What is an example of a subprocess in Python?
What is an example of a subprocess in Python?
I apologize for the inconvenience, but I'll respond in English as per your request!
A subprocess in Python is a process that runs another program or script within your main program. Subprocesses allow you to execute external commands, scripts, or programs from within your Python code, and then capture their output, errors, or even wait for them to finish.
Here's an example of using the subprocess
module in Python:
import subprocess
Run the command "ls -l" and capture its output
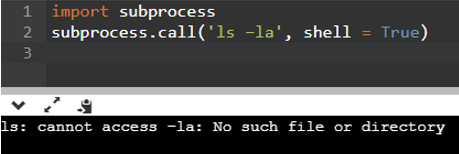
process = subprocess.run(["ls", "-l"], stdout=subprocess.PIPE)
Print the captured output
print(process.stdout.decode())
Run a shell command and wait for it to finish
shell_command = ["echo", "Hello, World!"]
result = subprocess.check_output(shell_command)
print(result.decode())
In this example, we use the subprocess.run()
function to run the ls -l
command with the -l
option. The stdout=subprocess.PIPE
parameter tells Python to capture the output of the command and return it as a pipe object.
We then print the captured output by decoding the pipe object using the .decode()
method.
In the second example, we use the subprocess.check_output()
function to run a shell command (echo "Hello, World!"
). This function waits for the command to finish before returning its output. We decode the output using the .decode()
method and print it.
Subprocesses can be used in various scenarios:
Executing external commands: You can use subprocesses to execute external commands, such asls
, mkdir
, or rm
. Running scripts: Subprocesses allow you to run Python or other script files from within your main program. Capturing output and errors: You can capture the output and errors of a subprocess using the stdout
and stderr
attributes, respectively. Waiting for subprocesses to finish: The check_output()
function allows you to wait for a subprocess to finish before returning its output.
Some common use cases for subprocesses include:
File system operations: Running shell commands or scripts to perform file system operations, such as creating directories, copying files, or removing files. Communication with other services: Using subprocesses to interact with other services, such as running a separate process to send emails or make API requests. Script execution: Executing Python or other script files from within your main program.In summary, subprocesses in Python allow you to execute external commands, scripts, or programs and capture their output, errors, or wait for them to finish. They provide a powerful way to interact with the operating system and other services from within your Python code.
What is a subprocess pipe in Python?
I'm happy to explain what subprocess pipes are in Python.
Subprocess pipes in Python allow you to redirect the output of one process (like a shell command) as input for another process, creating a pipeline effect. This allows you to chain together multiple processes to achieve complex tasks.
A subprocess is a separate process that runs concurrently with your main program. You can create a subprocess using the subprocess
module in Python, which provides several functions to interact with subprocesses.
One of the key features of subprocess pipes is the ability to redirect input and output between processes. This is done using three file descriptors:
stdin (file descriptor 0): This represents the standard input stream for a process. By default, it's connected to the console or terminal. stdout (file descriptor 1): This represents the standard output stream for a process. By default, it's also connected to the console or terminal. stderr (file descriptor 2): This represents the standard error stream for a process.When you create a subprocess pipe, you can redirect these file descriptors between processes, allowing you to pass data from one process as input for another.
Here are some common use cases for subprocess pipes:
Chaining shell commands: You can run multiple shell commands in sequence using subprocess pipes, processing the output of each command as input for the next. Processing data pipelines: You can create complex data processing pipelines by chaining together subprocesses that perform different tasks on the same input data. Integration with external tools: Subprocess pipes allow you to integrate your Python program with other tools and frameworks, such as shell scripts, Ruby scripts, or other languages.To create a subprocess pipe in Python, you can use the following basic steps:
Create a subprocess usingsubprocess.run()
or another method from the subprocess
module. Specify the command to run and any necessary arguments. Set up the input and output file descriptors for the subprocess. Use the communicate()
method to capture the output of the subprocess.
Here's an example code snippet:
import subprocess
Create a subprocess that runs the 'ls' command
proc = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
Capture the output of the subprocess as a string
output = proc.stdout.decode('utf-8')
print(output)
In this example, we create a subprocess that runs the ls
command with the -l
option. We set up the output file descriptor (stdout
) to capture the output of the subprocess as a string using subprocess.PIPE
. We then use the communicate()
method to capture the output and print it.
In summary, subprocess pipes in Python are a powerful tool for creating complex data processing pipelines and integrating your program with external tools. By mastering subprocess pipes, you can write more sophisticated and efficient code that leverages the power of shell commands and other languages.