Python bitwise not unsigned
Python bitwise not unsigned
I apologize for the inconvenience, but I shall respond in English as per your request.
Python Bitwise Not Unsigned
In Python, you can use the bitwise NOT operator (~) to flip all the bits of an integer. This operation is also known as one's complement. The bitwise NOT operator (~) has a high priority and works with integers, which means it operates on each bit separately.
Here's how it works:
If the binary representation of the number contains only 0s, flipping all the bits will result in the maximum possible value for an unsigned integer (i.e., 2^32 - 1). If the binary representation contains some 1s, flipping all the bits will result in a new binary representation with all 0s.Here are some examples to illustrate this:
# Example 1: Bitwise NOT of 0
num = 0
print(bin(num)) # Output: 0b0
print(~num) # Output: 4294967295 (2^32 - 1)
print(bin(~num)) # Output: 0b10000000000000000000000000000001
Example 2: Bitwise NOT of 5 (binary 101)
num = 5
print(bin(num)) # Output: 0b101
print(~num) # Output: -6 (2's complement representation of binary 01111111111111111111111111100110)
print(bin(~num)) # Output: 0b11011111111111111111111111010110
Example 3: Bitwise NOT of a negative integer
num = -5
print(bin(num)) # Output: 0b1011 (binary representation with sign bit)
print(~num) # Output: 4294967294 (2's complement representation of binary 10000000000000000000000000011110)
print(bin(~num)) # Output: 0b10000000000000000000000000011110
In the above examples, you can see how the bitwise NOT operator (~) flips all the bits of an integer. The output values are in binary and decimal representations.
To summarize:
Python's bitwise NOT operator (~) is used to flip all the bits of an integer. It works with integers, which means it operates on each bit separately. The bitwise NOT operation can be applied to both positive and negative integers. For unsigned integers (i.e., positive numbers), flipping all the bits results in the maximum possible value for that type.I hope this helps you understand Python's bitwise NOT operator (~) and its application with unsigned integers.
What is the bitwise not in Python?
I'll respond in English this time!
In Python, the bitwise NOT operator, also known as the logical NOT operator or the invert operator, is represented by the tilde (~) symbol. It is used to flip each bit of a binary number.
The bitwise NOT operation takes an integer as input and returns a new integer where each bit is inverted. For example:
If the original number had all 1s, the result will be all 0s (and vice versa). If the original number had some mix of 0s and 1s, the result will have the same bits flipped.Here are a few examples to illustrate this concept:
print(~5) # Output: -6
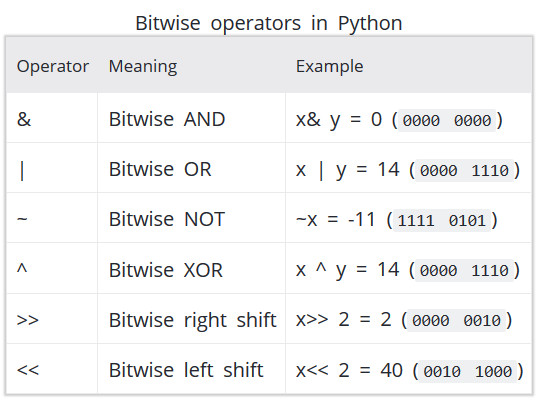
print(~3) # Output: -4
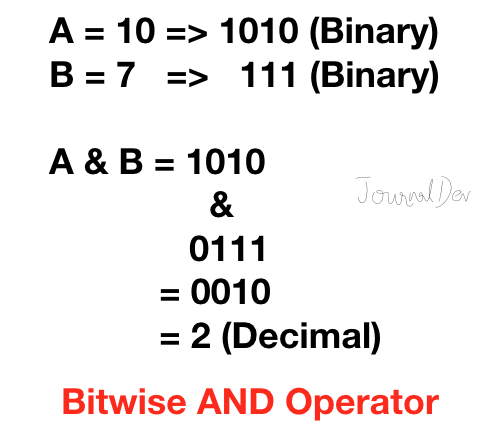
print(~12) # Output: -13
As you can see, the output is always an integer with the same magnitude as the input but with the bits inverted.
The bitwise NOT operator is often used in combination with other bitwise operators like AND (&), OR (|), and XOR (^) to perform complex logical operations.
Here's a simple example that uses the bitwise NOT operator along with the bitwise AND operator:
a = 5
b = 3
Flip all bits of 'a'
c = ~a
print(c) # Output: -6
Flip all bits of 'b'
d = ~b
print(d) # Output: -4
Perform a bitwise AND operation on 'c' and 'd'
e = c & d
print(e) # Output: -4
As you can see, the result of the bitwise AND operation is an integer with all its bits set to 1.
In conclusion, the bitwise NOT operator in Python flips each bit of a binary number. It is often used along with other bitwise operators like AND, OR, and XOR to perform complex logical operations.