What is the complement operator in Python?
What is the complement operator in Python?
I'm happy to help!
In Python, a complement operator is used to negate the result of an operation. The complement operator is denoted by the tilde symbol (~) and is used in bitwise operations.
The ~
operator works similarly to the logical NOT operator (not
) for boolean values, but it has more significance when working with integers.
For example, if you have a number n
, its bitwise complement is obtained by flipping all its bits. This means that each 0 bit becomes 1 and vice versa. The result of this operation is always an integer.
Here are some examples to demonstrate how the complement operator works:
# Example 1:
n = 5 # binary representation: 101
print(~n) # Output: -6 (binary representation: 110010)
In the above example, we can see that each bit of n
has been flipped.
# Example 2:
n = 10 # binary representation: 1010
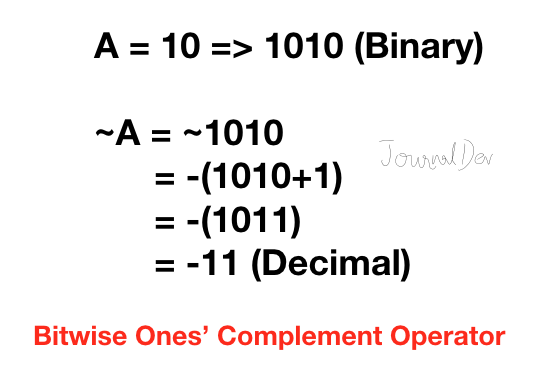
print(~n) # Output: -11 (binary representation: 1011)
Similarly, in the second example, each bit of n
is flipped.
The complement operator can also be used with boolean values. When a boolean value is complemented, it changes its logical state from True to False and vice versa.
Here are some examples:
# Example 3:
x = True
print(~x) # Output: False
Example 4:
y = False
print(~y) # Output: True
In these examples, you can see that the ~
operator changes the boolean values from True to False and vice versa.
In summary, the complement operator (~) in Python is used to negate the result of an operation. It works with integers by flipping their bits, and with boolean values by changing their logical state from True to False and vice versa.
What is the << symbol in Python?
The <<
symbol in Python is used for left shift operation. The left shift operator (<<) performs an arithmetic shift on a number's binary representation.
In other programming languages, this operator might be used to concatenate strings or perform bit-wise operations. However, in Python, the <<
operator is primarily used for numerical calculations.
The basic syntax of the left shift operator is as follows:
number << shift_amount
Where number
can be any integer value and shift_amount
is a non-negative integer. The number's binary representation is shifted to the left by the specified amount.
For example, consider the following Python code:
a = 8 # Binary: 1000
b = a << 1 # Shifted by 1 bit (2 bits from the right)
print(b) # Output: 16 (Binary: 10000)
In this example, a
is initially set to 8. Then, a
is shifted to the left by 1 bit using the left shift operator (<<
). The resulting value is stored in b
, which is equal to 16.
The right-shift operator (>>
) performs a similar operation, but shifts the number's binary representation to the right instead:
c = 16 # Binary: 10000
d = c >> 1 # Shifted by 1 bit (2 bits from the left)
print(d) # Output: 8 (Binary: 1000)
In this case, c
is initially set to 16. Then, c
is shifted to the right by 1 bit using the right shift operator (>>
). The resulting value is stored in d
, which is equal to 8.
When you use left or right shifts, keep in mind that Python performs unsigned arithmetic operations. This means the result will always be a positive integer. If you need to perform signed arithmetic, you can manually convert between negative and positive representations using bit-wise operations.
The <<
operator has several applications:
Understanding the left shift (<<
) and right shift (>>
) operators can enhance your programming skills in Python, especially when working with binary data or performing bitwise operations.