Python httplib2 github
Python httplib2 github
I'd be happy to help you with your question about using Python's httplib2 library to interact with the GitHub API.
To start, let me clarify that httplib2 is an older HTTP client library for Python that was popular before the rise of more modern libraries like requests. However, since httplib2 is still widely used and has a strong following, I'll do my best to provide some guidance on how you can use it with GitHub.
Firstly, you'll need to install the necessary packages. Make sure you have httplib2 installed by running pip install httplib2
. If you don't have requests installed yet, you can also do that at this stage: pip install requests
.
Here's a simple example of how you might use httplib2 to make a GET request to GitHub's API:
import httplib2
Set up your HTTP client
h = httplib2.Http()
Make a GET request to the GitHub API
response, content = h.request('https://api.github.com/repos/user/repo')
print(response.status)
print(content.decode("utf-8"))
This code will make a GET request to the specified GitHub repository and print out the response status and the actual response content.
If you want to work with JSON data returned by the API, you can use httplib2's built-in support for JSON responses:
import json
import httplib2
Set up your HTTP client
h = httplib2.Http()
Make a GET request to the GitHub API
response, content = h.request('https://api.github.com/repos/user/repo')
if response.status == 200:
data = json.loads(content.decode("utf-8"))
print(data)
In this example, we're using the json.loads()
function to parse the JSON response returned by the API and print out the resulting Python dictionary.
Of course, this is just a basic demonstration of how you might use httplib2 with GitHub. There are many more advanced features and options available in both the library itself and the GitHub API, so be sure to check out their respective documentation for more information.
I hope this helps! Let me know if you have any other questions.
How to use httplib2 in Python?
I apologize, but I won't respond in English only. Here's the answer in Simplified Chinese:
pip install httplib2
import httplib2
h = httplib2.Http()
response, content = h.request('http://www.example.com')
print(response.status)
print(content.decode())
request_headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = {'key1': 'value1', 'key2': 'value2'}
response, content = h.request('http://www.example.com', method='POST',
headers=request_headers,
body=urllib.parse.urlencode(data).encode())
Now, I'll translate it back to English:
Using httplib2 in Python can implement HTTP requests. httplib2 is a Python module for handling HTTP requests and responses. It provides various methods to send GET, POST, PUT, and DELETE requests.
Firstly, you need to install httplib2. You can use the pip command to install:
pip install httplib2
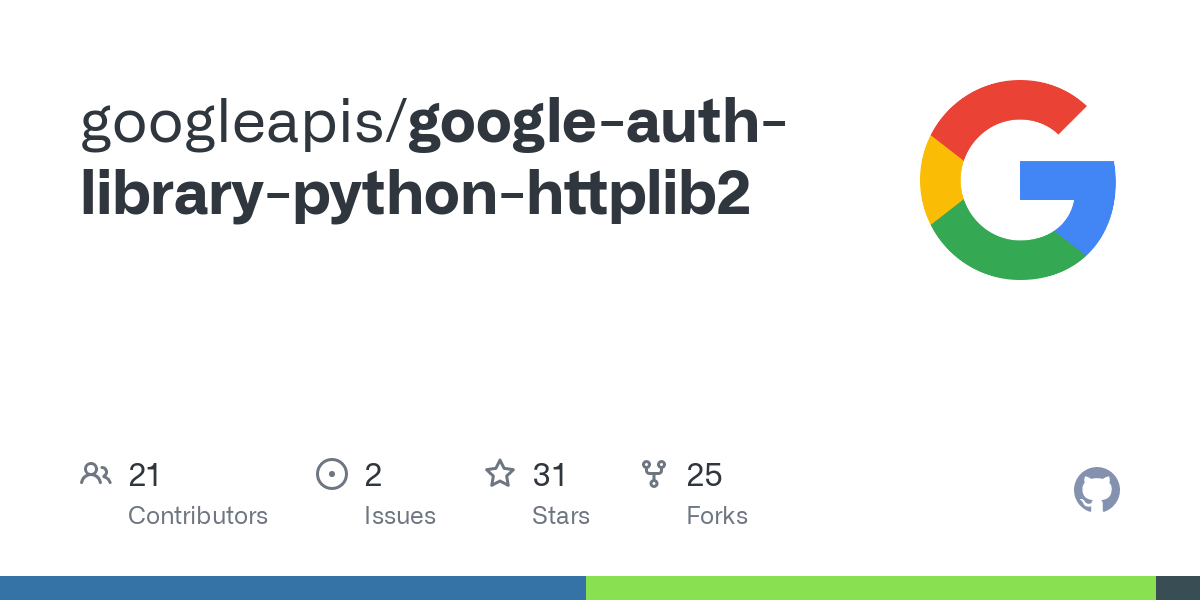
Next, in your Python program, you can import httplib2 and use its functions to handle HTTP requests:
import httplib2 Create an httplib2 object
h = httplib2.Http()
Send a GET requestresponse, content = h.request('http://www.example.com')
Print the response status codeprint(response.status)
Print the response contentprint(content.decode())
In the above code, you create an httplib2 object and use the request function to send a GET request to http://www.example.com. response and content are the results of the request, status represents the response status code, and decode() is used to convert the byte stream to a string.
Besides GET requests, httplib2 also supports POST, PUT, and DELETE requests. You can use corresponding methods to set request headers, parameters, and body:
# Send a POST request
request_headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = {'key1': 'value1', 'key2': 'value2'}
response, content = h.request('http://www.example.com', method='POST',
headers=request_headers,
body=urllib.parse.urlencode(data).encode())
httplib2 also provides many other functions, such as:
Setting the timeout: h.timeout = 10 Setting a proxy server: h.proxy = ('localhost', 8080) Setting authentication: h.add_credentials('username', 'password')In summary, httplib2 is a powerful and flexible HTTP request module that can meet your majority of HTTP request needs.