Python JSON dumps
Python JSON dumps
I'd be happy to respond in English and provide information about Python's JSON (JavaScript Object Notation) dumping capabilities.
JSON is a popular data interchange format that is widely used for exchanging data between web servers, web applications, and mobile apps. In Python, we can use the json
module to convert Python objects into JSON strings, which are then easily readable by humans and machines alike.
To dump a Python object as JSON using the json
module, you can use the dumps()
function, which stands for "dump in standard format". Here's an example:
import json
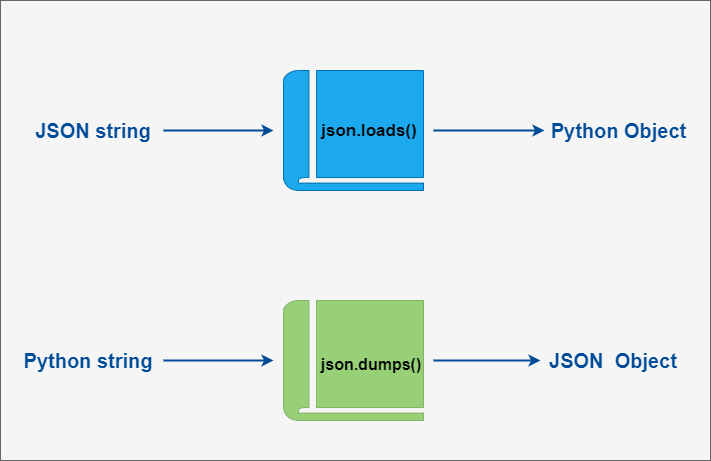
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
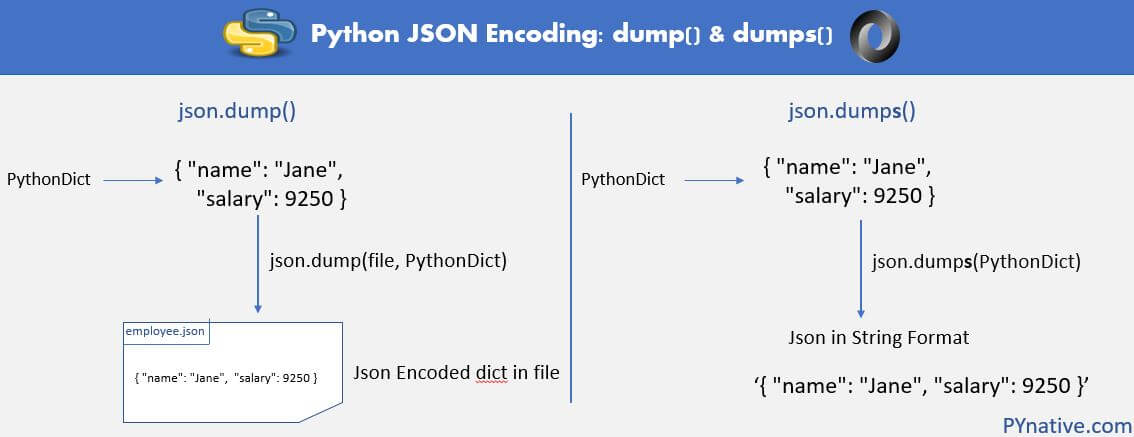
}
Convert person dictionary to JSON string
json_person = json.dumps(person)
print(json_person)
When you run this code, the output will be a JSON-formatted string:
{"name": "John Doe", "age": 30, "city": "New York"}
The dumps()
function takes an optional argument called indent
, which specifies the number of spaces to use for indentation. For example, if you set indent
to 4, the JSON output will be indented with four spaces:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Convert person dictionary to JSON string with indentation
json_person = json.dumps(person, indent=4)
print(json_person)
This will produce the following output:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
As you can see, the JSON output is nicely formatted with indentation.
In addition to the dumps()
function, the json
module also provides the dump()
function for writing JSON data to a file. Here's an example:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Write person dictionary to JSON file
with open('person.json', 'w') as f:
json.dump(person, f)
print("JSON file written successfully!")
When you run this code, a new file called person.json
will be created in the same directory, containing the following contents:
{"name": "John Doe", "age": 30, "city": "New York"}
Overall, Python's json
module provides convenient functions for converting Python objects to JSON strings and files. Whether you're working with web applications, data science, or scientific computing, JSON is a widely used format that can help facilitate data exchange and processing.
Python JSON load
I'm happy to help you with your question!
To load a JSON file using Python, you can use the json
module, which is included in the standard library. Here's an example of how to do it:
import json Load the JSON file
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
In this example, we're assuming that you have a JSON file named data.json
in the same directory as your Python script. The file contains a JSON object (or array) that we want to load into our Python program.
The json.load()
function is used to parse the JSON file and return it as a Python object. In this case, we're opening the file with open('data.json', 'r')
, which opens the file in read mode ('r'
). The with
statement ensures that the file is properly closed after we're done with it.
The json.load()
function returns a dictionary (or list) that represents the JSON object or array. You can then access the values in the JSON object using standard Python dictionary methods, such as .keys()
, .values()
, and .items()
.
Here's an example of how you might use this loaded data:
import json Load the JSON file
with open('data.json', 'r') as f:
data = json.load(f)
for item in data['items']:
print(item['name'], item['age'])
In this example, we're assuming that our JSON file contains a dictionary with an items
key that points to a list of objects. Each object has name
and age
keys.
If you have a more complex JSON structure, such as nested dictionaries or arrays, the json.load()
function will still work correctly. For example:
import json Load the JSON file
with open('data.json', 'r') as f:
data = json.load(f)
for person in data['people']:
for skill in person['skills']:
print(person['name'], skill)
In this example, we're assuming that our JSON file contains a dictionary with people
and skills
keys. Each person
dictionary has name
, age
, and skills
keys, which is an array of strings.
These are just a few examples of how you can use the json.load()
function to load and work with JSON data in Python. I hope this helps! Let me know if you have any questions or need further clarification.