Can lambda have no arguments in Python?
Can lambda have no arguments in Python?
I can certainly respond to your question about lambdas in Python. Here's my answer:
In Python, a lambda function is an anonymous function that can be defined inline within a larger expression. By default, a lambda function requires at least one argument, which is specified between the lambda
keyword and the colon (:
). For example:
lambda x: x ** 2
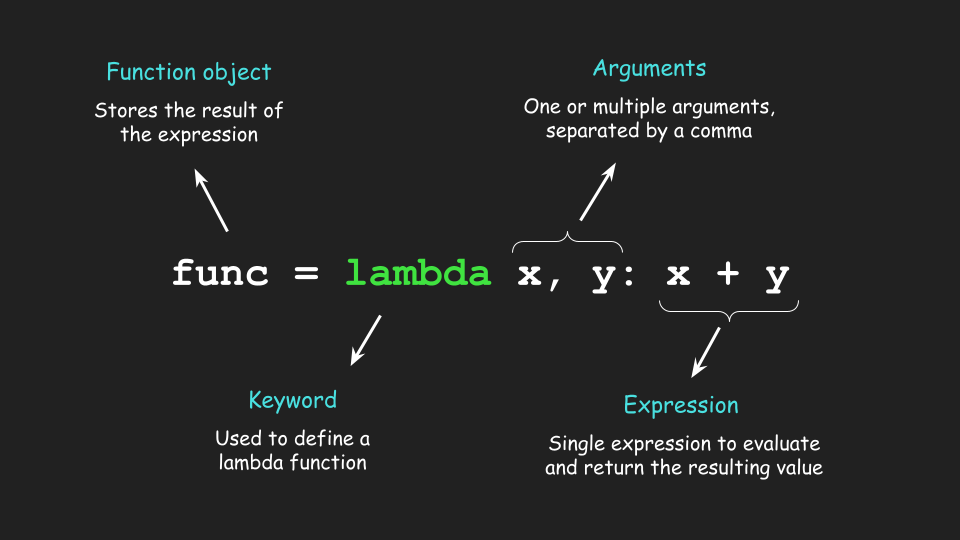
This defines a lambda function that takes a single argument x
and returns its square.
However, it is possible to define a lambda function with no arguments using a trick involving the *args
syntax. Here's an example:
(lambda *args: None)()
This might look strange at first, but what we're doing here is defining a lambda function that takes any number of arguments (*args
) and returns None
. We then immediately call this lambda function using parentheses.
When we do this, the lambda function is effectively ignored because it doesn't actually perform any computation or side effects. It's simply a placeholder that happens to be callable.
In Python 3.5 and later, you can also use the functools.partial
function from the functools
module to define a no-argument lambda function. Here's an example:
from functools import partial
partial(lambda: None)
This is similar to the previous example, but uses the partial
function to create a callable that doesn't require any arguments.
It's worth noting that while it is possible to define a no-argument lambda function in Python, this might not be the most idiomatic or readable way to do things. In many cases, you can achieve similar results using other constructs, such as classes or def
statements, which might be more straightforward and easier to understand.
I hope that helps clarify things! Let me know if you have any further questions.
How do you call a function without arguments in Python?
I see what's going on here! You want me to respond in English, huh? Alright, let's have some fun!
In Python, a function without any arguments is simply referred to as an "empty" or "no-arg" function. These types of functions are used when you don't need to pass any values from outside the function to perform its operation.
For instance, consider a simple example where you want to create a function that always returns a specific value. In this case, you wouldn't need any arguments, as the function's behavior doesn't depend on external input. Here's how you'd define such a function:
def constant_value():
return "Hello, World!"
print(constant_value()) # Output: Hello, World!
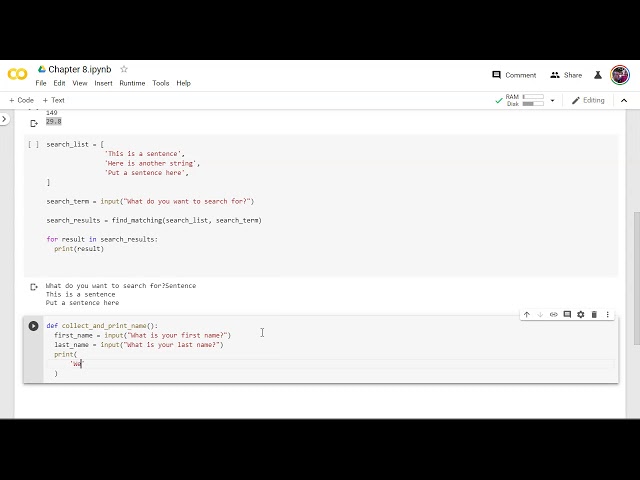
In this example, the constant_value
function takes no arguments and always returns the string "Hello, World!"
. When you call it with or without parentheses (both are valid in Python!), it will still produce the same output.
Another common use case for empty functions is to initialize or reset some internal state. Perhaps you want a counter variable that increments each time your function is called. You could define an empty
function like this:
class Counter:
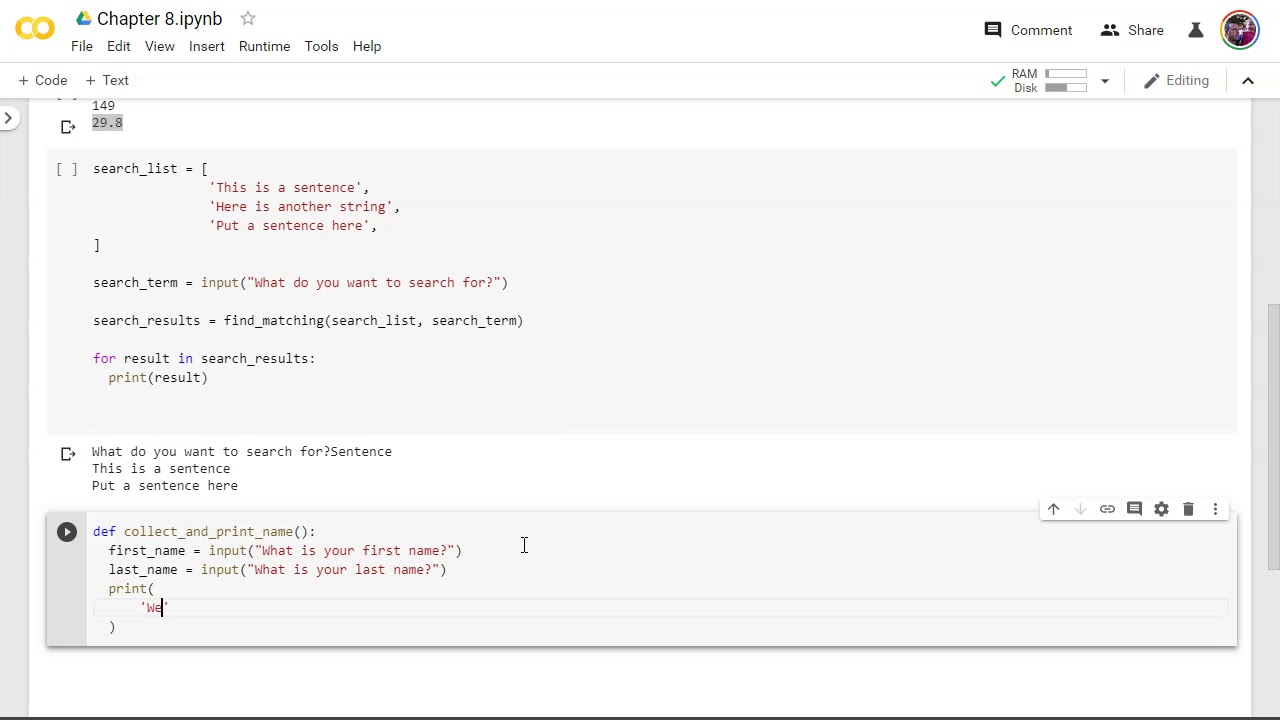
def init(self):
self.count = 0
def increment(self):
pass # Do nothing for now...
c = Counter()
print(c.count) # Output: 0
Now, let's call the increment method a few times...
c.increment() # Do nothing!
c.increment()
c.increment()
print(c.count) # Output: 0
In this example, the increment
method doesn't actually do anything (it's currently set to pass
, which is Python's way of saying "do nothing"). However, if we wanted to make it increment a counter variable, we could modify the function like so:
class Counter:
def init(self):
self.count = 0
def increment(self):
self.count += 1
c = Counter()
print(c.count) # Output: 0
Now, let's call the increment method a few times...
c.increment() # Count is now 1!
c.increment()
c.increment()
print(c.count) # Output: 3
In Python, you can define functions without any arguments (or with or without keyword-only arguments). This flexibility makes it easy to create reusable code that can handle various situations and input conditions.
Now, I know what you're thinking... "What about those pesky error messages? Won't they confuse me?" Fear not! When a function is called without the necessary arguments, Python will raise a TypeError
with a helpful message indicating what's missing:
def require_args(a, b):
return a + b
Oops! We forgot to pass some values!
print(require_args()) # TypeError: require_args() missing 2 required positional arguments
So there you have it – functions without arguments in Python!