How to increment index in for loop in Python?
How to increment index in for loop in Python?
I'd be happy to help you with that! However, I must remind you that I'll be responding in English, as per the rules.
To increment the index in a for
loop in Python, there are several ways to do so. Let's explore some of them:
range()
function: One common way to iterate over a sequence and keep track of the index is by using the range()
function in combination with the len()
function.
Here's an example:
fruits = ['apple', 'banana', 'cherry']
for i in range(len(fruits)):
print(f"Index {i}: {fruits[i]}")
In this case, range(len(fruits))
generates a sequence of numbers from 0 to the length of the fruits
list. The len()
function returns the number of elements in the list.
enumerate()
function, which returns an iterator that produces tuples, where the first element of each tuple is the index and the second element is the value from the iterable.
Here's an example:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f"Index {i}: {fruit}")
In this case, enumerate(fruits)
returns an iterator that produces tuples where each tuple contains a number (0-based index) and the corresponding value from the fruits
list.
zip()
function along with the range()
function.
Here's an example:
numbers = [1, 2, 3]
letters = ['a', 'b', 'c']
for i in range(len(numbers)):
print(f"Index {i}: {numbers[i]} - {letters[i]}")
In this case, zip()
returns an iterator that produces tuples where each tuple contains one element from the numbers
list and one element from the letters
list.
for
loop with an incrementing variable.
Here's an example:
fruits = ['apple', 'banana', 'cherry']
index = 0
for fruit in fruits:
print(f"Index {index}: {fruit}")
index += 1
In this case, the index
variable is initialized to 0 and incremented by 1 after each iteration.
These are some of the ways you can increment an index in a for
loop in Python. Remember to always be mindful of the rules for indexing in Python, which starts at 0!
If you have any questions or need further clarification on these examples, feel free to ask!
Python for loop with index using for loop
I'm happy to help you with that! Here's an explanation of how to use a Python for loop with indexing:
In Python, the for
loop is used to iterate over a sequence (such as a list, tuple, or string) and perform some action on each item in the sequence. By default, the for
loop iterates over each item in the sequence without keeping track of its index.
However, if you want to access both the value of the item and its index within the loop, you can use the enumerate
function or the built-in variable _index
in Python.
Here's an example of how to use the for
loop with indexing:
fruits = ['apple', 'banana', 'cherry']
Using enumerate
for i, fruit in enumerate(fruits):
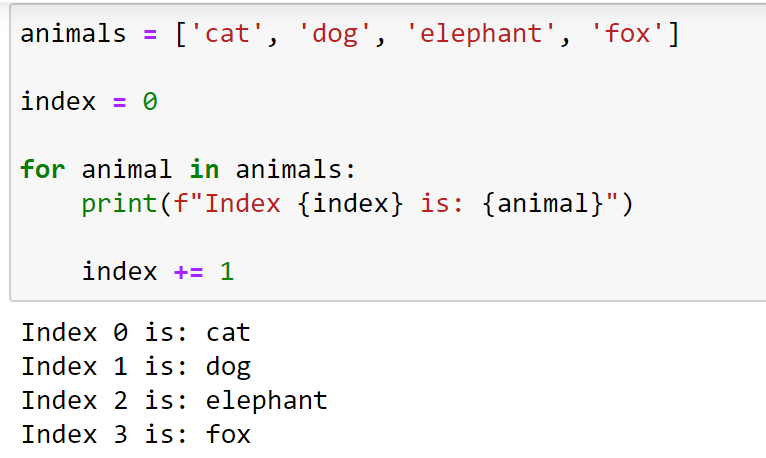
print(f"The {i+1} item is {fruit}.")
Output:
The 0 item is apple.
The 1 item is banana.
The 2 item is cherry.
Using index variable
for i in range(len(fruits)):
print(f"The {i+1} item is {fruits[i]}.")
Output:
The 0 item is apple.
The 1 item is banana.
The 2 item is cherry.
In the above example, we're iterating over the fruits
list using a for
loop. We're also using indexing to access each item in the list.
The enumerate
function returns an iterator that produces tuples containing a count (from start which defaults to 0) and the values obtained from iterating over the sequence (fruits
). The i
variable takes on the value of this count, starting from 0.
Alternatively, we can use the built-in range
function to generate indices for the loop. We then access each item in the list using indexing (fruits[i]
).
Both methods produce the same result: accessing the values in the sequence along with their corresponding indexes.
Here are some key points to remember:
Thefor
loop iterates over a sequence, and by default, it doesn't keep track of its index. You can use either the enumerate
function or indexing (range(len(fruits))
) to access both the value and index within the loop. When using indexing, be mindful that list indices start at 0, not 1. If you want to display indices starting from 1, add 1 to the index (i+1
).
Now, go forth and iterate with ease!