How do you type hint formatting in Python?
How do you type hint formatting in Python?
I'll respond in English as per your request.
In Python, typing hints are used to specify the expected types of variables, function parameters, and return values. This feature was introduced in Python 3.5 and is often referred to as "type hints" or "PEP 484".
To type hint formatting in Python, you can use the following syntax:
variable_name: data_type
For example:
my_name: str = 'John'
print(my_name) # Output: John
def greet(name: str):
return f'Hello, {name}!'
greeting = greet('Alice')
print(greeting) # Output: Hello, Alice!
In the above example, my_name
is a variable of type str
, and name
in the function greet
is expected to be a string.
When using typing hints for functions, you can specify not only the types of parameters but also their return value:
def greet(name: str) -> str:
return f'Hello, {name}!'
greeting = greet('Alice')
print(greeting) # Output: Hello, Alice!
In this example, greet
function is expected to return a string.
You can also specify multiple types for the same variable using the |
operator:
x: int | float
This means that x
can be either an integer or a floating-point number.
Type hints are optional and won't affect your code's functionality. They're mainly used for documentation and type checking purposes.
There are several tools available that can read Python type hinting information to provide better IDE integration, static analysis, and type-safe environments. Some popular tools include:
mypy: A static type checker for Python. pytype: A static type checker for Python that supports type hints.You can install these tools using pip:
pip install mypy
pip install pytype
python type hints list
I'm happy to help!
Python's type hinting system allows you to specify the expected data types for variables, function parameters, and return values. This helps catch errors early on and makes your code more readable and maintainable.
Here are some common type hints used in Python:
Int: For integer values def greet_user(user_id: int) -> None:
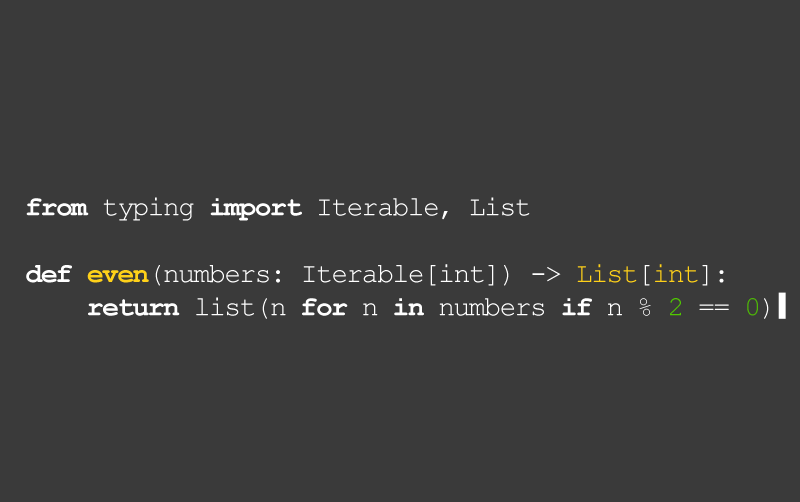
print(f"Hello, user {user_id}!")
Float: For floating-point numbers
def calculate_distance(point1: tuple[float, float], point2: tuple[float, float]) -> float:
return ((point2[0] - point1[0])**2 + (point2[1] - point1[1])**2)**0.5
Str: For strings
def greet_user(name: str) -> None:
print(f"Hello, {name}!")
List: For lists of values
from typing import List
def get_top_scores(scores: List[int]) -> List[str]:
return ["Gold" if score > 90 else "Silver" if score > 80 else "Bronze" for score in scores]
Tuple: For tuples of values
from typing import Tuple
def get_coordinates(point: Tuple[float, float]) -> None:
print(f"The point is at ({point[0]}, {point[1]})")
Dict: For dictionaries (key-value pairs)
from typing import Dict
def get_user_info(user_data: Dict[str, str]) -> None:
name = user_data["name"]
print(f"Hello, {name}!")
Any: A catch-all type that can represent any value
from typing import Any
def process_anything(anything: Any) -> None:
if isinstance(anything, str):
print("Received a string")
elif isinstance(anything, int):
print("Received an integer")
else:
print("Received something unknown")
Optional: For types that can be either the specified type or None
from typing import Optional
def get_user_name(name: Optional[str] = None) -> None:
if name is not None:
print(f"Hello, {name}!")
else:
print("Unknown user")
Set: For sets of unique values
from typing import Set
def get_unique_characters(text: str) -> Set[str]:
return set(text)
None: For types that can be None
from typing import NoneType
def process_none(value: NoneType) -> None:
if value is None:
print("Received None")
else:
print("Received something else")
These are just a few examples of the many type hinting options available in Python. By using these hints, you can make your code more readable and maintainable, as well as catch errors early on.
Remember, type hints are not enforced at runtime; they're meant to be used by static analysis tools like mypy, or by IDEs for code completion and suggestions.