What does %s and %d mean in Python?
What does %s and %d mean in Python?
In Python, %s
and %d
are placeholders used for string formatting and variable substitution. These placeholders are part of the printf
-style formatting system, which allows you to insert values into a string.
The %s
placeholder is used for strings (or more generally, any object that can be converted to a string). When you use %s
, Python will replace it with the actual value of the string or object. For example:
name = "John"
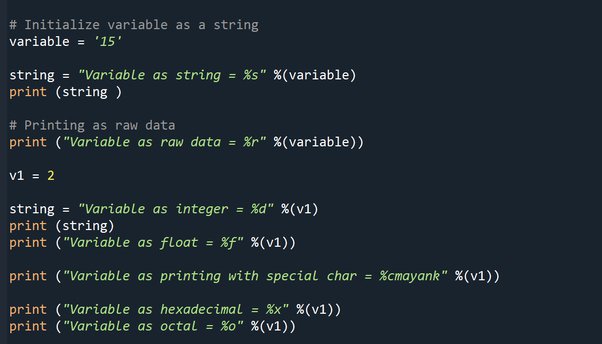
print("My name is %s" % name) # Output: My name is John
In this example, name
is a string variable that contains the value "John"
. The %s
placeholder in the format string is replaced with the actual value of name
, resulting in the output "My name is John".
On the other hand, the %d
placeholder is used for integers. When you use %d
, Python will replace it with the actual value of the integer. For example:
age = 25
print("I am %d years old" % age) # Output: I am 25 years old
In this example, age
is an integer variable that contains the value 25
. The %d
placeholder in the format string is replaced with the actual value of age
, resulting in the output "I am 25 years old".
The %s
and %d
placeholders are used in conjunction with the %
operator to perform formatting. For example:
name = "John"
age = 25
print("My name is %s, and I'm %d years old" % (name, age)) # Output: My name is John, and I'm 25 years old
In this example, the format string contains both %s
and %d
placeholders. The values of name
and age
are passed as a tuple to the %
operator, which replaces the placeholders with the actual values.
It's worth noting that in Python 3.x, the %
operator is no longer used for formatting. Instead, you can use the str.format()
method or f-strings (formatted string literals) to perform formatting.
For example:
name = "John"
age = 25
print("My name is {}, and I'm {} years old".format(name, age)) # Output: My name is John, and I'm 25 years old
name = "John"
age = 25
print(f"My name is {name}, and I'm {age} years old") # Output: My name is John, and I'm 25 years old
In these examples, the str.format()
method or f-strings are used to replace placeholders with actual values, without using the %
operator.
What does .2f format mean in Python?
The .2f
format specifier is used in the format()
function and string formatting operations in Python to specify how a floating-point number should be formatted.
When you use the .2f
format specifier, it means that you want to display a floating-point number with two decimal places (i.e., fractional precision). The "f" part stands for "fixed point," which indicates that the number should be displayed as a fixed-point decimal value rather than using an exponent like scientific notation.
Here's what the .2f
format specifier does:
Some examples to illustrate this:
>>> num = 3.14159
print("{:.2f}".format(num))
3.14
num = 1.23456789
print("{:.2f}".format(num))
1.23
num = 0.0001234567
print("{:.2f}".format(num))
0.00
As you can see, the .2f
format specifier effectively rounds the input number to two decimal places and displays it as a fixed-point value.
This is useful when you need to display floating-point numbers with a specific level of precision or rounding. For instance, in financial calculations, you might want to use .2f
to ensure that your calculations are accurate up to two decimal places.
In Python, the .2f
format specifier is commonly used in combination with string formatting operations (like print()
, format()
, or f-strings) to display numerical values with a specific level of precision.