Can Python read XML files?
Can Python read XML files?
Yes, Python can definitely read XML files! In fact, there are several ways to do so, depending on your specific needs and preferences.
One way is to use the xml.etree.ElementTree
module, which is a built-in part of the Python Standard Library. This module provides a simple and efficient way to parse and manipulate XML documents. You can use its various methods, such as parse()
, fromstring()
, or Element()
to load an XML file into memory.
Here's a basic example:
import xml.etree.ElementTree as ET
Load the XML file
tree = ET.parse('example.xml')
Get the root element
root = tree.getroot()
Iterate over the elements and print their text
for elem in root.findall('./*'):
print(elem.text)
Another way is to use a third-party library, such as xmltodict
. This library provides a more Pythonic way of working with XML documents, allowing you to easily convert XML into dictionaries or lists. It's particularly useful if you need to extract specific data from your XML files.
Here's an example using xmltodict
:
import xmltodict
Load the XML file
with open('example.xml', 'r') as f:
doc = xmltodict.parse(f.read())
Extract specific data from the XML document
person_data = doc['people']['person']
print(person_data['name'])
print(person_data['age'])
Yet another option is to use a library like lxml
, which provides a more comprehensive set of tools for working with XML. It includes support for parsing, serializing, and manipulating XML documents, as well as integration with other libraries and frameworks.
Here's an example using lxml
:
from lxml import etree
Load the XML file
root = etree.parse('example.xml').getroot()
Iterate over the elements and print their text
for elem in root.findall('./*'):
print(elem.text)
All of these methods have their own strengths and weaknesses, and the choice ultimately depends on your specific use case, requirements, and personal preferences.
In conclusion, Python can indeed read XML files using various built-in modules or third-party libraries. Whether you're working with simple parsing tasks or more complex data extraction requirements, there's a way to do it in Python!
How to deal with XML in Python?
I'll respond in English for this one.
XML (Extensible Markup Language) is a popular format for storing and transporting data between different systems, applications, or languages. In Python, you can work with XML files using various libraries and modules. Here are the ways to deal with XML in Python:
xml.etree.ElementTree: This is a built-in module that allows you to parse and manipulate XML documents. You can use it to read, write, and validate XML files. It's a powerful tool for working with XML. import xml.etree.ElementTree as ET
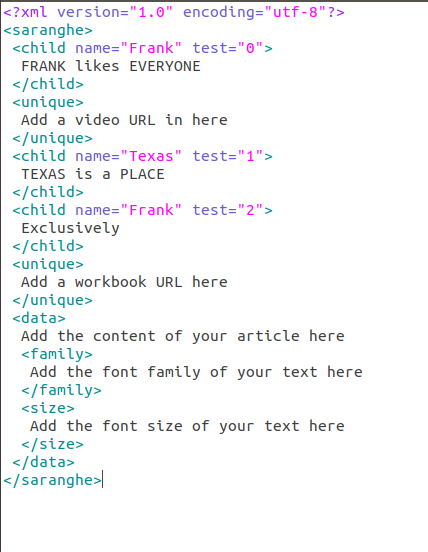
Parse an XML file
tree = ET.parse('your_file.xml')
root = tree.getroot()
Accessing elements in the XML document
for child in root:
print(child.tag, child.text)
Modifying the XML document
new_child = ET.Element('new_element')
root.append(new_child)
Writing the modified XML file
ET.dump_tree(root).write('output_file.xml', encoding='unicode', xml_declaration=True, short_empty_elements=False)
lxml: This is a more powerful and flexible library for parsing and manipulating XML files than the built-in xml.etree.ElementTree
. It provides better performance and supports more advanced features.
from lxml import etree
Parse an XML file
root = etree.parse('your_file.xml').getroot()
Accessing elements in the XML document
for child in root:
print(child.tag, child.text)
Modifying the XML document
new_child = etree.Element('new_element')
root.append(new_child)
Writing the modified XML file
etree.tostring(root, encoding='unicode', xml_declaration=True).decode().encode('utf-8').write('output_file.xml')
xml.dom.minidom: This module provides a simple way to work with XML documents, but it's not as powerful as xml.etree.ElementTree
and lxml
.
from xml.dom import minidom
Parse an XML file
dom = minidom.parseString('hello')
Accessing elements in the XML document
for child in dom.documentElement.childNodes:
print(child.nodeName, child.textContent)
Modifying the XML document
new_child = dom.createElement('new_element')
dom.documentElement.appendChild(new_child)
Writing the modified XML file
with open('output_file.xml', 'w') as f:
f.write(dom.toxml())
In conclusion, Python provides several ways to deal with XML files. The choice of which library or module to use depends on your specific needs and preferences.