Python serverless example
Python serverless example
Here is an example of a Python-based serverless application:
Serverless computing is a cloud computing model where the cloud provider manages the infrastructure and dynamically allocates computing resources as needed. With this approach, you don't have to manage any servers or worry about scaling your application.
In this example, we will create a simple serverless API using AWS Lambda and API Gateway. This API will respond to GET requests and return a message in JSON format.
Here are the steps:
Install the necessary libraries:You can install them by running the following command:
pip install boto3
Create an IAM role:
This role should have the necessary permissions for your Lambda function. You can do this by navigating to the AWS Management Console, selecting the IAM service, and then clicking on "Roles".
Create a Lambda function:Create a new Lambda function with Python as the runtime. You'll need to give it a name, such as my-serverless-api
. Set the handler to index.handler
, which will be our entry point.
Here's an example of what the code might look like:
import boto3
dynamodb = boto3.resource('dynamodb')
table_name = 'my-table'
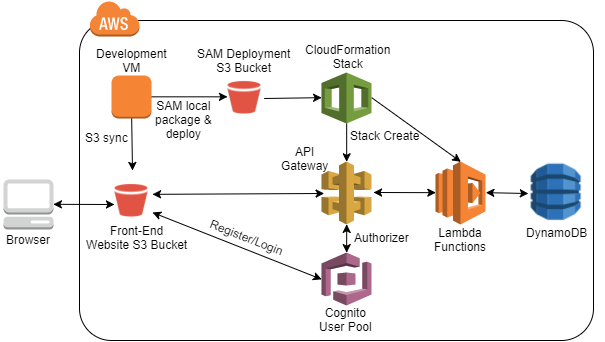
def lambda_handler(event, context):
table = dynamodb.Table(table_name)
if event['httpMethod'] == 'GET':
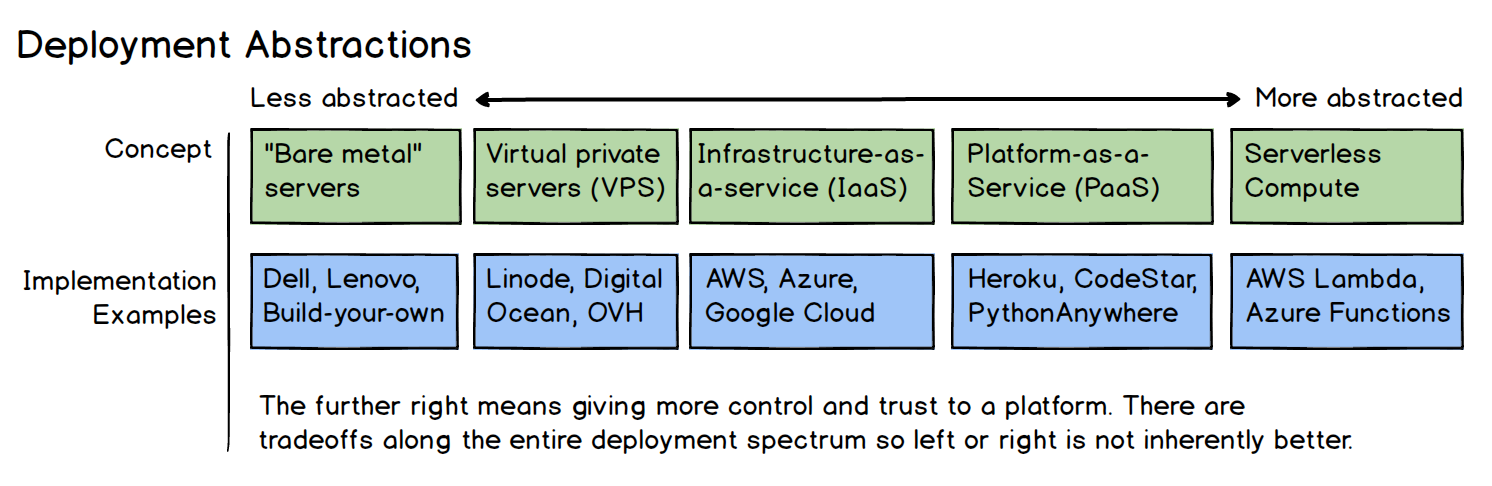
Return a message in JSON format
return {
'statusCode': 200,
'body': JSON.stringify({
"message": "Hello from serverless!"
})
}
In the AWS Management Console, select the API Gateway service and click on "Create API". Choose the REST API option and give it a name, such as my-serverless-api
.
Click on the "Integration Request" button in the "Resources" tab. Then, select "Lambda Function" as the integration type and choose your Lambda function from the list.
Test your API:To test your API, you can use a tool like curl
or Postman to send a GET request to your API Gateway endpoint.
Here's an example of how this might look:
curl -XGET https://execute-api.us-east-1.amazonaws.com/my-serverless-api/
Monitor and maintain:
Use CloudWatch to monitor the performance and errors of your serverless application. You can also use AWS X-Ray to visualize the performance of your function.
This example demonstrates a simple serverless API that responds to GET requests and returns a message in JSON format. Of course, this is just the tip of the iceberg when it comes to what's possible with serverless computing!
Some benefits of using Python for serverless computing include:
Fast execution: Python's Just-In-Time (JIT) compiler ensures that your code executes quickly. Low memory usage: Python's garbage collector helps keep memory usage low, making it a good choice for serverless applications. Large community: Python has a large and active community, which means there are many resources available to help you learn and troubleshoot.I hope this example gives you a sense of what it's like to build a serverless application using Python!
What is serverless in Python?
Serverless computing, also known as Function-as-a-Service (FaaS), is a cloud-based model where applications are broken down into smaller, discrete functions that are executed on-demand by the cloud provider. In other words, instead of managing and provisioning servers to run your code, you write and deploy small, single-purpose functions that can be triggered and scaled automatically based on demand.
In Python, serverless computing is made possible through frameworks such as AWS Lambda, Google Cloud Functions, or Azure Functions. These platforms provide a managed environment for executing your Python code without the need to worry about infrastructure provisioning, scaling, or patching.
Here are some key benefits of using serverless computing in Python:
Cost-effective: You only pay for the compute time consumed by your code, rather than maintaining servers that may be idle most of the time. Scalability: Cloud providers automatically scale your functions based on demand, so you don't need to worry about handling sudden spikes or dips in traffic.To get started with serverless computing in Python, you'll typically follow these steps:
Here's an example of a simple Python function on AWS Lambda:
import boto3
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('my-table')
def lambda_handler(event, context):
Read data from DynamoDB table
item = table.get_item(Key={'id': event['id']}).Item
Perform some business logic
result = process_data(item)
return {
'statusCode': 200,
'body': json.dumps({'message': 'Data processed successfully'})
}
In this example, the lambda_handler
function is triggered by an HTTP request and reads data from a DynamoDB table. It then performs some business logic and returns a JSON response.
Serverless computing in Python offers a powerful way to build scalable, cost-effective applications that can handle varying levels of traffic and demand. By leveraging cloud providers' managed services, you can focus on writing code rather than managing infrastructure, making it an attractive option for many developers.