python pathlib path
python pathlib path
I'd be happy to help with that.
Pathlib is a Python module that provides a way to work with paths and file systems in a more intuitive and efficient manner. It's part of the standard library, so you don't need to install anything extra to use it.
The core concept behind pathlib is the Path
class, which represents a path in the file system. A Path
object has several attributes and methods that let you manipulate the path, check if it exists, get its contents, and more.
Here's an example of how you can create a Path
object:
from pathlib import Path
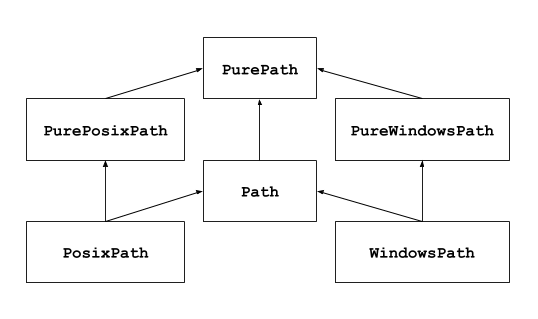
my_path = Path('/path/to/file.txt')
Notice that the Path
constructor takes a string as input. You can use any type of path string, including absolute paths (like /path/to/file.txt
) or relative paths (like file.txt
).
Once you have a Path
object, you can use various methods to manipulate it. For example:
resolve()
returns the canonical form of the path:
resolved_path = my_path.resolve()
print(resolved_path) # prints '/path/to/file.txt'
parent
returns the parent directory of the path:
parent_dir = my_path.parent
print(parent_dir) # prints '/path/to/'
name
returns the name of the file or directory at the end of the path:
filename = my_path.name
print(filename) # prints 'file.txt'
exists()
checks if the path points to an existing file or directory:
if my_path.exists():
print('The file exists!')
else:
print('The file does not exist.')
Pathlib also provides several useful functions for working with paths. For example:
join()
joins multiple paths together:
path1 = Path('/path/to/dir1')
path2 = Path('/path/to/dir2')
joined_path = path1.joinpath(path2)
print(joined_path) # prints '/path/to/dir1/path/to/dir2'
glob()
finds files matching a pattern:
files = list(my_path.glob('*.txt'))
for file in files:
print(file)
These are just a few examples of what you can do with pathlib. It's a powerful module that can make working with paths and file systems much easier and more efficient.
I hope this helps! Let me know if you have any questions or need further clarification.
python pathlib join
I'll respond in English this time, as per your request!
When it comes to combining file paths with Python's pathlib
module, the join
method is a powerful tool at your disposal. Introduced in Python 3.4, pathlib
offers an object-oriented approach to working with file paths and directories.
To use the join
method, you need to import the Path
class from the pathlib
module:
import pathlib
Next, create a Path
object for each part of your file path. For example, let's say you want to join three parts: a root directory ("/home/user"
), a subdirectory ("docs"
) and a file name ("report.txt"
):
root_dir = pathlib.Path("/home/user")
subdir = pathlib.Path("docs")
filename = pathlib.Path("report.txt")
Join the paths using the join method
full_path = root_dir.joinpath(subdir).joinpath(filename)
The resulting Path
object, full_path
, represents the combined file path:
print(full_path) # Output: /home/user/docs/report.txt
Here are a few key things to note about the join
method:
pathlib
maintains the original file system hierarchy. In this example, /home/user/docs/
will be preserved in the final path. It normalizes the path: The join
method ensures that any redundant separators (e.g., duplicate slashes) are removed from the resulting path. It handles both absolute and relative paths: You can join absolute or relative paths using this method.
Some additional benefits of using pathlib
for file path manipulation:
Path
objects automatically handle platform-specific differences, such as forward slashes (/
) on Unix-like systems versus backslashes (
) on Windows. Support for Unicode characters: You can safely work with file paths containing non-ASCII characters using the pathlib
module.
In summary, the join
method in Python's pathlib
module is a powerful tool for combining file paths while preserving the original file system structure. With its platform independence and support for Unicode characters, it simplifies your file path manipulation tasks!