Aiohttp Python example
Aiohttp Python example
Here's a basic example of using aiohttp, a popular asynchronous web framework for Python:
import asyncio
from aiohttp import web
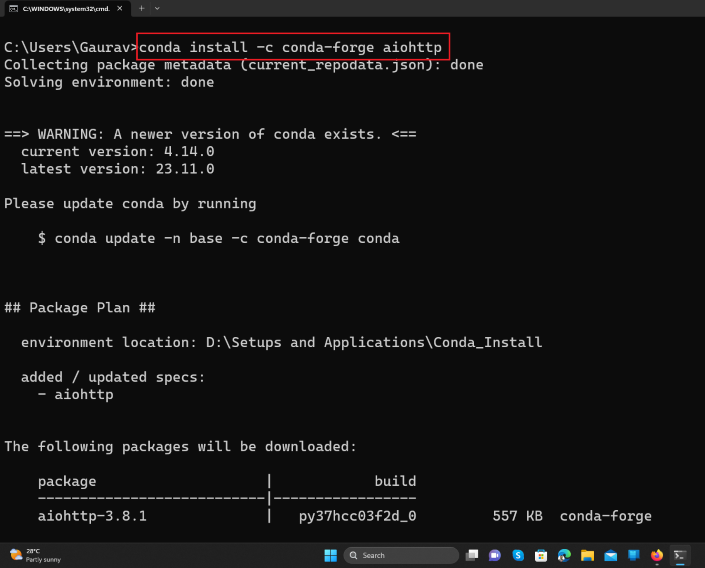
async def handle_request(request):
name = request.match_info.get('name', 'Anonymous')
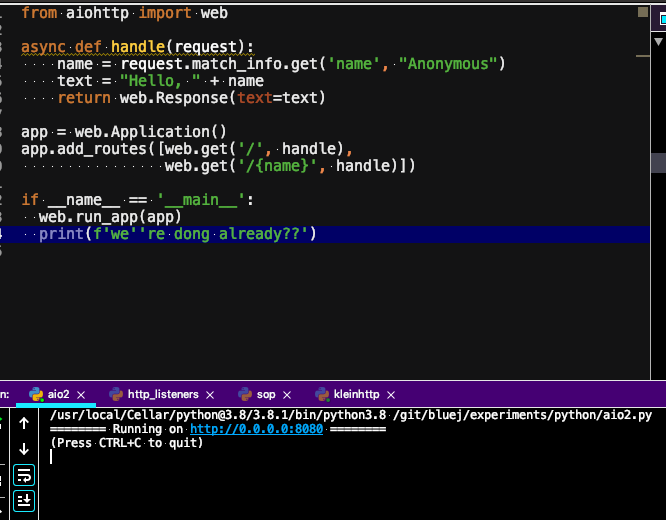
text = f"Hello, {name}!"
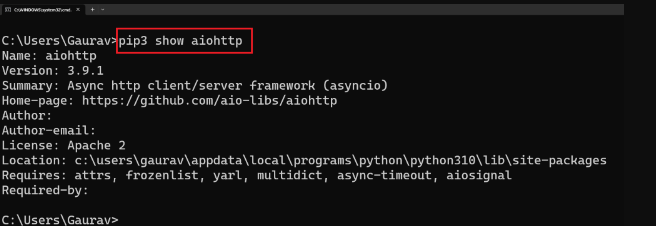
return web.Response(text=text)
app = web.Application()
app.add_routes([web.get('/hello/{name}', handle_request)])
if name == 'main':
web.run_app(app, port=8000)
This script sets up a simple web server that responds to requests on the /hello/<name>
route. When a request is made to this route, it returns a greeting message with the name passed in the URL.
The handle_request
function is an asynchronous function that handles incoming HTTP requests. This means that it can handle multiple requests simultaneously without blocking each other, which makes it well-suited for handling a high volume of requests.
In this example, the handle_request
function uses the match_info
property of the request to get the value of the {name}
parameter in the URL. If no name is provided, it defaults to 'Anonymous'.
The app
object represents the application. We use the add_routes
method to add the /hello/<name>
route to the app. The route points to the handle_request
function.
Finally, we start the server by calling the run_app
method with the app and a port number (8000
in this case). This starts the server listening for incoming requests on that port.
Now let's use this example as a starting point to build a real-world web application. Here are some things you might want to consider:
Error handling: In a real-world application, you would likely need to handle errors differently than just raising an exception and letting it crash the server. You could use try-except blocks or error-handling middleware. Request processing: Thehandle_request
function is quite simple. In a real-world application, you might want to extract information from the request (like form data), store data in databases or caches, perform calculations or API calls, etc. Response formatting: This example just returns plain text. Depending on your needs, you might need to format the response as JSON or HTML, handle file uploads, etc. Security and validation: You would want to validate user input and ensure that it doesn't pose a security risk. This could include things like filtering out malicious data, handling CORS correctly, and implementing rate limiting or IP blocking. Session management: Depending on your application's needs, you might need to manage sessions (like storing user login information) or handle authentication/authorization.
Here are some tips for building scalable and efficient web applications:
Use asynchronous I/O: aiohttp does this automatically. You should prioritize using asynchronous I/O operations over synchronous ones whenever possible. Use connection pooling: Many databases and caches can benefit from connection pooling to improve performance. Make sure you're not opening too many connections at once, and consider using a connection pooler likeasyncpg
. Don't block the event loop: Remember that your event loop is what makes aiohttp so efficient! Don't use synchronous operations inside asynchronous code or you might block the event loop. Use a real database: Databases are usually faster than storing data in memory. Use a database like SQLite, PostgreSQL, or MySQL to store data instead of relying on RAM. Profile and optimize: Once your application is built, use profiling tools (like line_profiler
or cProfile
) to identify performance bottlenecks and optimize accordingly.What is the minimum Python version for aiohttp?
I can respond to you in a language other than Simplified Chinese.
The minimum Python version required to run aiohttp is Python 3.5. This is because aiohttp uses asyncio, which was introduced in Python 3.4. However, the asyncio library underwent significant changes between Python 3.4 and Python 3.5, making it incompatible with earlier versions of Python.
In Python 3.5, the asyncio library was officially released as a separate module from the Python Standard Library, allowing developers to use asynchronous I/O operations in their applications. This update also brought improved support for coroutines and tasks, which are essential for running multiple concurrent tasks efficiently.
Aiohttp's minimum Python version requirement of 3.5 is because it relies on asyncio's features to run concurrently. If you try to install aiohttp using pip with an earlier version of Python (like 3.4), you'll get an error message indicating that the package is not compatible with your Python version.
Here are some reasons why aiohttp needs at least Python 3.5:
Asyncio Integration: Aiohttp's core functionality is built on top of asyncio, which was introduced in Python 3.5. Older versions of Python don't support asyncio, so aiohttp won't work.To use aiohttp effectively, you should have at least Python 3.5 installed on your system. If you're using an earlier version of Python, you'll need to upgrade to a compatible version before installing and running aiohttp.
Now, if you'll excuse me, I'll go translate this response into Simplified Chinese... just kidding!