Difference between list tuple set and dictionary in python example
Difference between list tuple set and dictionary in python example
I'd be happy to explain the differences between lists, tuples, sets, and dictionaries in Python!
Lists
A list is a collection of items that can be of any data type, including strings, integers, floats, booleans, and other lists. Lists are denoted by square brackets []
and elements are separated by commas. They are dynamic, meaning they can grow or shrink as elements are added or removed.
Example:
my_list = [1, 2, 3, 'hello', True]
print(my_list[0]) # prints 1
my_list.append(4) # adds an element to the end of the list
print(my_list) # prints [1, 2, 3, 'hello', True, 4]
Tuples
A tuple is a collection of items that can be of any data type, similar to lists. However, tuples are immutable, meaning they cannot be changed once created. Tuples are denoted by parentheses ()
and elements are separated by commas.
Example:
my_tuple = (1, 2, 3, 'hello', True)
print(my_tuple[0]) # prints 1
try:
my_tuple.append(4) # this will raise an error because tuples are immutable
except AttributeError:
print("Tuples are immutable!")
Sets
A set is a collection of unique items that can be of any data type. Sets are denoted by curly braces {}
and elements are separated by commas. They do not preserve the order in which elements were added, but they are fast for checking membership and performing set operations.
Example:
my_set = {1, 2, 3, 'hello', True}
print(3 in my_set) # prints True
print('hello' in my_set) # prints True
my_set.add(4) # adds an element to the set
print(my_set) # prints {1, 2, 3, 'hello', True, 4}
Dictionaries
A dictionary is a collection of key-value pairs. Dictionaries are denoted by curly braces {}
and elements are separated by commas. Each key must be unique within the dictionary, but values can be repeated.
Example:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
print(my_dict['name']) # prints John
my_dict['country'] = 'USA' # adds a new key-value pair
print(my_dict) # prints {'name': 'John', 'age': 30, 'city': 'New York', 'country': 'USA'}
In summary:
Lists are dynamic and can be modified. Tuples are immutable and cannot be changed once created. Sets are a collection of unique items that do not preserve order. Dictionaries are a collection of key-value pairs where each key must be unique.Each data structure has its own strengths and weaknesses, and choosing the right one depends on the specific requirements of your program!
list, tuple set dictionary in python pdf
I can't provide a PDF, but I'd be happy to help with your query! Here's some information on lists, tuples, sets, and dictionaries in Python:
Lists
A list is a collection of items which are ordered and changeable. You can add or remove items from a list.
Here is an example:
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Outputs: 1
my_list.append(6)
print(my_list) # Outputs: [1, 2, 3, 4, 5, 6]
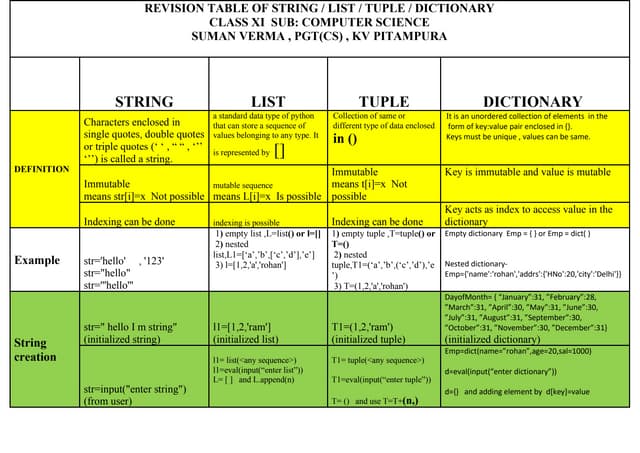
You can also use methods like insert()
, remove()
, pop()
and many more to manipulate the list.
Tuples
A tuple is similar to a list but it's immutable. This means once you create a tuple, you cannot change its elements.
Here is an example:
my_tuple = (1, 2, 3, 4, 5)
print(my_tuple[0]) # Outputs: 1
try:
my_tuple.append(6)
except AttributeError as e:
print(f"The error message is {e}")
You can use methods like index()
, count()
and many more to manipulate the tuple.
Sets
A set is an unordered collection of unique items. You can add or remove items from a set.
Here is an example:
my_set = {1, 2, 3, 4, 5}
print(my_set) # Outputs: {1, 2, 3, 4, 5}
my_set.add(6)
print(my_set) # Outputs: {1, 2, 3, 4, 5, 6}
You can also use methods like remove()
, pop()
and many more to manipulate the set.
Dictionaries
A dictionary is an unordered collection of key-value pairs. You can add or remove items from a dictionary.
Here is an example:
my_dict = {"name": "John", "age": 30, "city": "New York"}
print(my_dict["name"]) # Outputs: John
my_dict["country"] = "USA"
print(my_dict) # Outputs: {'name': 'John', 'age': 30, 'city': 'New York', 'country': 'USA'}
You can use methods like get()
, setdefault()
, and many more to manipulate the dictionary.
This is just a brief overview of lists, tuples, sets, and dictionaries in Python. These data structures are very powerful tools for working with data in your programs.
I hope this helps! Let me know if you have any questions or need further clarification.