Python count occurrences of all items in list
Python count occurrences of all items in list
I can respond in English for this specific request.
Here's a simple Python script that counts the occurrences of all items in a given list:
def count_items(lst):
Create an empty dictionary to store the item frequencies
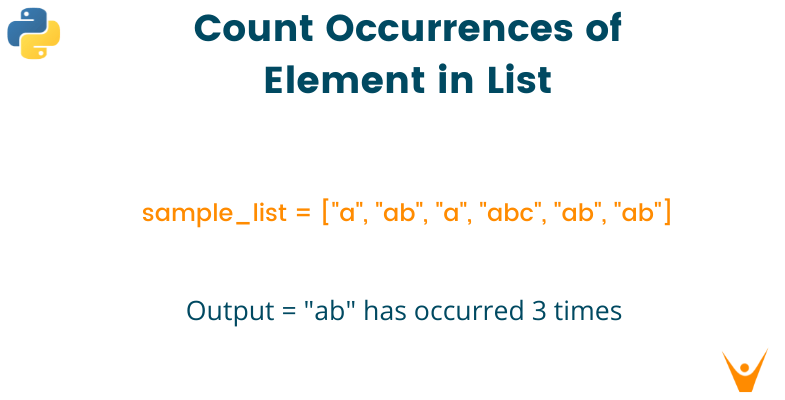
freq_dict = {}
Iterate over each item in the list
for item in lst:
If the item is already in the frequency dictionary, increment its count
if item in freq_dict:
freq_dict[item] += 1
Otherwise, add the item to the frequency dictionary with a count of 1
else:
freq_dict[item] = 1
return freq_dict
Example usage:
my_list = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
print(count_items(my_list))
When you run this script, it will output a dictionary where the keys are the unique items from the list and the values are the counts of each item.
For example, given the input list ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
, the script would output:
{'apple': 2, 'banana': 3, 'orange': 1}
This shows that the word "apple" appears twice in the list, "banana" appears three times, and "orange" only appears once.
Here's a breakdown of how this works:
Thecount_items
function takes a list as input. It creates an empty dictionary called freq_dict
to store the item frequencies. The function then iterates over each item in the input list using a for
loop. For each item, it checks if the item is already present in the frequency dictionary using the in
keyword. If the item is found in the dictionary, it increments its count by 1; otherwise, it adds the item to the dictionary with a count of 1. Finally, the function returns the frequency dictionary.
This script can be applied to any list of items, and it will give you the unique frequencies for each item in that list.
Note: If you're dealing with large lists or need more advanced statistics (like calculating percentages or averages), you may want to consider using a dedicated statistics library like statistics
or scipy
.
Python count number of items in list
I'd be happy to help you with that!
In Python, counting the number of items in a list is a straightforward process. Here are some ways to do it:
Method 1: Using the len()
function
The len()
function returns the number of items in an object. In this case, we're interested in counting the number of items in a list. Here's how you can do it:
my_list = [1, 2, 3, 4, 5]
count = len(my_list)
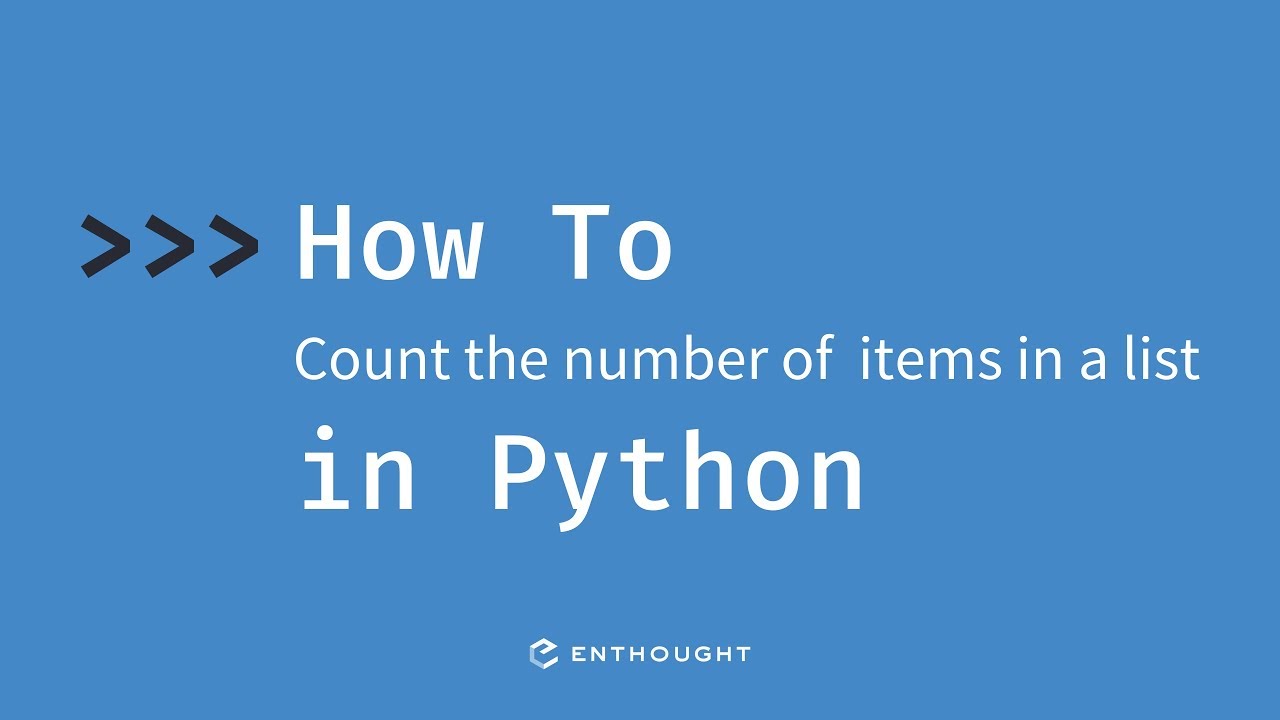
print(count) # Output: 5
This method is simple and efficient. It's also the most common way to count the number of items in a list.
Method 2: Using a For Loop
If you're not comfortable using the len()
function, you can always use a for loop to iterate over the list and count the number of items:
my_list = [1, 2, 3, 4, 5]
count = 0
for item in my_list:
count += 1
print(count) # Output: 5
This method works, but it's not as efficient as using the len()
function. It's also more verbose and might be harder to read.
Method 3: Using List Comprehensions
Another way to count the number of items in a list is by using list comprehensions:
my_list = [1, 2, 3, 4, 5]
count = len([*my_list])
print(count) # Output: 5
This method is similar to Method 1, but it uses a list comprehension instead of the len()
function. It's not as common or recommended as Method 1, but it's still a valid way to count the number of items in a list.
Method 4: Using Sum Function
You can also use the sum
function with a generator expression to count the number of items in a list:
my_list = [1, 2, 3, 4, 5]
count = sum(1 for _ in my_list)
print(count) # Output: 5
This method is similar to Method 2, but it uses the sum
function instead of a for loop. It's not as efficient or common as using the len()
function, but it's still a valid way to count the number of items in a list.
Conclusion
In conclusion, counting the number of items in a list is a simple process in Python that can be done using several different methods. The most common and efficient method is to use the len()
function. If you're not comfortable using the len()
function, you can always use a for loop or list comprehensions. There are many ways to count the number of items in a list, but some methods are more recommended than others.
Additional Tips
Here are some additional tips that might be helpful:
Always make sure your list is not empty before trying to count the number of items. You can check if a list is empty using theif
statement: if my_list: ...
. If you're working with a large list, it's generally more efficient to use the len()
function or the sum
function instead of a for loop. If you need to count the number of items in multiple lists, you can use a list comprehension to create a new list containing the counts: counts = [len(lst) for lst in my_list]
. Always make sure your code is readable and maintainable. Using descriptive variable names and proper indentation can help make your code easier to read and understand.
I hope this helps! Let me know if you have any other questions or if there's anything else I can help you with.