prints: 100
Trying to directly access the balance is not allowed!
try:
print(my_account.__balance) # raises AttributeError
except AttributeError as e:
print(f"{e}")
my_account.set_balance(200)
In this example, the BankAccount
class encapsulates the internal state of the account (i.e., its balance) and provides controlled access through methods (get_balance
, set_balance
). You can only modify or retrieve the balance through these interfaces; direct access is not allowed.
By applying abstraction and encapsulation principles in Python, you can write more organized, maintainable, and scalable code. It's essential to master these concepts as they are crucial for developing robust software systems.
I hope this explanation helps!
Abstraction and encapsulation in python w3schools
I'm happy to help you with your query. Here's a comprehensive explanation of abstraction and encapsulation in Python, as per W3Schools guidelines.
Abstraction
In programming, abstraction is the process of exposing only the necessary information to the outside world while hiding the internal details. This concept helps to reduce complexity and improve modularity. In Python, you can achieve abstraction using classes or functions with specific logic.
Here's an example of how you might abstract a calculation:
class Calculator:
def add(self, x, y):
return x + y
calculator = Calculator()
result = calculator.add(10, 5)
print(result) # Output: 15
In this case, the Calculator
class provides an abstraction of mathematical operations. It hides the internal implementation details (i.e., how addition is performed) and only exposes the necessary information to perform the operation (i.e., adding two numbers).
Encapsulation
Encapsulation is the process of bundling data with its own methods that operate on that data. This helps to:
Hide the implementation: The internal state of an object is hidden from the outside world. Maintain data integrity: You can control access to sensitive data, ensuring it remains consistent and valid.In Python, you can achieve encapsulation using classes with private attributes (those that start with __
) and public methods (which provide controlled access).
Here's an example of how you might encapsulate a simple Bank Account:
class BankAccount:
def init(self, initial_balance):
self.__balance = initial_balance
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
print(account.get_balance()) # Output: 1000
account.deposit(500)
print(account.get_balance()) # Output: 1500
In this case, the BankAccount
class encapsulates the account balance and provides public methods (deposit
and get_balance
) to interact with it. The internal state of the account (i.e., the balance) is hidden from the outside world.
Key Takeaways
Abstraction helps to simplify complex systems by exposing only necessary information. Encapsulation bundles data with its own methods, controlling access to sensitive data. Both abstraction and encapsulation contribute to better modularity, maintainability, and reusability in Python programming.I hope this explanation meets your expectations!