When should you use yield in Python?
When should you use yield in Python?
I'd be happy to provide an answer in English!
In Python, the yield
keyword is used to define a generator function, which is a special type of function that can be used to create iterators.
A generator function is a normal function that contains one or more yield
statements. When the function is called, it returns an iterator object. Each time you iterate over this object using a for loop or the next()
function, the function is executed until it reaches the next yield
statement, at which point it pauses and returns the value yielded.
You should use yield
in Python when:
yield
can help you achieve lazy evaluation. For example, consider a function that calculates the sum of all numbers up to a given number. Instead of calculating the sum upfront and returning it, you can use yield
to return each number in the range as you iterate over it. Handling Large Datasets: When working with large datasets, using yield
can be an efficient way to process the data. For instance, consider a function that reads lines from a large file. Instead of loading the entire file into memory, you can use yield
to yield each line as you read it. Cooperative Multitasking: In Python 3.x, you can use yield
in a coroutine to implement cooperative multitasking. A coroutine is a special type of generator that can be used to implement asynchronous programming using the async
and await
keywords. Creating Infinite Iterators: When you need to create an infinite iterator, such as an iterator over all prime numbers or all Fibonacci numbers, yield
can help you achieve this. You can use a recursive function with a base case that yields each value in the sequence.
Here's a simple example of using yield
:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib_gen = fibonacci()
for _ in range(10):
print(next(fib_gen))
In this example, the fibonacci()
function is a generator that yields each Fibonacci number up to a certain point (in this case, 10 numbers). The next()
function is used to iterate over the generator and print each value.
In conclusion, yield
is an important keyword in Python that allows you to define generators, which can be used for lazy evaluation, handling large datasets, cooperative multitasking, and creating infinite iterators. It's a powerful tool that can help you write more efficient and effective code.
What is yield in Python?
I'd be happy to help!
Yield is a built-in Python function that returns the next value from an iterator, allowing you to process data one item at a time. It's often used to implement iterators and generators.
A generator is a special type of iterator in Python that can remember its state between function calls. When you use yield, your function becomes a generator. You can think of it like a paused program that can be restarted from where you left off.
Here's an example of how yield works:
def my_generator():
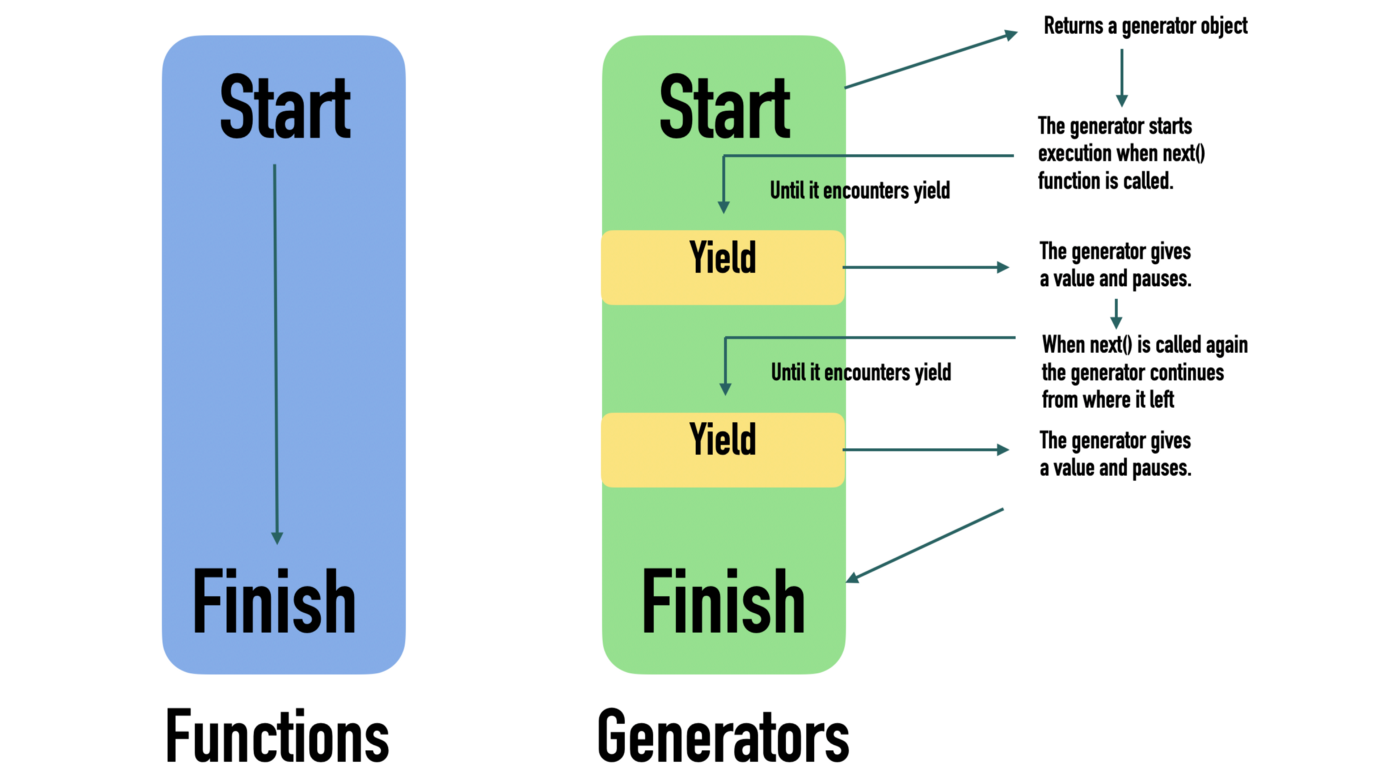
for i in range(3):
yield i
g = my_generator()
print(next(g)) # prints 0
print(next(g)) # prints 1
print(next(g)) # prints 2
In this code, we define a generator function called my_generator
. Inside the function, we use yield
to produce the values from 0 to 2.
When we call my_generator()
, it returns an iterator that remembers its state. The first time we call next(g)
, it gives us the first value (0). The next time we call next(g)
, it gives us the second value (1), and so on.
Yield has a few key benefits:
It allows you to process data one item at a time, which can be very useful for working with large datasets or processing infinite streams. It helps keep your memory usage down by only keeping track of one item in memory at any given time. It makes it easy to create iterators and generators that can be reused or shared.Yield is often used in conjunction with other Python features like for
loops, list comprehensions, and the itertools
module. It's a powerful tool for working with data in Python!
So, that's yield in Python! I hope this helps you understand it better.