How do you read and write an existing file in Python?
How do you read and write an existing file in Python?
I'm happy to respond in English for this special request!
Reading an Existing File in Python
Python provides several ways to read an existing file, depending on the format of the file and your specific requirements. Here are a few common methods:
Text Files: For text files (e.g.,.txt
, .log
, etc.), you can use the built-in open
function to open the file in read mode ('r'
). The file is automatically closed when you're done with it.
Example:
with open('example.txt', 'r') as file:
content = file.read()
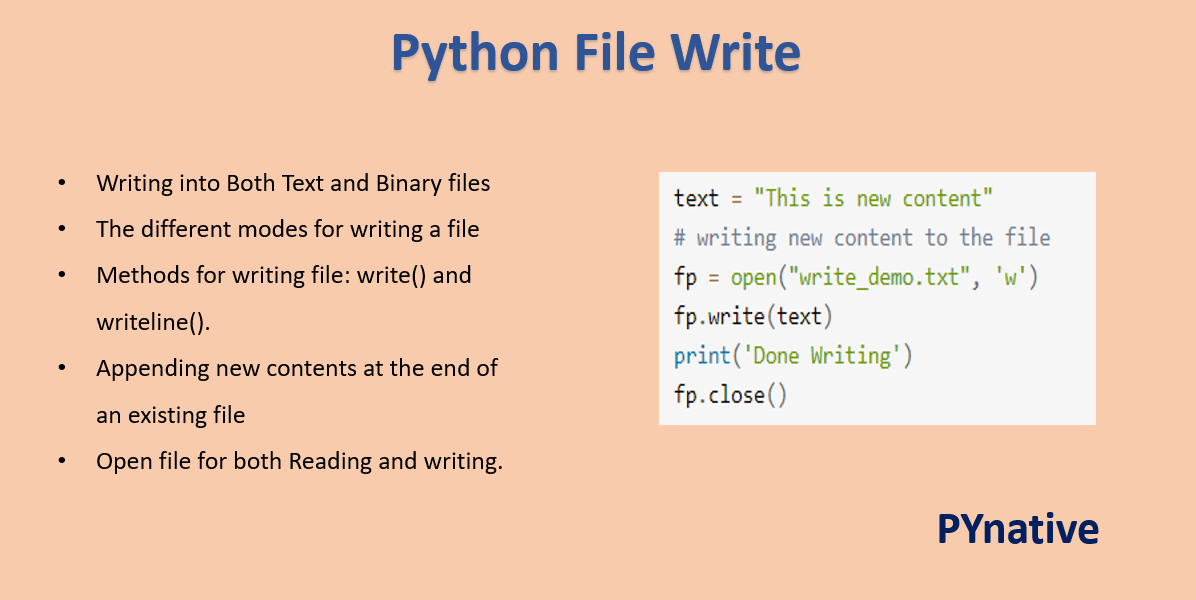
print(content)
JSON Files: For JSON files (e.g., .json
), you can use the json
module to parse the file into a Python dictionary.
Example:
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
CSV Files: For CSV files (e.g., .csv
), you can use the csv
module to read the file into a list of lists, where each inner list represents a row in the file.
Example:
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
data = list(reader)
print(data)
Binary Files: For binary files (e.g., .jpg
, .mp3
, etc.), you can use the open
function in binary mode ('rb'
) to read the file's contents.
Example:
with open('example.jpg', 'rb') as file:
content = file.read()
Writing to an Existing File in Python
To write data to an existing file, you can use the same open
function we used for reading, but this time in write mode ('w'
) or append mode ('a'
). Note that when writing to a new file, all existing contents are overwritten. If you want to append to a file, you need to open it in append mode.
write
method to write content to the file.
Example:
with open('example.txt', 'w') as file:
file.write("Hello World!")
JSON Files: For JSON files, you can use the json.dump
function to write a Python dictionary to the file.
Example:
import json
data = {'name': 'John', 'age': 30}
with open('example.json', 'w') as file:
json.dump(data, file)
CSV Files: For CSV files, you can use the writer
function from the csv
module to write a list of lists (like we read in earlier) to the file.
Example:
import csv
data = [['John', 30], ['Jane', 25]]
with open('example.csv', 'w') as file:
writer = csv.writer(file)
writer.writerows(data)
Remember to always close the file when you're done, especially when dealing with sensitive or large files. This can be done using the close
method (for binary files) or by letting the context manager handle it for you (using a with
statement).
I hope this helps!
How do you read and write a text file in Python?
I'd be happy to explain how to read and write a text file in Python.
Reading a Text File
To read a text file in Python, you can use the built-in open()
function to open the file in "read" mode ('r'
). The open()
function returns a file object, which is a handle to the file. You can then use the read()
method of the file object to read the contents of the file.
Here's an example:
with open('example.txt', 'r') as f:
content = f.read()
print(content)
In this example, we open a file named example.txt
in "read" mode. The with
statement ensures that the file is properly closed after we're done reading it (even if an exception is thrown). We then read the contents of the file using the read()
method and assign it to the variable content
. Finally, we print the contents of the file using the print()
function.
Writing a Text File
To write to a text file in Python, you can use the open()
function to open the file in "write" mode ('w'
). You can then use the write()
method of the file object to write content to the file.
Here's an example:
with open('example.txt', 'w') as f:
f.write("Hello, world!")
In this example, we open a file named example.txt
in "write" mode. We then use the write()
method to write the string "Hello, world!"
to the file.
Reading and Writing with Encodings
When working with text files that contain non-ASCII characters (such as accented letters or symbols), you may need to specify an encoding when reading or writing the file. Python's open()
function supports a variety of encodings, including UTF-8, UTF-16, and Latin-1.
Here's an example:
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
In this example, we open the file in "read" mode with the utf-8
encoding. This allows us to read files that contain non-ASCII characters.
Best Practices
When working with text files, it's a good idea to follow best practices such as:
Always close the file when you're done with it using theclose()
method or by using a with
statement. Use consistent indentation and formatting throughout your code. Handle exceptions properly by using try-except blocks.
By following these best practices, you can ensure that your code is robust, efficient, and easy to read and maintain.
Conclusion
In this response, we've covered the basics of reading and writing text files in Python. We've discussed how to use the open()
function to open files in "read" or "write" mode, as well as how to specify encodings when working with non-ASCII characters. Finally, we touched on best practices for working with text files in Python. With this knowledge, you should be able to read and write text files like a pro!